Functions allow us to use a block of statements multiple times without writing the code again and again. Once you define a function, you can call the function name whenever you want to use it.
In Python, we can create our own functions by using the def keyword. The syntax is as follows.
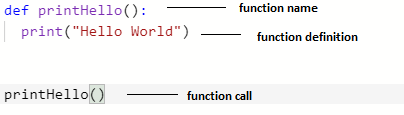
In this post, I have compiled a list of examples of functions in Python. Check out these examples and understand how functions work in various scenarios. I hope this will help you get a clear picture of Python functions. Let’s dive right in.
1. Python function that prints a text
The statements in the function get executed only when we call the function. The function definition should be written before the function call.
# creating a function using def keyword
def pythonista_function():
print("Welcome to the Python world")
#calling the function
pythonista_function()

2. Python function that accepts two numbers as arguments and returns the sum
Functions can take arguments (one or more). Functions can return a value to the main method. To do this, we can use the return statement.
def addNumbers(x,y):
sum = x + y
return sum
output = addNumbers(12,9)
print(output)
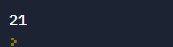
3. Python function that accepts different values as parameters and returns a list
def myFruits(f1,f2,f3,f4):
FruitsList = [f1,f2,f3,f4]
return FruitsList
output = myFruits("Apple","Bannana","Grapes","Orange")
print(output)

4. Python function that returns a dictionary
def myAnimals(a1,a2,a3):
Animalgroup = {'Kitten':a1,'Puppy':a2,'Pup':a3}
return Animalgroup
output = myAnimals("Cat","Dog","Rat")
print(output)

5. Python function that returns a tuple
def myVeggies(v1,v2,v3):
vegtuple = (v1,v2,v3)
return vegtuple
output = myVeggies("Carrot","Potato","Tomato")
print(output)

6. Python function that accepts a list as a parameter
def myChocolates(cList):
for i in cList:
print(i)
chocolateList = ["Dairy Milk","Snickers","Kitkat"]
myChocolates(chocolateList)
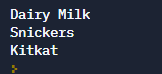
7. Python function that accepts a dictionary as a parameter
def myVehicles(vDict):
print(vDict)
vehicleDictionary = {
'Tesla': 'Car',
'Royal Enfield' : 'Bike'
}
myVehicles(vehicleDictionary)

8. Python function using positional arguments
Here, the order in which arguments are passed matters.
def Car(name,model):
print(name)
print(model)
Car("Audi","Q7")
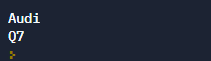
9. Python function using keyword arguments
Here, the order of function arguments doesn’t really matter. You can assign the parameters that you want to pass to the respective keyword arguments.
def Car(name,model):
print(name)
print(model)
Car(model="Q7",name="Audi")
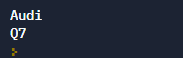
10. Python function using default arguments
If there is a default value for the arguments, then it is not mandatory to pass that parameter while calling the function.
def Car(name,model="Q7"):
print(name)
print(model)
Car("Audi")
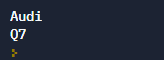
def Car(name,model="Q7"):
print(name)
print(model)
Car("Audi","Q8")
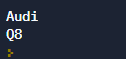
11. Python function using optional arguments
Here, passing the parameter becomes optional.
def Calendar(year,month,date=''):
print(year,month,date)
Calendar(2023,2)
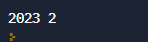
def Calendar(year,month,date=''):
print(year,month,date)
Calendar(2023,2,14)

12. Passing an arbitrary number of arguments to a Python function
def Insects(*Insectslist):
print(Insectslist)
Insects("Mosquito","Honeybee")
Insects("Mosquito","Honeybee","Beetles")

13. Python function that returns multiple values
def SwapTwoNumbers(a,b):
print("Before Swap: ",a,b)
a = a+b
b = a-b
a = a-b
return a,b
a,b = SwapTwoNumbers(17,24)
print("After Swap: ",a,b)
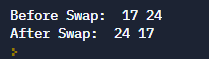
14. Python function to check if a number is palindrome or not
def palindromeCheck(num):
temp = num
rev = 0
while(num != 0):
r = num%10
rev = rev*10+r
num = num//10
if(rev == temp):
print(temp, "is a palindrome number")
else:
print(temp, "is not a palindrome number")
palindromeCheck(131)
palindromeCheck(34)
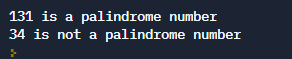
15. Python function to find the factorial of a number
def factorial(n):
fact = 1
while(n!=0):
fact *= n
n = n-1
print("The factorial is",fact)
inputNumber = int(input("Enter the number: "))
factorial(inputNumber)
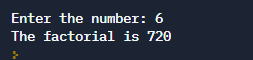
16. Python nested functions
def function1():
p ="Hello Pythonista"
def function2():
print(p)
function2()
function1()

great give me more?
great site More! More! More!
great sit for learning
great were can i get more?
VERY VERY GREAT PROGRAMS
Absolutely Amazing
Can we have more of your programs ??
Sure, I will try adding more.