In computer science, a data structure is a way to store and organize data, and it helps to use the data efficiently. In this article, let’s learn what stack data structure is and how to implement it using Python.
Stack is a data structure that stores items in a Last In First Out (LIFO) manner, which means that the last element inserted inside the stack is removed first. In stack, insertion and deletion of elements are allowed only at one end. The other end is closed.
The process of inserting items into the stack is called push and the process of removing items from the stack is called pop. To understand the working of the stack data structure, have a look at the following diagrams.
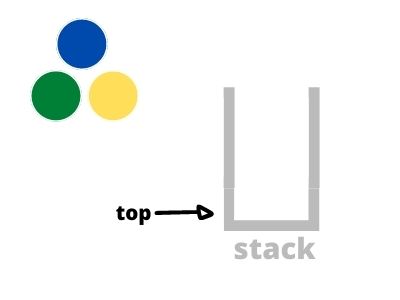
Here, the stack is represented by ash-colored lines. The yellow, green, and blue circles are items that we will use to do push and pop operations.
Let’s say we have an empty stack. We are pushing items into the stack one by one.
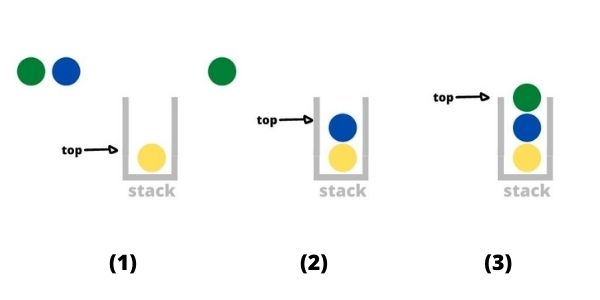
As you can see, we have inserted the yellow item first, followed by the blue item and then the green item. We can use a pointer called top to keep track of the top element in the stack.
Now, let’s see how we can pop these items from the stack. Since it is a LIFO (Last In First Out) data structure, the item that was pushed last will be popped first. If you want to pop the item at the bottom, you must first remove all the items on top.
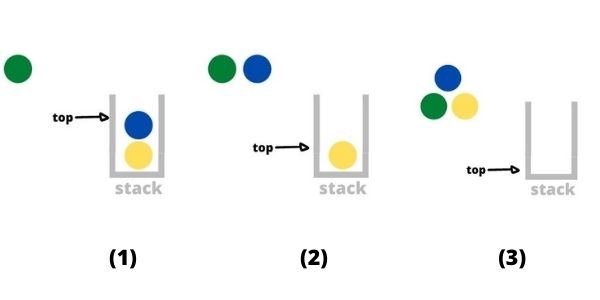
Stack Implementation using Python
Let’s try to implement this data structure in the Python programming language. There are various ways to implement the stack data structure in Python. We will do it in the easiest way, which is by using a list.
The list is an in-built data structure in Python, and it can be used as a stack. We can use the append() method to push elements to the top of the stack. We also have the pop() method which removes the items in LIFO order.
Given below is the Python code that implements the stack data structure.
stack = []
max_size = int(input("Enter the max limit of the stack: "))
while True:
print("What do you want to do? ")
print("1. Push")
print("2. Pop")
print("3. Exit")
choice = int(input("Enter your choice: "))
if choice == 1:
if(len(stack) == max_size):
print("Stack is full.")
else:
value = input("Enter the value: ")
stack.append(value)
print('Stack after elements are pushed:', stack)
elif choice == 2:
if(len(stack) == 0):
print("Stack is empty.")
else:
stack.pop()
print('Stack after elements are popped:', stack)
elif choice == 3:
break
else:
print("Invalid choice.")
print()
Output:
Enter the max limit of the stack: 2
What do you want to do?
1. Push
2. Pop
3. Exit
Enter your choice: 1
Enter the value: Red
Stack after elements are pushed: ['Red']
What do you want to do?
1. Push
2. Pop
3. Exit
Enter your choice: 1
Enter the value: Blue
Stack after elements are pushed: ['Red', 'Blue']
What do you want to do?
1. Push
2. Pop
3. Exit
Enter your choice: 1
Stack is full.
Stack after elements are pushed: ['Red', 'Blue']
What do you want to do?
1. Push
2. Pop
3. Exit
Enter your choice: 2
Stack after elements are popped: ['Red']
What do you want to do?
1. Push
2. Pop
3. Exit
Enter your choice: 2
Stack after elements are popped: []
What do you want to do?
1. Push
2. Pop
3. Exit
Enter your choice: 2
Stack is empty.
Stack after elements are popped: []
What do you want to do?
1. Push
2. Pop
3. Exit
Enter your choice: 3
If you haven’t understood the working of stack yet, check out this online tool, where you can visualize the concepts and play around with stack.
I hope this article was helpful. Check out my articles on queues and linked lists in Python.