In Python, we use dictionaries to store data in the form of key-value pairs. You can find the value from the dictionary by searching for the key.
The syntax of dictionaries is as follows:
{ key1: value1, key2 : value2, … }
In this post, I have added some simple examples of using dictionaries in Python. You can check out these examples to understand the working of dictionaries in various scenarios. Let’s dive right in.
Example of a dictionary in Python
alphabetDict = {
"a":"apple",
"b" :"ball",
"c":"cat",
"d":"doll"
}
print(alphabetDict)

2. Python Dictionary with duplicate items
In Python, dictionaries do not allow duplicate keys.
num_dict = {
1:11,2:22,2:22,3:33,3:33,3:33
}
print(num_dict)

Python dictionaries allow duplicate values.
num_dict = {
1:11,2:22,3:22,4:33,5:33,6:33
}
print(num_dict)

3. Clearing all keys and values in a Python dictionary
clearDict = {1:"python",2:"csharp",3:"php"}
print("Before clearing the dictionary:",clearDict)
clearDict.clear()
print("After clearing the dictionary:",clearDict)

4. Getting the value from a Python dictionary based on the key
programmingLangauges = {1:"Python",2:"CSharp",3:"PHP"}
#if value exists, it returns the value
print(programmingLangauges.get(1))
#if value doesn't exist, it returns None
print(programmingLangauges.get(4))
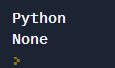
5. Getting the keys and values from a dictionary using items() method
programmingLangauges = {1:"Python",2:"CSharp",3:"PHP"}
print(programmingLangauges.items())

6. Getting the keys from a dictionary using keys() method
programmingLangauges = {1:"Python",2:"CSharp",3:"PHP"}
print(programmingLangauges.keys())
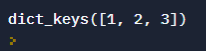
7. Getting the keys from a dictionary using values() method
programmingLangauges = {1:"Python",2:"CSharp",3:"PHP"}
print(programmingLangauges.values())

8. Popping out items from a Python dictionary using pop() method
The method pop(key) removes the key from the dictionary and returns its value.
programmingLangauges = {1:"Python",2:"CSharp",3:"PHP"}
print(programmingLangauges.pop(3))
print(programmingLangauges)
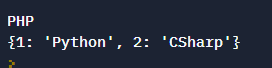
9. Converting two lists to a dictionary in Python
letters = ['a','b','c','d']
numbers = [1,2,3,4]
myDict = dict(zip(letters,numbers))
print(myDict)

10. Deleting elements from a dictionary using del
programmingLangauges = {1:"Python",2:"CSharp",3:"PHP"}
if 3 in programmingLangauges:
del programmingLangauges[3]
print(programmingLangauges)

11. Sorting elements in a Python dictionary
programmingLangauges = {1:"Python",5:"Java",3:"PHP"}
for key in sorted(programmingLangauges):
print(key,programmingLangauges[key])
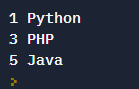
12. Finding the sum of all values in a Python dictionary
myDict = {"n1":100,"n2":200,"n3":300}
print(sum(myDict.values()))
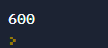
13. Example of the fromkeys() method in Python
In Python, the fromkeys()
method returns a dictionary with the specified keys and the specified value.
p = ("p1","p2","p3")
q = 5
myDict = dict.fromkeys(p,q)
print(myDict)

14. Finding the min and max of values in a Python dictionary
myDict = {"n1":100,"n2":200,"n3":300}
print(max(myDict.values()))
print(min(myDict.values()))
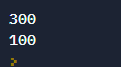
15. Python dictionary of square of numbers
squareDict ={}
for i in range(1,6):
squareDict[i] = i**2
print(squareDict)
