Shell scripting is writing a computer program that is designed to be run by the Unix/Linux shell. A shell script is a code that contains series of commands for the shell to execute.
Even though it is designed to be run on Unix or Linux operating systems, we can run shell scripts on Windows or Mac using software like GitBash, Cygwin, or the Windows Linux subsystem. You can also use the help of online editors to run shell scripts. One online editor I use is ReplIt.com, where you can create a new Bash repl and run shell scripts.
Why is shell scripting important? Well, shell scripting is pretty important if you are considering system admin roles, DevOps, managing Linux servers, or other IT roles.
In this article, I’ll walk you through the basics of shell scripting so that you can get familiarized with its syntax and rules. Let’s dive right in.
Shell Scripting
Shell scripts are lists of commands which are listed in the order of execution. A shell script program file ends with the extension “.sh”. Shell scripts are interpreted, not compiled.
Printing Hello World
If you want to execute shell scripts, install Cygwin or GitBash if you don’t have Linux. Then open up your Cygwin or GitBash shell and create a new file with the extension “.sh” using the touch command.
touch hello.sh
Now, open that file using any text editor and type in the following code to print “Hello World”.
echo "Hello World"
Now, come back to the shell and give execute permission to that file. You can do that using the chmod command.
chmod +x hello.sh
And, finally, type in the following command to execute the script.
./hello.sh
Now, you’ll be able to see “Hello World” on the screen. You can see the following screenshot of running the script using the Cygwin software.
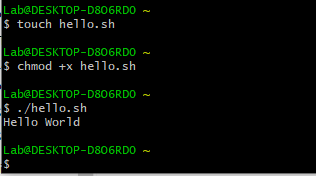
You can run shell scripts on online editors too. Open a new “Bash” repl on replit.com and run the code.
echo Hello World
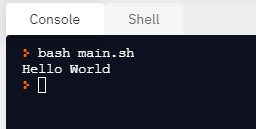
Variables
In shell scripting, we can use variables to store data. There are some rules that you must follow while creating variables.
- Variable names can contain only letters (either small (a-z) or capital (A-Z)), numbers (0-9), and underscore(_).
- Unix shell variables are named in UPPERCASE letters by convention.
- Variable names shouldn’t start with a number.
- Some examples of correct variable names are VAR1, _NAME, AGE, etc.
- Variables can be scalar (that contains only a single value) or array (that contains a list of values).
- To access a value stored in a variable, you need to prefix the variable name with a dollar symbol ($).
- You can assign values to a variable using the assignment operator (=).
- When you assign a value to a variable, there shouldn’t be any spaces on either side of the “=” symbol.
Let’s see an example for defining a variable and printing it.
NAME="John"
echo $NAME
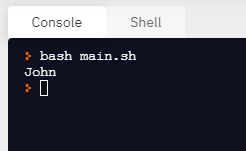
Reading Inputs
To read user inputs from the console, you can use the keyword “read” followed by a space and the variable name.
For example:
echo "What's your name?"
read person
echo "Hello, $person"
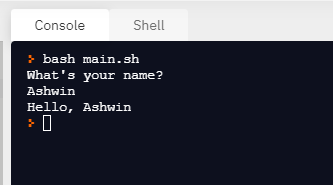
Unsetting Variables
To delete or unset a variable, you can use the keyword “unset”. When you unset a variable, the shell removes it from the list of variables that it tracks. After that, you will not be able to access the stored value in that variable.
NAME="John"
unset NAME
echo $NAME
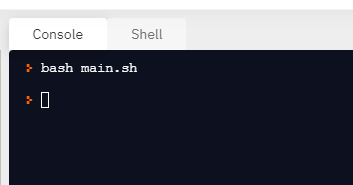
You can see that the value is no longer there when you try to access the variable.
Operators
There are several different operators available in shell scripting, mainly, the arithmetic operators and the relational operators.
Arithmetic Operators
The following are the important arithmetic operators that are used in shell scripting:
+ | Addition |
– | Subtraction |
* | Multiplication |
/ | Division |
% | Modulus |
= | Assignment |
== | Equality |
!= | Non Equality |
Relational Operators
Given below are the mostly used relational operators in shell scripting:
! | Inverts a true condition into false and vice versa |
-o | Logical OR operator |
-a | Logical AND operator |
-lt | Less than |
-gt | Greater than |
-ge | Greater than or equal to |
-le | Less than or equal to |
-eq | Equal to |
Now, let’s see how we can write an expression to do an arithmetic operation. For doing that, you can use the keyword “expr”. The entire expression should be written inside two backticks ` `. Also, note that there should be spaces between operators and expressions. Take a look at an example of adding two numbers.
val=`expr 2 + 4`
echo "Total: $val"
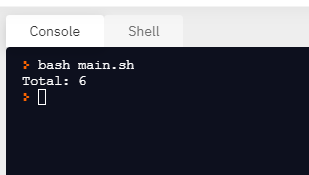
Conditional Statements
Conditional statements are used in programming to make correct decisions and perform the right actions based on the conditions. You can add logic to your program using conditional statements.
If-Else Statements
The main conditional statements in any programming language are the if-else statements. You can check a condition using the if statement, and execute a set of statements if that condition is true. If that condition is false, it will execute the statements under the else condition. You can also check for additional conditions using the else if statements. An if-else block should end with the keyword fi.
In shell scripting, all conditional expressions should be written inside square braces with spaces around each values.
See the following example. In this script, there are two variables with values. The program checks whether both the values are equal, or if one value is greater than the other, or vice versa. Based on the conditional checks, it prints a string as the output.
a=10
b=20
if [ $a -eq $b ]
then
echo "a is equal to b"
elif [ $a -lt $b ]
then
echo "a is less than b"
else
echo "a is greater than b"
fi
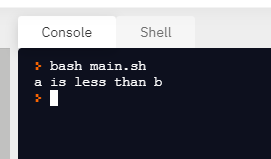
Case Statements
In case statements, the program evaluates an expression, and based on the value of that expression, it executes some statements. The interpreter will check each case against the value of the expression until a matching case is found. If no matching cases are found, a default case will get executed.
The following code snippet is an example for case statements. Here, we have a variable with value “apple”. The case block checks different case expressions to find out a match. If it finds a match, it will execute the statements given along with it. In shell scripting, the symbol “;;” is used to as a break. When it is executed, the program flow will jump to the end of the entire case block.
The * symbol is used for default case. Each case block will end with the keyword “esac”.
FRUIT="apple"
case "$FRUIT" in
"apple") echo "I like Apples"
;;
"banana") echo "Bananas are yummy"
;;
*) echo "No fruit found"
;;
esac
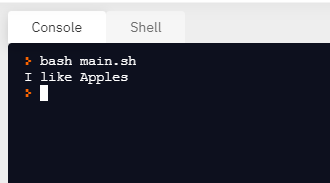
Loops
In programming, loops are used to execute a set of statements multiple times without writing the same code again and again. There are different types of loops in shell scripting, like while loop, until loop, and for loop.
While Loops
In while loops, we use the keyword “while” and a check condition. If that condition is true, the statements inside the while block will be executed. The statements inside a while loop will start with the keyword “do” and end with the keyword “done”.
Let’s see an example in which we use a while loop to print numbers starting from 0 to 10 (excluding 10). Loops help us to execute this easily instead of writing print statements 10 times.
a=0
while [ $a -lt 10 ]
do
echo $a
a=`expr $a + 1`
done
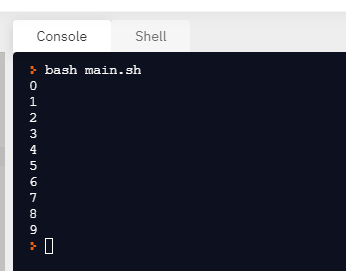
Until Loops
The until loop is pretty similar to the while loop. The only difference is that until block executes the statements when its conditional expression is false, and while block executes the statements when its conditional expression is true.
You can see the following example of until loop in shell scripting.
a=0
until [ $a -eq 10 ]
do
echo $a
a=`expr $a + 1`
done
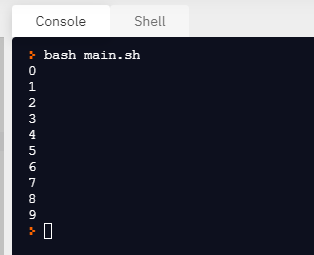
For Loops
The for loop is mainly used to operate on lists of items. The for loop repeats a set of statements for every item in a list.
You can see the following example in which the for loop is used on a list of numbers starting from 0 to 6.
for var in 0 1 2 3 4 5 6
do
echo $var
done
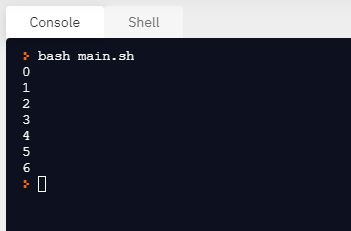
Infinite Loops
An infinite loop is a loop that never stops its execution because the terminating condition is never met.
To create an infinite loop, add a condition that will never become successful. You can see the following example in which an infinite until loop is executed.
a=10
until [ $a -lt 10 ]
do
echo $a
a=`expr $a + 1`
done
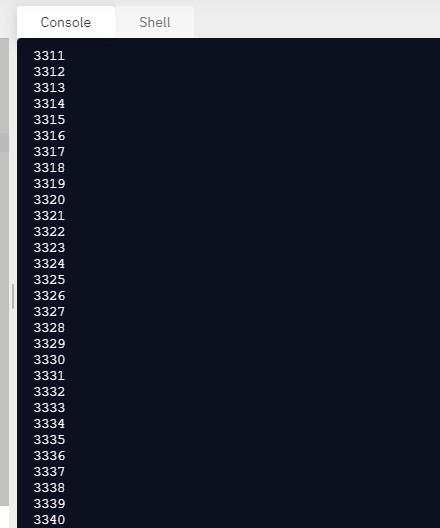
When you run the code, you can see that the program will keep on running forever, until you manually stop it.
Break and Continue
Sometimes, we might want to stop the flow of the loop completely or skip the current iteration of the loop. The break and continue statements are used for that purpose.
The break statement is used to stop the execution of a loop before it actually ends. You can add a condition and then execute the break statement to stop the execution of the loop when that condition occurs. Take a look at the following example. Here, the loop is terminated when the value of a becomes 5.
a=0
while [ $a -lt 10 ]
do
echo $a
if [ $a -eq 5 ]
then
break
fi
a=`expr $a + 1`
done
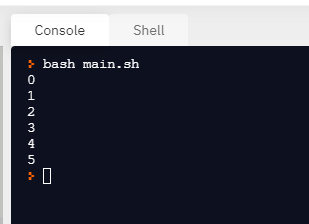
The continue statement is also used for a similar purpose. The difference is that continue statement will only skip the current iteration of the loop. The next iterations will happen as defined in the loop.
See the following example. Here, I’ve used the continue statement when the value of a becomes 5. So it will skip that iteration and continue the loop with 6 as the value of a. In the output, you can see that the value 5 is not printed.
a=0
while [ $a -lt 10 ]
do
if [ $a -eq 5 ]
then
a=`expr $a + 1`
continue
fi
echo $a
a=`expr $a + 1`
done
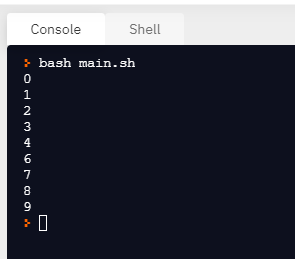
Final Thoughts
That was just the basics of Unix shell scripting. You can use this article as a reference to learn the syntax of shell scripting. If you want a video explanation of the basics of shell scripting, I would recommend you check out this tutorial from Traversy Media.
If you want to get an overview of the Unix operating systems and understand the basic commands, check out this article.
I hope this article was helpful to you. Happy coding!