A servlet is a Java class that is processed on the server. This Java class can generate HTML and return it to the browser. You can use servlets to read HTML form data, use cookies and sessions, etc.
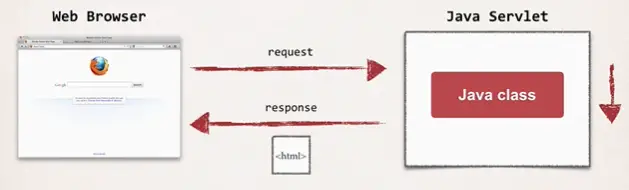
Servlets are similar in functionality to JSP. But there are some differences between servlets and JSP. The table below shows the differences between JSP and Servlets.
JSP | Servlet |
---|---|
HTML file with .jsp extension | Java class file |
Contains static HTML and JSP generates dynamic HTML | Generates all HTML |
Has built-in JSP objects | More work to access web objects |
You can build an entire website only using either JSP or Servlets. In general, it is comparatively easier to write code in JSP. Then, why do we use servlets? The problem with creating an entire website using JSP is that you will be mixing the presentation layer and the business layer together.
It is always better to use both JSP and servlets together. You can use JSP to handle the presentation view whereas servlet will do the business logic. This is a popular design pattern and is called Model View Controller (MVC).
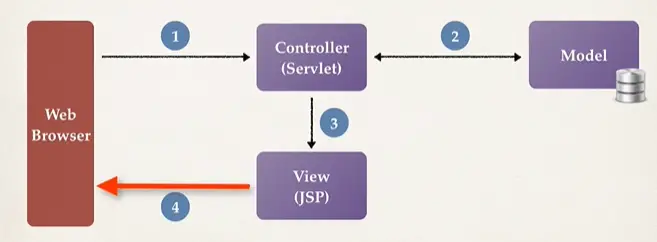
Eclipse and Tomcat
To create a servlet project, we can use Eclipse as the IDE and Tomcat as the application server. Once you install Eclipse IDE on your laptop, go ahead and download Tomcat and place it in your system.
Launch the Eclipse IDE.
Window -> Show View -> Servers -> Open
Create a new Tomcat Server here.
Click the given link and add a runtime environment by opening the folder where you have Tomcat software -> Select Apache -> Tomcat server version -> Next -> Finish.
Once it is done, you will see the Tomcat server in the Servers Window. You can right-click and start the server.
Hello World Project using Servlet
Let’s go ahead and create a project inside the Eclipse IDE.
File -> New -> Dynamic Web Project -> Give a project name -> Next -> Change the "src" folder if you want -> Make the content directory as "WebContent" -> Checkmark the Generate.web.xml deployment descriptor option -> Finish.
Now we have a project folder structure. Now, let’s create a servlet file.
Right-click on the project folder -> New -> Servlet -> Give a package name (e.g: com.servletdemo) and class name (e.g: HelloWorldServlet) -> Next -> Next -> Finish.
Eclipse will create a new servlet file inside the src folder. Given below is the directory structure of this project.
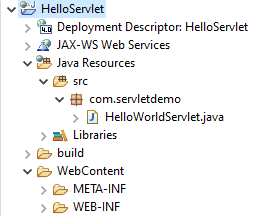
The HelloWorldServlet.java file will have some auto-generated code.
To generate the HTML content, we will override the doGet() method and provide the response from the server when the browser makes a request.
Inside the doGet() method, we will have to do 3 steps to generate a response.
- Set the content type for the response object.
- Import java.io.PrintWriter and create a PrintWriter object.
- Generate the HTML content.
Let’s add the following code to the doGet method in our servlet. You can keep the remaining auto-generated code in the file as it is.
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
out.println("<h2>Hello from Servlet</h2>");
out.println("The current time is: "+ new java.util.Date());
out.println("</body></html>");
}
Let’s run this file after making the above changes to the doGet() method.
Here is the full code:
package com.servletdemo;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/HelloWorldServlet")
public class HelloWorldServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public HelloWorldServlet() {
super();
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
out.println("<h2>Hello from Servlet</h2>");
out.println("The current time is: "+ new java.util.Date());
out.println("</body></html>");
}
}
Run the servlet using the Tomcat server.
Right-click -> Run As -> Run on Server -> Select the Tomcat Server -> Next -> Finish
Output:
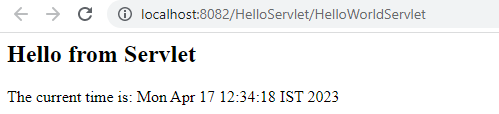
Reading Form Data using Servlet
Now let’s try to read some HTML form data using Servlet. For that, let’s create an HTML page (myform.html) inside the WebContent folder.
This HTML page will have a form that reads the first name and last name of the user. The form will use the GET method to send data.
<html>
<head>
<title>User Form</title>
</head>
<body>
<form action="FormServlet" method="get">
FirstName: <input type="text" name="firstName"> <br> <br>
FirstName: <input type="text" name="lastName"> <br> <br>
<input type="submit" value="Submit">
</form>
</body>
</html>
Now let’s create a servlet called FormServlet.java to handle the data that the form sends. We will create a new Servlet file inside the com.servletdemo package.
Let’s make changes to the doGet() method in this servlet file. Here is the updated code for the doGet() method.
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
out.println("The name of the user is: "
+ request.getParameter("firstName") + " "
+ request.getParameter("lastName")
);
out.println("</body></html>");
}
Once the servlet code is updated, go ahead and run the myform.html file using the Tomcat server.
Output:
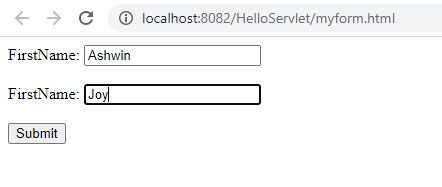

The GET and POST methods are used to transfer data from the browser to the server in the HTTP protocol. In GET, the form data is added to the end of the URL.
In the POST method, the form data is passed in the body of the HTTP request. You won’t see the data in the browser’s URL. If you have some sensitive data, you can use the POST method.
The form GET method calls the doGet() method in the servlet. Similarly, the form POST method calls the doPost() method.