When you learn a web framework or any other technology, it is very important to understand what is happening behind the scenes. Have you ever wondered about what Django is doing when a person types in a URL to visit the website?
I know many people have this doubt, just like I had a few days before. So, let’s try to understand the working of a Django web application when a user makes a web-based request.
We can call the entire process as the Request-Response cycle. Whenever you go to a website, you are making a request. This web request (HTTP request) is made by a user assuming that they will get a response back from the website.
For example, if you type in a URL in your browser, say google.com, you are making a request. After you’ve made the request, you’ll be directed to Google’s homepage. That is a response. When you reach the homepage, say you’ve clicked a button. That is again a request. So the cycle continues until you close the website.
If you’re running a Django project on your command line, you’ll be able to see all the requests that you make.
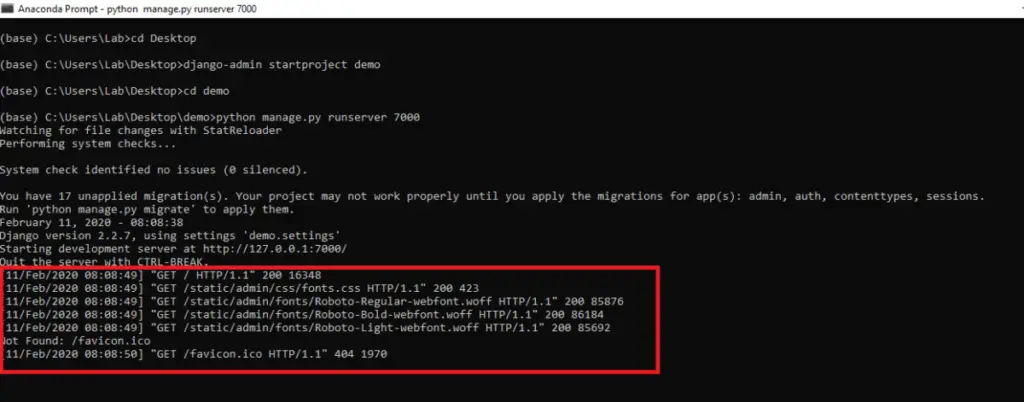
These are all the requests that were made when I run a Django project in my localhost. When I click a button or do something else in the browser, the requests made will be displayed in the command line.
So how does Django handle web-requests? Let’s have a look at the scenes happening behind.
How Does Django Handle an HTTP Request?
Here is a diagram that shows the entire process:
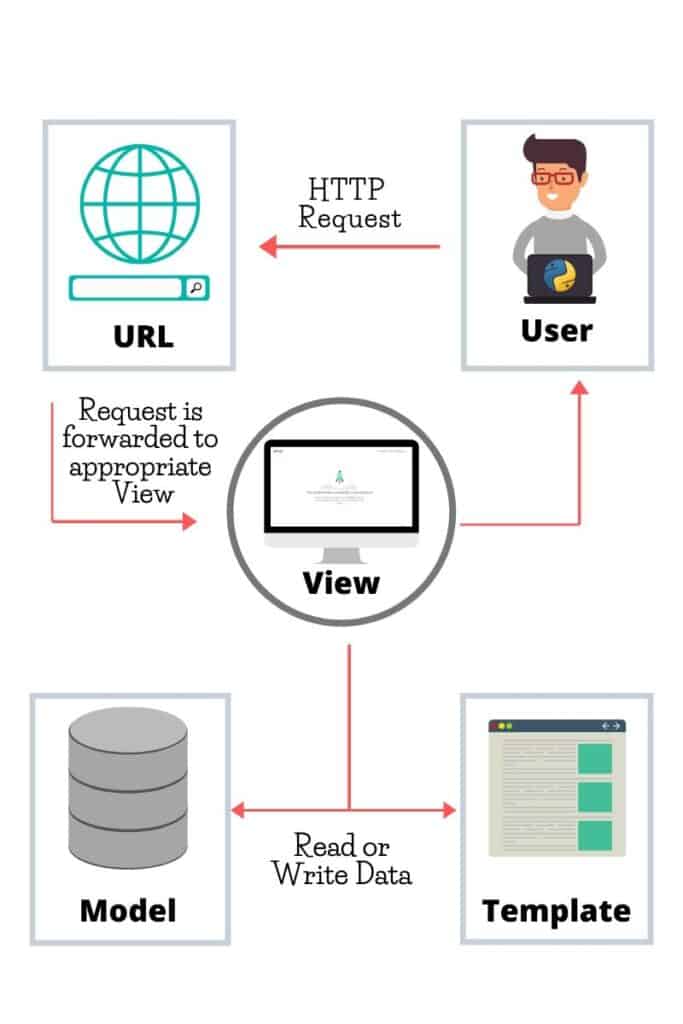
First of all, the user types in a URL to get results. Any request made on a Django web application is checked in the urls.py file. If the requested URL matches with a URL pattern in the file, then it is mapped to a corresponding view.
Now, the execution is passed to the views.py file. The method is then executed, which generates a response, which can be a simple HTTP response or any template, with or without context variables.
If the requested URL is not matched, then Django creates a not found error and redirects to a 404 error page.
As you might know, Django follows an MVT architecture (Model-View-Template). Let’s have a look at each part to understand what they do.
URL
URL part of a Django application (urls.py file) handles all the HTTP requests. The URL mapper checks the HTTP requests and maps them to the corresponding views. The view can be a python function or a class-based view.
When a user browses a URL, Django unravels the URL by using URL patterns. For example, take a look at a few examples of URL patterns.
from django.urls import path
from . import views
urlpatterns = [
path('', views.ArticleListView.as_view(), name='home'),
path('article/<int:pk>', views.ArticleDetailView.as_view(), name='detail'),
]
You can see that for two different URL patters, corresponding views are called. You don’t need to worry about understand this piece of code. I just put it here to show an example.
Here are a few fundamental things you need to understand from this example:
- Each URL pattern is written using path() or re_path() methods.
- Angle brackets are used to take a particular value from the URL.
- When a URL matches a pattern, the corresponding view method is called to generate the response.
If you want to learn more about URLs in Django, check out the official Django docs here.
View
A view is a Python function or class that takes an HTTP request and returns an HTTP response. The response can be anything, an HTML webpage, a redirect, an image, or even a 404 error.
The view contains the logic needed to return these responses. Views are conventionally written in a file named views.py. A view can access data via models and HTML web pages via templates. You can check out the Django documentation to learn more about views.
Models
Models are Python objects that contain information about your data. Models define the data structure of a web application. Generally, each model maps to a single database table. Models provide options to create, edit, update, and query data records in the database. You can learn more about models here.
Templates
Templates represent the structure of a file layout to represent data in a web page. They provide a convenient way in Django to generate HTML dynamically. Templates consist of the static parts of a web page along with the dynamic content that can be inserted.
You can configure a Django project with zero or one or several template engines based on your requirements. If you want to learn more about Django templates, check out the documentation.
Requests and Responses in Django
Understanding the Request-Response cycle in Django is really important. It is a fundamental concept that you need to grasp so that you’ll know the working of every web application you build.
I hope you got a basic idea about how Django handles a web request. If you aren’t yet clear with the Request-Response cycle mechanism, you can watch the following YouTube video to get a better understanding of things.
Django has a lightweight plugin that processes during request and response execution, which is called middleware. Middleware is used to perform a function in the web application. Django provides numerous built-in middleware and also lets us create our own middleware.
Can Django Handle Multiple Requests at the Same Time? Actually, Django handles only one request at a time. It processes a single request and considers the next one only after the first request is completed.
Conclusion
When a user requests a URL, Django checks the URL pattern, calls a view method if the pattern matches and the view returns an HTTP response. That is how Django handles a web request.
I hope this article was helpful to you. If you have any doubts or anything to add to this article, feel free to put your thoughts down in the comments section.
I would appreciate it if you would be willing to share this article. It will encourage me to create more useful tutorials like this.
Happy Coding!
The article is very descriptive! Tanks!!!
Really Good knowledge for the Beginner. I have also doubt previously but know this is clear because of you.