Python is one of the best languages, if not the best, for beginners. It can be a great entry-level language because of its readability and simple syntax. In this tutorial, I will try to walk you through the basics of the Python programming language.
Why Python?
Python is the easiest, most popular, and powerful programming language out there. We can use Python as a great tool to solve real-world problems.
I suggest Python for beginners because it is easy to learn. It is a high-level programming language with applications in numerous areas, including web development, machine learning, and many more.
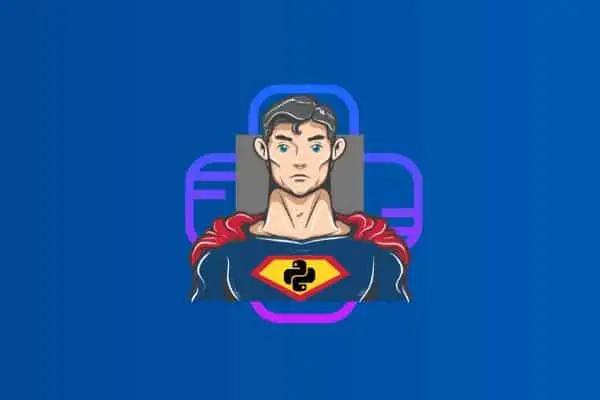
Since it is so beginner-friendly, many universities are now using Python to teach programming concepts to students. Also, the most promising technology, artificial intelligence, is powered mainly by Python. Python contains many libraries that help us to do things pretty easily.
I’m not saying Python is better than other languages. Every language has its own specialties. But, if you’re a beginner, and I’ve convinced you enough to start with Python, then let’s start learning it.
Getting Started
Before we start, if you don’t know anything at all about computer programming and you feel intimidated about it, I suggest you read a small article that I wrote on Introduction To Computer Programming.
This article will help you understand the fundamentals of problem-solving using code. Once you’ve understood everything that I’ve written there, come back to this post and learn the basics of Python.
Download Python
Before starting to learn the basics of the language, you need to install Python software in your system. So, go to this link and download Python. We will be using Python 3 (not the old Python 2 version).
If you don’t want to install any software, just go to repl.it and get started quickly. Select Python 3 as your language, and click “create repl”. It is a free and easy-to-use platform for coding.
Printing In Python
We can print something in Python using the print statement, followed by a parenthesis, which contains the things we want to print.
If we want to print a text or string, we will write the text inside parenthesis surrounded by either single quotes or double quotes.
Let’s see an example.
print("hello world")
This code will print the string “hello world” as the output.
If we want to print a numeric value or a variable, we will write it inside parentheses without using quotes. I will tell you what a variable is in a moment. Now, let’s see an example of printing a numeric value.
print(1500)
This code will print the number 1500.

Note: A Python program is saved using the extension ” .py “.
Variables
We use variables in Python to store values. We can assign the variable a name.
We can use letters, numbers, and underscore while naming a variable. A variable name should not begin with numbers. It can begin with either a letter or an underscore.
In Python, we don’t need to explicitly initialize a variable as we do in other programming languages such as C, C++, Java, etc. They can be dynamically used without specifying their data type.
Let’s see an example code in which we assign the variable some value and use it.
applesCount = 50
print(applesCount)
This program will print 50 as the output.

Getting User Input
Getting input from the user is an important task in programming. We can use the user input to do the required operations and produce the output.
In Python, user input is obtained by using the input() method. You can store the user input in a variable and use it for required operations.
Let’s see an example.
number = input()
We can also give instructions to the user for typing in the required input. These instructions can be written inside parentheses as an argument of the input() method.
Let’s see an example of that.
backgroundColor = input(" Enter a color: ")
This program will ask the user to enter the name of a color. The user can enter the name of a color, and it will be stored in the variable as a string.

Writing Comments
We use comments in Python for a better understanding of code. These are ignored by the compiler, and the machine does not care about them. So, we can write whatever we want as comments in the code.
Comments are written only for humans to read so that they get a swift and solid understanding of the code. If you use it correctly, it’ll be helpful to read and understand your code for other programmers as well.
In Python, single-line comments are created by using the # symbol. We can write our comments just after the # symbol.
Let’s see an example of a comment.
#This is a single line comment
Multiple line comments are created by placing the comment between ”’ and ”’.
Let’s see an example of multiple-line comments in Python.
''' This is
a multiple-line
comment '''
You can use comments to enhance the readability of your code.
Operators in Python
Operators are predefined symbols that can carry out particular operations. The participants of the operation are called operands.
There are many different types of operators available in Python. We can generally categorize them into the following types:
- Arithmetic Operators
- Increment and Decrement Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
Now let’s discuss each category in detail.
Arithmetic Operators
Arithmetic operators are those symbols that can do arithmetic calculations. In general, there are five of them.
+ | Addition operator | We use the addition operator to carry out the addition of two operands. |
– | Subtraction operator | The subtractor operator helps to carry out the subtraction of two numbers. |
* | Multiplication operator | We use it to multiply two operands. |
/ | Division operator | It helps to divide two operands. |
% | Modulus operator | This operator gives the reminder value after the division of two operands. |
There are also two different types of arithmetic operators, unary and binary. Unary operators operate on a single operand. Operators applied to two operands are called binary operators.
Note that we can use ** to find the exponent of one number over another. Also, we can use // to find the quotient after dividing two numbers.
Increment and Decrement Operators
The increment operator (++) helps to increment a number, that is, adding 1 to the number. Also, the decrement operator (—) helps to decrement a number, that is, subtracting 1 from a number.
Relational Operators
We can use relational operators for comparing two numbers to find the relation between them. The relational operators that are available in Python are:
== | This operator checks whether the operand values are equal |
!= | This operator is used to check whether operand values are not equal |
> | This operator checks whether the first operand is greater than the second one |
< | This operand checks whether the first operand is lesser than the second one |
>= | This operator checks whether the first operand is greater than or equal to the second one |
<= | This operator checks whether the first operand is lesser than or equal to the second one |
Logical Operators
We can use logical operators to obtain true or false values. There are mainly 3 logical operators:
and | This operator returns true if both the operands on it’s either side are true. |
or | This operator returns true if any of the operands on it’s either side are true. |
not | This operator is used to reverse the logical state of an operand. |
Bitwise Operators
Bitwise operators in Python are used to do operations on binary data. I’m not talking about binary operations here since you’re a beginner. As of now, just understand that the following bitwise operators are available in Python:
- & (Binary AND)
- | (Binary OR)
- ^ (Binary XOR)
- ~ (Binary One’s Complement)
- << (Binary Left Shift)
- >> (Binary Right Shift)
If you want to learn how to do bitwise operations on binary data, you can check out this tutorial.
Assignment Operator
= is used as the assignment operator in Python. This operator assigns a value to a variable.
We can also use a compound assignment operator like += to do addition and assignment within a single line of code.
For example, a += 5 is equivalent to a = a + 5.
Expressions in Python
An expression in Python consists of operators and operands. The operands may be either constants or variables.
According to the type of result, we can classify expressions into arithmetic expressions and logical expressions.
Arithmetic Expressions
If an expression contains only arithmetic operators, it is called an arithmetic expression. The result of an arithmetic expression will always be a numeric value. For example, a + b.
They are of two types:
- Integer expressions
- Real expressions (floating-point expressions)
Logical Expressions
If the result of the expression is either logic 1 (true) or logic 0 (false), then the expression is called logical expression. A logical expression may contain constants, variables, logical operators, and relational operators.
For example, a <= b
Conditional Statements in Python
The three conditional statements used in Python are if, elif, and else. We can use these statements wisely to perform many tasks that involve some conditional checking.
These are very important to build logic in our programs. So, I recommend you give extra attention to conditional statements, loops, and functions while learning Python basics.
if statement
We use if statements to determine what the program should do next after the statement is evaluated.
else statement
The else statement runs if the if statement is false.
elif statement
The elif statement allows you to include multiple if statements into one code block to handle various cases.
Let’s have a look at the syntax of these conditional statements.
if condition :
statements
elif condition:
statements
else:
statements
Let’s see an example of using conditional statements in Python. The following Python code will read an integer value as input and print whether the number is odd or even.
myNumber = int(input("Enter a number: "))
if(myNumber%2==0):
print("The number is even.")
else:
print("The number is odd.")
Output:
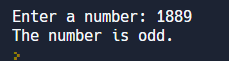
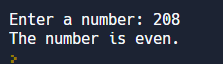
Indentation in Python
Python uses indentation (white space at the beginning of a line) to delimit blocks of code. Other languages such as C, Java, C++, etc., use curly braces { } to accomplish this, but in Python, indentation is mandatory.
Loops in Python
It is necessary to understand the working of loops in order to develop your programming skills.
Loops allow us to execute a statement or group of statements multiple times. Thus we can reduce the burden of repeatedly writing a piece of code many times.
while loop
The while loop checks a condition and executes the task if the condition is satisfied. The syntax of the while loop looks like this:
while condition:
statements
Let’s look at an example.
count=1
while count<=5:
print(count)
count = count+1
The output of this code will be 1 2 3 4 5.
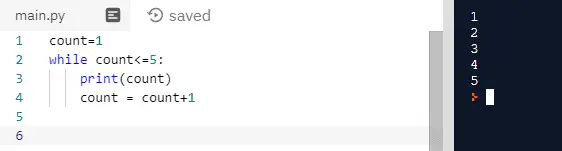
We use the break statement to end a loop prematurely.
We can use the continue statement to stop the current iteration and continue with the next one.
for loop
Iterating through a list using a while loop requires quite a lot of code. That’s why Python provides the for loop.
Let’s see an example:
words = ['hello','world','hi']
for word in words:
print(word)
This code prints all three words separately as output.
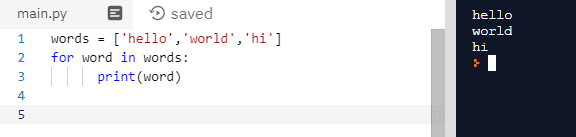
The for loop is commonly used to repeat some code a certain number of times. We can do this by combining “for loops” with range objects.
For example :
for i in range(3):
print('hello')
This code prints hello three times as output.
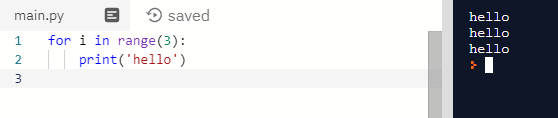
Range
The range function creates a sequential list of numbers. Let’s see an example.
numbers = list(range(10))
print(numbers)
This will produce an output like: [0,1,2,3,4,5,6,7,8,9].
We can use two arguments to set the starting and ending points.
For example:
numbers = list(range(3,10))
print(numbers)
This will give the output like: [3,4,5,6,7,8,9]
The range method can have a third argument, which determines the interval of the sequence produced. The argument must be an integer.
For example:
numbers= list(range(5,10,2))
print(numbers)
The output of this code will be [5,7,9].
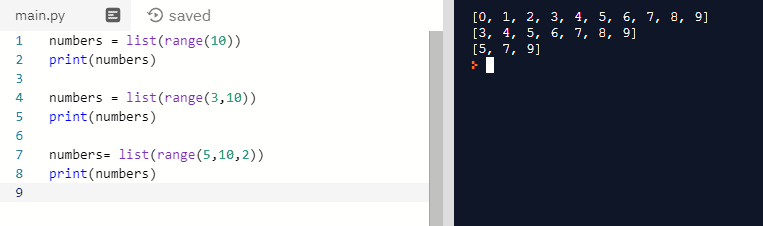
Functions in Python
Any statement that consists of a word followed by information in parenthesis is a function call.
For example:
print('hello')
In addition to the predefined functions, we can create our own functions by using the def statement.
Let’s see an example.
def greetings():
print('Hey')
print('Hellooooo')
greetings()
The first three lines are the function definition, and the last line is the function call. You can call the function whenever you want, once it is defined.
When we call this method, the output will be:
‘Hey’
‘Hellooooo?’
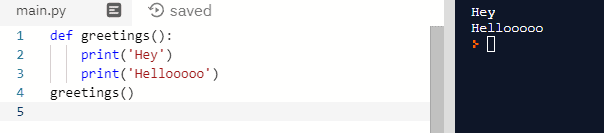
The statements in the function are executed only when they are called. We must define our functions before we call them.
Functions can take arguments (one or more).
Let’s see an example function to add two numbers and print their sum.
def add(x,y):
print(x+y)
add(5,8)
This function call will produce an output: 13.

Certain functions return a value that can be used later. To do this, we can use the return statement.
Let’s see an example function to find the largest of two numbers.
def max(x,y):
if x>=y:
return x
else:
return y
z=max(8,5)
print(z)
This code will produce an output 8.
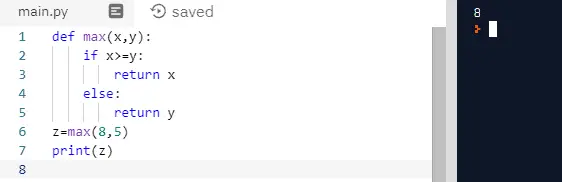
When a function finds a return statement, it immediately stops its execution. The function will not consider the code we write after the return statement.
There are different types of function arguments in Python. If you want to learn more about those, check out this article.
Strings in Python
A string is created by entering a text between two single or double quotes.
For example, “Pythonista Planet” and ‘Learn Python Basics’ are strings.
String Concatenation
We can join together two strings using the + operator.
For example, ‘Pythonista’ + ‘Planet’ gives ‘Pythonista Planet’.
We can also multiply strings in Python.
For example, ‘mango’ * 3 gives ‘mangomangomango’.
To get the letters of a string separately, we can use the index of the letter inside square brackets.
For example, ‘python'[0] gives ‘p’.

String Slicing
To slice a string, use the starting and ending index inside square brackets (like [ start : stop ] ).
For example, ‘python'[0:2] gives ‘py’ .
And ‘python'[0:4] gives ‘pyth’.
If we want to slice the string from the other end, we can do that by using negative numbers.
For example, ‘python'[-1] gives ‘n’ .
Also, ‘python'[0:-1] gives ‘pytho’.
We can also slice the string by doing stepping. [ start : stop : step ]
For example, ‘python'[0:-1:2] gives ‘pto’.
Also, ‘howareyou'[0:-1:3] gives ‘hay’.
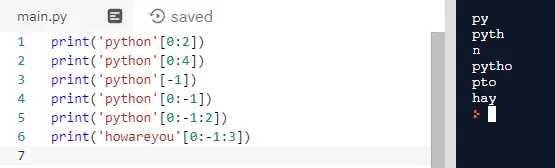
Splitting a String
To split a sentence into a list of words, we can use the split() method.
sentence = "Pythonista Planet"
words = sentence.split()
print(words)
This will produce the output: [‘Pythonista’,’Planet’].

Lists in Python
If we want to store a set of items in an arranged order, we can use lists in Python. We can create a list by using square brackets with items inside. We can use commas to separate each item.
If we want to access a particular item in a list, we can do that by using the index of that item in square brackets.
For example:
words= ['Hello','world','how','are','you']
print(words[0])
This will produce an output ‘Hello‘.
Often, a list contains items of the same type. But, we can also create a list that includes different types of items as well. Lists can also be nested within other lists.
Let’s see an example.
things= ['mango',0,[1,2],4.56]
print(things[2][1])
This will produce the output: 2.
Strings can also be indexed like lists.
For example:
message="hello world"
print(message[6])
This code will produce an output ‘w’.
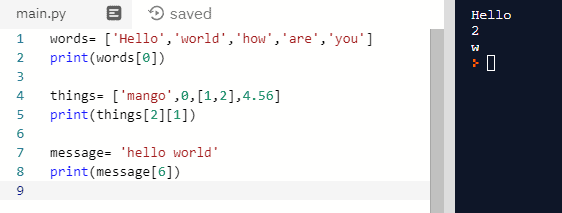
List functions
Now, let’s look at some of the useful methods available in Python for working with lists.
append
The append method adds an item to the end of an existing list.
For example:
numbers= [1,2,3]
numbers.append(4)
print(numbers)
This code will produce the output: [1,2,3,4].
insert
The insert method allows inserting a new item to the end of an existing list.
For example:
words = ['python','fun']
words.insert(1,'is') # (position,item)
print(words)
The output of this code will be: [‘python’,’is’,’fun’].
len
If we want to get the number of items in a list, we can use the len method.
Let’s see an example.
numbers= [1,2,3,4,5]
print(len(numbers))
The output will be 5.
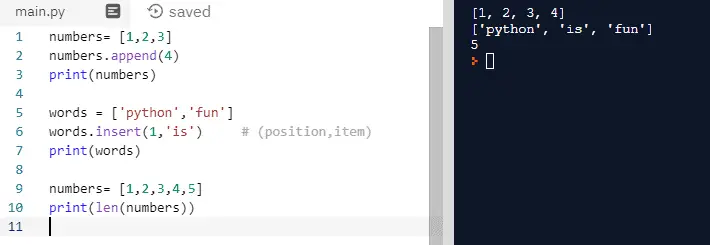
There are a few more useful functions and methods for lists like :
max(list) | To find the maximum value from a list |
min(list) | To find the minimum value from a list |
list.remove(item) | To remove an item from a list |
list.reverse() | To reverse a list |
Modules in Python
Some great developers have written a lot of code to simplify common tasks. Those pieces of code are called modules. These are also called libraries.
Just think of it like Tony Stark designed a powerful Iron Man suit for you, and you can just wear it to have some superpower.
Modules are kind of like this. Some genius people have already spent their sleepless nights writing code in order to make your life easier.
You can just import the modules and call some methods to do the tasks. Problem solved in just two or three lines of code.
Let’s see how we can use the superpower of modules.
You can use a Python module with the help of the import statement. Include ‘import module_name‘ at the beginning of the code. Then you can use the functions and values available in the module using the dot operator.
Consider an example of using the ‘random’ module.
import random
for i in range(5):
value= random.randint(90,99)
print(value)
The output of this code will be some other random values between 90 and 99.
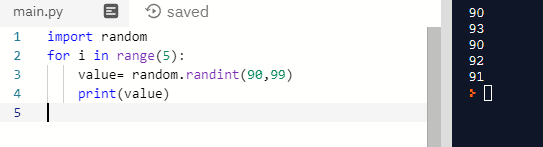
If you need only certain methods or values from a module, you can use the format: ‘from module_name import function_name’.
For example :
from math import sqrt,pi
By using the ‘as’ statement, we can import a module under a different name. This can be used when a module or function has a long or confusing name.
For example :
from math import sqrt as square_root
Trying to import a module that is not available will cause ImportError.
There are three main types of modules in Python: those you write yourself, those you install from external sources, and those that are pre-installed with Python.
Standard Library in Python
The modules that are pre-installed with Python constitute the standard library. Some of the standard library’s useful modules are string, turtle, math, random, datetime, os, multiprocessing, socket, email, json, doctest, etc.
Python’s standard library is one of its main strengths as a programming language.
Dictionaries in Python
Dictionaries are a special type of data structure in Python. They are used to store key-value pairs. So if you put in a key, it gives back the value.
The syntax of dictionaries would look like this:
{ key1: value1, key2 : value,…. }
For example, if we want to create a phonebook, then we will create a dictionary like this.
phone_book = {
'John' : '8592000000',
'Bob' : '7994000000',
'Elisa' : '9749777777'
}
After that, if we go to the contacts and type in the name, it will give you the phone number.
print(phone_book['John'])
Then, the output will be: ‘8592000000’
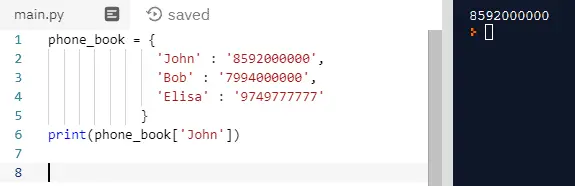
What if we want to store more values along with phone numbers, say we want to add email too? Then, we use lists inside dictionaries.
phone_book = {
'John' : ['8592000000', 'john@xyzmail.com'],
'Bob' : ['7994000000', 'bob@xyzmail.com'],
'Elisa' : [ '9749777777' , 'elisa@xyzmail.com']
}
print(phone_book['John'])
The output of this code will be: ‘8592000000’, ‘john@xyzmail.com’
What if we want only the phone number of John from this dictionary? Then we use the index of the list.
Let’s look at an example:
print(phone_book['John'][0])
The output will be: ‘8592000000’
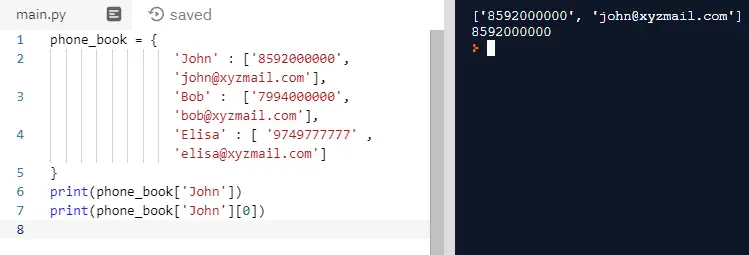
Adding a new item into the dictionary
Let’s see how we can add a new key-value to this dictionary. We can do this by writing the following line of code.
phone_book[ 'Adam' ] = '9567974800'
Now, imagine that one person in your phonebook changed his phone number to another one. So, in that case, we want to update our dictionary, right? Let’s see how we can do this.
phone_book[ 'John' ] = '1567933400'
Now, John’s phone number is changed to ‘1567933400’.
Looping through a dictionary
We can loop through a dictionary using the following syntax.
for k,v in phone_book.items():
print(k, ":", v)
Here, k and v represent key and value respectively.
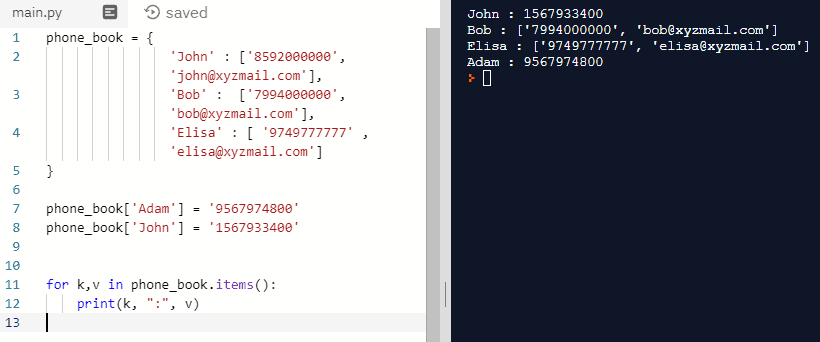
Tuples in Python
Tuples are very similar to lists, except that they are immutable, that is, they can’t be changed once defined. Also, they are represented using parentheses rather than square brackets.
Let’s look at an example of a tuple.
exam_register_numbers = ('15001147' , '15001148' )
If you want to access the values in the tuple, you can do that using the corresponding indices.
For example :
print(exam_register_numbers[0])
The output will be: ‘15001147’.
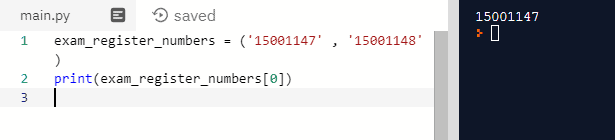
Trying to change a value in the tuple causes TypeError since they are immutable. Tuples can be nested within each other, just like lists. Tuples are faster than lists.
Mutable vs Immutable Data Types
Mutable data types are those that can be changed. For example, lists are mutable.
Let’s see an example :
countries = [ 'India' , 'Poland' , 'Germany' ]
countries[2] = 'U.S.A'
print(countries)
The output for this code will be ‘India’, ‘Poland’, ‘U.S.A’
Immutable data types are those that can’t be changed. For example, tuples are immutable.
Let’s look at the example:
ucl_semifinal = ('Real_Madrid', 'Liverpool' , 'Bayern_Munich' , 'Roma' )
ucl_semifinal[3] = 'Barcelona'
print(ucl_semifinal)
In this case, the second line shows a TypeError.
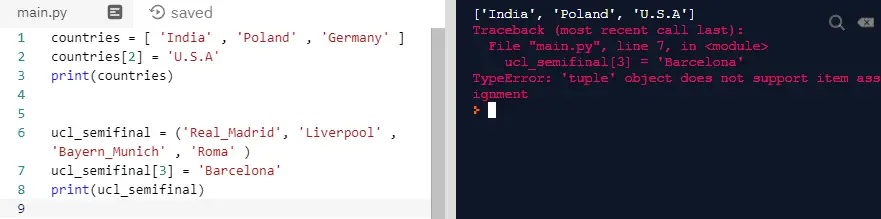
If you are a Barcelona football club fan, don’t take it seriously. I just wrote this code to show you an example.
Sets in Python
A set is an unordered collection of items. All the elements in a set are unique and immutable. However, the set itself is mutable. We can insert or delete items from it if we want.
A set is created by placing all the items inside curly braces, and the items are separated by a comma.
For example:
groceries = { 'apple', 'milk', 'chicken'}
A set can have different types of items. We can’t access or change an element of a set using indexing or slicing. Sets in Python do not support it.
We can add a single element using add() method and multiple elements using the update() method. A particular item can be removed from the set using methods, discard() and remove().
We can remove and return an item using the pop() method (arbitrary item). If we want to remove all items from a set, we can use the clear method for doing that.
groceries = {'apple', 'mango', 'apple'}
print(groceries)
The output will be : {‘apple’,’mango’} , since every element is unique.

Sets can also perform mathematical set operations like union, intersection, symmetric difference, etc.
For example :
A = {2,3}
B = {1,2}
print(A|B)
print(A&B)
print(A-B)
The output of this program will be :
{1,2,3}
{2}
{3}
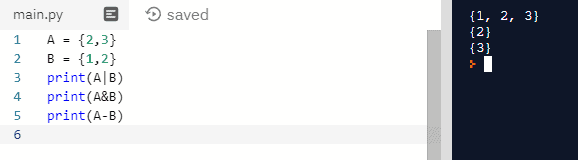
String Formatting in Python
Now, let’s move to the next level of Python basics. A little more advanced concepts like string formatting and exception handling.
String formatting provides a powerful way to combine non-strings within strings. It uses the format() method to substitute a number of arguments in the string.
Let’s see an example.
fruits=['apple','orange','mango']
bucket = "The fruits inside the bucket are : {0} , {1} and {2} .".format( fruits[0],fruits[1],fruits[2] )
print(bucket)
The output of this piece of code will be: ‘The fruits inside the bucket are apple, orange, and mango’.

Each argument of the format() method is placed in the string at the corresponding positions, which is determined using the curly braces {}.
We can also do string formatting with named arguments.
Let’s see an example.
goals= "Ronaldo : {rono} , Messi : {leo} ".format(rono=28,leo=27)
print(goals)
The output will be: ‘Ronaldo: 28, Messi: 27’
Let’s see one more example case.
print("{0}{1}{0}".format("abra","cad"))
The output for this code is ‘abracadabra’.

Conclusion
It was a long tutorial. A thumbs up to you if you have followed it completely till the end. I hope this article was helpful. You can bookmark this article to use it as a reference. Whenever you have any doubts, you can come back to them and clear your doubts.
If you are comfortable with the basics of Python, I would suggest a few articles you can check out as the next step.
- 35 Python Programming Exercises and Solutions (if you want to practice problems in Python)
- Python OOP Concepts (if you want to learn object-oriented programming in Python)
- A Deep Dive into Python Advanced Concepts (to learn advanced concepts in Python)
You can also start exploring web development, machine learning, desktop app development, etc. Python has a lot of use cases.
If you have any doubts, feel free to comment down below. I’ll be happy to help you. I spent a long time making this article. I would appreciate it if you would be willing to share it. It will inspire me to create more useful tutorials like this.
Happy coding!
very nice article bro…
Thanks.
very useful article.Thanks for writing it Ashwin
Cheers mate!
Good article. Thumbs up.
Cheers man 🙂
Nice article, but i wish you could include environments. How to import, export and work with envs
Thanks again
Thanks for the suggestions man.
It is a very good article! and very useful
Can I become successful as a self taught programmer by hardworking can u guide me ?
msg = “Thank-you”
Print ( msg )
Definitely! You can become a good programmer with hard work!
msg = “All the very best!”
print(msg)
Great post and will help new Python learners like me!!!
There is one small typo under the “Standard library in Python” section you meant to say tuple, not turtle! Thank you again for this blog post!
Hey, thanks! That wasn’t a typo. I actually meant “turtle”. Turtle is a module in Python which used to implement basic graphics.
Very good article. I learned a lot.
Your articles are easy to understand! Thank you.
Glad it helped you. Thanks for the comment.
A= “I commented on this link”
B= “You read this comment”
if A== “I commented on this link” and B== “You read this comment”:
print(“I enjoyed the training. God bless you” )
print(“Thank you so much. Happy coding!”)
Good article. Small notice about insert in python list. From your example I understand that insert method allows inserting a new item in front of a specified index and append method as you mentioned allows adding a new item to the end of the list.
EASIEST EXPLANATION OF THE BASIC TOPICS OF PYTHON.
AMAZING MAN.
print(“effective explanation man!”)
Superb article, 75% my concept is clear….