A queue is a data structure in computer science, and its concept is very similar to the queues that we see in real-life scenarios. For example, we see queues of people at ticket windows, shops, etc.
In a queue, the item that enters first exists first, which means that it is a First In First Out (FIFO) data structure. Insertion of elements happens at one end (rear-end) and deletion of elements happens at the other end (front-end).
The process of inserting data into a queue is known as enqueue, and the deletion of elements from a queue is called dequeue.
We use a pointer called head to denote the front of the queue and another pointer called tail to denote the rear of a queue.
To understand the working of the queue data structure, check out the following diagrams. Here is a graphical representation of a queue:
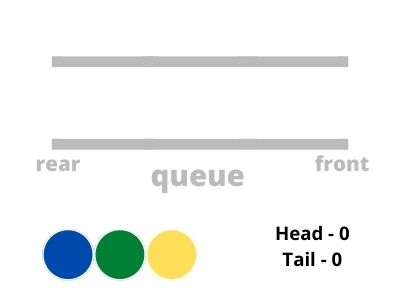
Here, the queue is represented by the ash-colored lines. The yellow, green, and blue circles are items that we will insert into the queue.
Initially, we have an empty queue. Let’s insert the items into the queue one at a time.
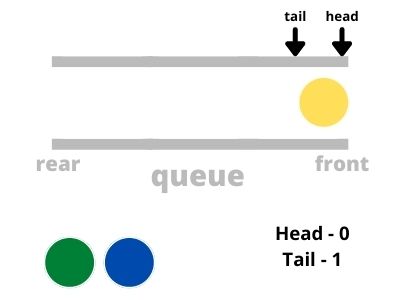
We have inserted one item into the queue through the rear-end. Now, the value of tail is incremented by one. The value of head remains the same.
Now, let’s insert the other two items in the same way.
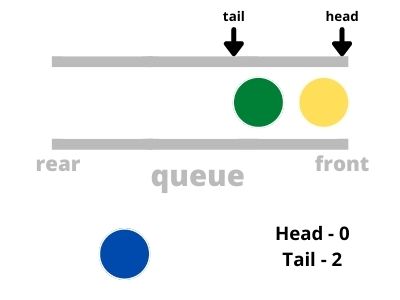
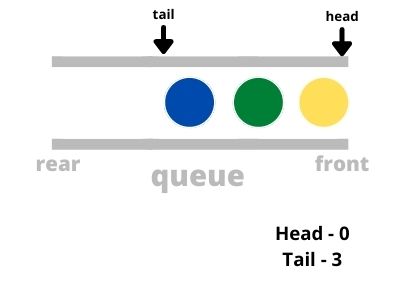
As you can see, we have inserted the yellow item into the queue first, followed by the green item and then the blue item.
Now, let’s see how we can delete items from the queue. Since it is a FIFO (First In First Out) data structure, the item which was inserted first will be deleted first.
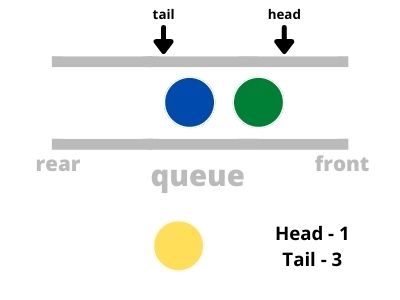
You can see that when the first item was deleted, the value of head was incremented by 1. Now, the item at the front end is the green circle. Let’s delete the other two items as well.
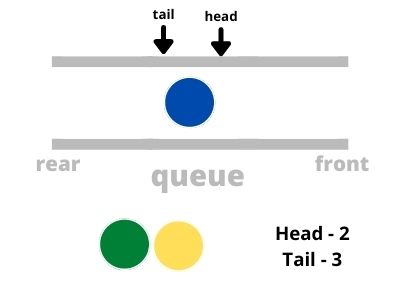
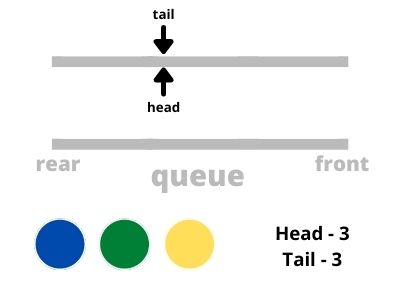
When all the three items got deleted from the queue, the value of head and tail became “3”. The queue is said to be empty when the value of head is equal to the value of tail.
Queue Implementation using Python
Let’s implement the queue data structure in the Python programming language. Python provides a lot of ways to implement this data structure. Now we will do it by using the queue module in Python.
We can use the put() method to insert elements into the queue. We also have the get() method to delete items from the queue in FIFO order.
The Python code that implements the queue data structure is given below.
from queue import Queue
myQueue = Queue()
print(myQueue.queue)
#inserting items into the queue
myQueue.put("Yellow")
myQueue.put("Green")
myQueue.put("Blue")
print(myQueue.queue)
#deleting items from the queue
myQueue.get()
print(myQueue.queue)
myQueue.get()
print(myQueue.queue)
myQueue.get()
print(myQueue.queue)
Output:
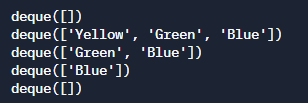
You can check out this online tool to visualize the concepts of the queue data structure.
If this article was helpful, check out my articles on stacks and linked lists in Python.