Let’s create a Rock Paper Scissors game (Stone Paper Scissors game) using the Tkinter library of Python.
I guess you already know what the game is all about since it is a famous game around the world. In the place where I’m living, the game is popularly known as Stone-Paper-Scissors and is played very often by the children using their hands.
Game Rules
In case if you don’t know what the Rock Paper Scissors game is, it is a game with three choices: rock, paper, and scissors. Two players can play this game at a time. Each one has to choose from the three options available.
In this app that we make, you are going to play against your enemy, which is the computer. The rules of the game are listed below:
- If one player chooses the rock, and the other chooses the scissor, rock wins.
- If one player chooses the rock, and the other player chooses paper, paper wins.
- If one player chooses the scissor, and the other player chooses paper, scissor wins.
- If both the choices are the same, then no one will win. Both the user and the computer will not get a point.
Now, you know the rules and how to play the game. Without any further introduction, let’s jump right into coding our game.
Let’s Write the Code
First of all, we need to import two libraries into our code, which are tkinter and random.
import random
import tkinter as tk
Now, let’s create a window for our game, and name it Rock Paper Scissors Game.
window = tk.Tk()
window.geometry("400x300")
window.title("Rock Paper Scissors Game")
Next, we are going to define the global variables that we are going to use in our program. We have four of them for storing the user’s score, computer’s score, user’s choice, and computer’s choice. Initially, we set the computer’s score and the user’s score into zero.
USER_SCORE = 0
COMP_SCORE = 0
USER_CHOICE = ""
COMP_CHOICE = ""
Then, we are going to define two methods for converting the user’s choice to a number and vice versa.
def choice_to_number(choice):
rps = {'rock':0,'paper':1,'scissor':2}
return rps[choice]
def number_to_choice(number):
rps={0:'rock',1:'paper',2:'scissor'}
return rps[number]
Now, let’s create a function to get the computer’s choice. We use the random library here. The computer selects a random choice from either rock, paper, or scissors.
def random_computer_choice():
return random.choice(['rock','paper','scissor'])
Next, we are going to create the most important function in our code, the result function, that determines the winner. This function evaluates both user and computer choices, and based on the comparisons, it picks a winner and updates the scores.
Also, let’s create a text area for displaying the current choices and current scores of both the user and the computer.
def result(human_choice,comp_choice):
global USER_SCORE
global COMP_SCORE
user=choice_to_number(human_choice)
comp=choice_to_number(comp_choice)
if(user==comp):
print("Tie")
elif((user-comp)%3==1):
print("You win")
USER_SCORE+=1
else:
print("Comp wins")
COMP_SCORE+=1
text_area = tk.Text(master=window,height=12,width=30,bg="#FFFF99")
text_area.grid(column=0,row=4)
answer = "Your Choice: {uc} \nComputer's Choice : {cc} \n Your Score : {u} \n Computer Score : {c} ".format(uc=USER_CHOICE,cc=COMP_CHOICE,u=USER_SCORE,c=COMP_SCORE)
text_area.insert(tk.END,answer)
Then, we are going to define three methods for three different user choices. These methods take the user’s choice, generate a computer’s random choice, and pass them into the result function, which we created previously.
def rock():
global USER_CHOICE
global COMP_CHOICE
USER_CHOICE='rock'
COMP_CHOICE=random_computer_choice()
result(USER_CHOICE,COMP_CHOICE)
def paper():
global USER_CHOICE
global COMP_CHOICE
USER_CHOICE='paper'
COMP_CHOICE=random_computer_choice()
result(USER_CHOICE,COMP_CHOICE)
def scissor():
global USER_CHOICE
global COMP_CHOICE
USER_CHOICE='scissor'
COMP_CHOICE=random_computer_choice()
result(USER_CHOICE,COMP_CHOICE)
Now, let’s build three buttons so that the user can click them to play the game.
button1 = tk.Button(text=" Rock ",bg="skyblue",command=rock)
button1.grid(column=0,row=1)
button2 = tk.Button(text=" Paper ",bg="pink",command=paper)
button2.grid(column=0,row=2)
button3 = tk.Button(text=" Scissor ",bg="lightgreen",command=scissor)
button3.grid(column=0,row=3)
Finally, let’s run everything inside the window using the mainloop() method.
window.mainloop()
After you have coded all the above in the correct order, run this on your IDLE or command line.
Then you will get an interface with three buttons: Rock, Paper, and Scissor. You can then click any of those buttons to start the game.
You need to click a button to select your choice. Then, the computer will select its own choice automatically.
The functions in our code will compare both these choices, and then it will give one point to the winner of that round.
Let’s take a quick look at the complete Python code we wrote to build our rock paper scissors game.
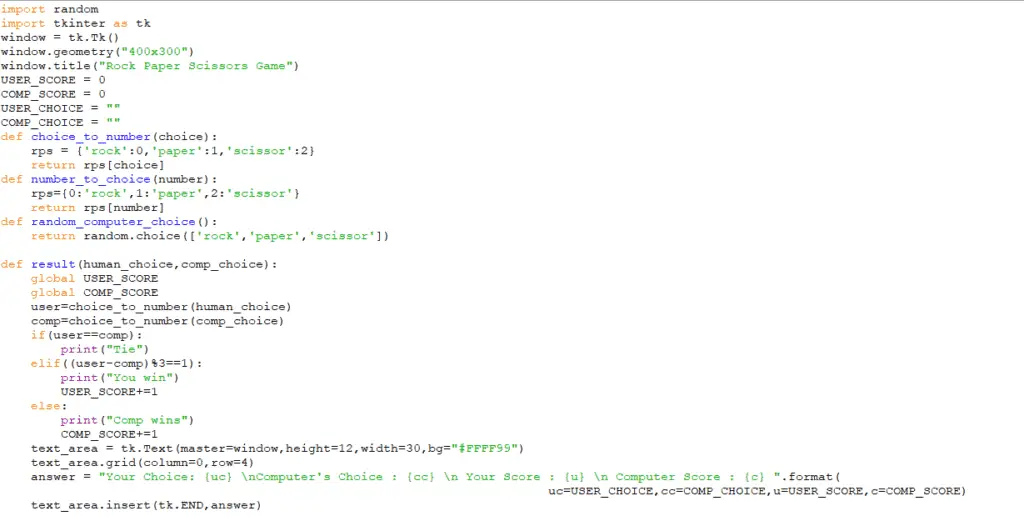
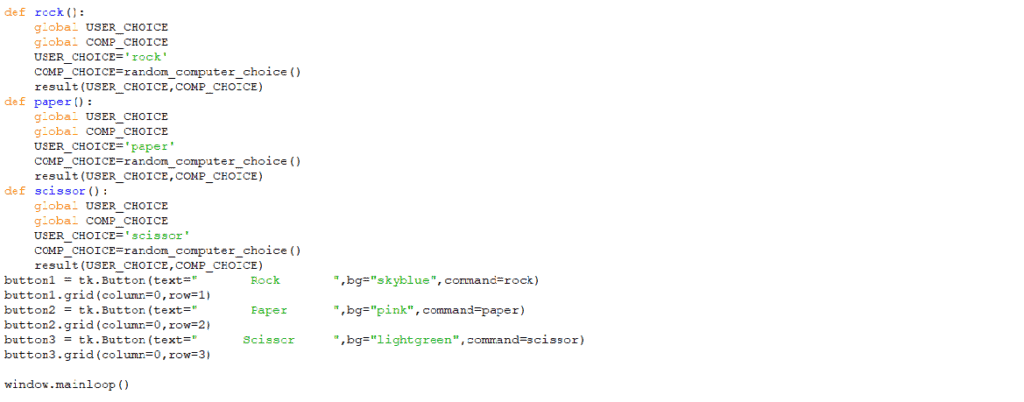
When you run the code, the graphical user interface(GUI) of the game looks like this.
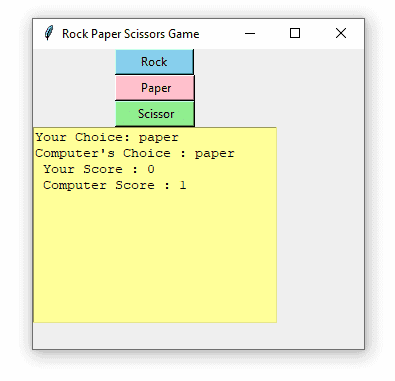
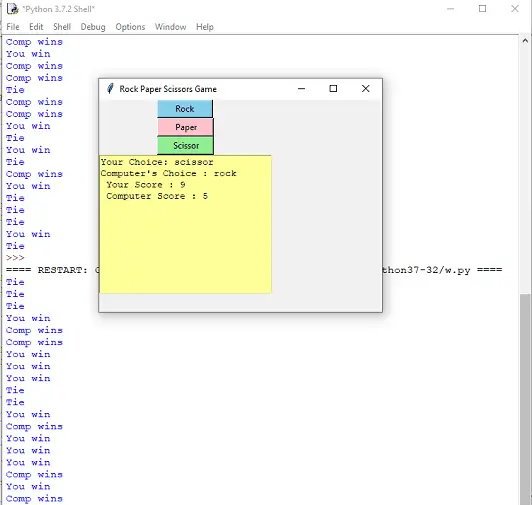
That’s it. Now, play and enjoy the game. Also, show your game to your friends and loved ones.
Convert the Game to an EXE File
We completed a cool project, a game that took us pretty little effort to make.
We can convert the code that we have written into clickable software. It will make our life extremely easy. We can run the game without going to the command line or IDLE.
If the program is like an application that runs on one or two clicks, it would have been pretty cool, right?. Let’s see how we can do this thing and build some software or application with our python code.
py2exe for Python
There is a software called py2exe for this purpose. You can download the software, and it will make your life easy. But, for all the geeky programmers out there, who want to do it by themselves, without using any third-party software, I will show you how to do it.
Converting .py to .exe without using py2exe
For that, we need to install the Pyinstaller library. If it does not exist in your system, go to the PowerShell or CMD and type in the following command. If you’re using Mac, use “pip3” instead of “pip”.
pip install pyinstaller
Once we have done that, go to the directory of the Python code we have written, using the cd command. After that, type in the following:
pyinstaller -F -w file_name.py
Use the name of your file instead of file_name.py in the above command. This command will automatically create some folders, and we will have an executable file inside the ‘dist’ folder.
Add an Icon to the Python Software
We can add an icon to our app to make it look much more real. For that, you should have an icon, with a .ico extension, ready to use. You can download a good-looking icon by searching the Internet.
To add an icon to the executable file, type in the following command:
pyinstaller -F -w -i icon_name.ico file_name.py
That’s it. If you have followed these steps, your executable python app will be ready to rock and roll.
Conclusion
Congratulations on creating a cool Python app! I hope this tutorial was able to show you the ease of creating beautiful apps using the Python language.
I want you to take it to the next level. Now, you know that making apps in Python is easy. So, brainstorm some cool ideas, and turn them into reality with Python.
Remember this thing: If you can think of an idea, then you can code it! How cool is that?
You must realize that mastering Tkinter will not land you any job. No real-world companies create software using Tkinter.
After doing many projects, your confidence in programming would be much higher than it was before.
Now, it’s time to take action. You can now move on to more advanced fields like machine learning, web development, etc. I hope you will do so.
There are lots of resources and books out there to learn Python programming, and it is often overwhelming for beginners. It is crucial to get the right resources to increase your knowledge, and not waste your time.
I have done some research on the best resources for programmers, and you can check out my resources page to find them.
If you have any doubts, queries, or bugs, feel free to comment down below. I will be happy to respond.
I would appreciate it if you would be willing to share this tutorial. It will encourage me to create more useful stuff like this.
i have some issues
Hi,
What are the issues?
Sir I have used your code but im my case its working but not quite showing the result
1)Whenever I press any button rock ,paper or scissor …result always shows tie .
2)And the computer score or user score doesn’t increment it remains 0
I just checked the code again, and I couldn’t see any issues. I’ve added an image above in the article, where I beat the computer 9-5 just now.
So, I think you might have accidentally made some errors in your code. Could you check the code again? Avoid all the indentation errors if any.
it works fine for me and using windows 7 i already converted to exe in windows , is very neat now i`m into apache to run it in android . Thank you very much
Cool
When i select scissors, i keep getting errors, only for the scissors button
hello, how do i get a image to appear each time i choose and how do i use a radio button instead of what is there?
Sir can we add image of scissors and rock and paper in the output window to make it more attractive
Yes, you can add images to make it cool. Check out the last part of this tutorial for reference: http://pythonistaplanet.com/age-calculator-app-using-python-tkinter/
In line number 25, you can use (user-comp==-1) instead of ((user-comp)%3==1)
How can I make that the game is played for only 10 rounds and that the winner is printed afterwards?
You can use a variable to count the number of plays. Then, add some conditional statements in the code to check the number of plays. When it reaches 10, you can compare the user score and computer score, and the one with the larger value will win.
I wanted to show the result (comp. win / user win / tie) above the Your score in Tkinter GUI. Then what changes to be done in program .
Add a new element to display text and change the grid accordingly.
I can’t understand the fundamental of this condition.
please explain it.
elif((user-comp)%3==1):
print(“You win”)
USER_SCORE+=1
In the choice_to_number() method, each choice is given a score associated with it (0, 1, and 2). Take a few possible conditions and put the corresponding values in (user-comp)%3==1 condition. You’ll understand the logic.
Error in printing values of user choice,comp scores — formatted print lines gives me a syntax error
I don’t see any errors in the code. It’s working fine. Debug the error and solve it. After all, programming is all about problem-solving.
Hi!
How will the following statement change if we change the numbering of elements in the dictionaries? Like if we use numbers 1, 2 & 3 instead of 0, 1, 2.
elif((user-comp)%3==1)
elif((user-comp)%3==1):
print(“You win”)
i didnt understood why you used it.how the user will win if elif((user-comp)%3==1).please make it clear
[0, 1, 2]
[r, p, s]
Situation 1
————
rock vs scissors
(rock – scissors) % 3 == 1
(0 – 2) % 3 == 1
(-2) % 3 == 1
1 == 1
rock wins (user)
Situation 2
——————
paper vs scissors
(paper – scissors) % 3 == 1
(1 – 2) % 3 == 1
2 == 1
scissor wins (computer, since the condition is not met)
Situation 3
————
paper vs rocks
(paper – rocks) % 3 == 1
(1 – 0) % 3 == 1
1 % 3 == 1
1 == 1
paper wins (user)
You can check the other situations similarly.
Hi, I am new to programming. Also I am not that expert in mathematics. I just want to ask you, something I can’t understand due to me being not good in math.
In this part here:
(1 – 2) % 3 == 1
2 == 1
When we subtract the first line, it would give ‘-1’ right? and we modulus it, the remainder would be a repeated threes right? -0.33333….
How did it became 2 after we modulus it? Did we round it? I know how to round but I don’t how it was done here.
I hope you can reply to my silly question, I really want know about this, also I can improve my math more 🙂
If you consider (-1) % 3 as ((-1)*3 + 2) = 1, you could see that 2 is the remainder here.
I would recommend you check out this article for more clarity on performing the modulo operation on negative numbers:
https://www.geeksforgeeks.org/how-to-perform-modulo-with-negative-values-in-python/
Eyyy thanks for the quick reply and for the recommendation man. You’re the best !
Cheers man. Glad that I could help.
What is the reason for the defining the function, number_choice()? The program works perfectly fine without the function
You can remove that from your code
after writing this step- pyinstaller -F -w file_name.py
i am getting a error like-‘pyinstaller’ is not recognized as an internal or external command,
operable program or batch file.
please help me out of this.
Maybe Pyinstaller wasn’t properly installed on your system. Try installing it again. If pip doesn’t work, try easy_install Pyinstaller.
well, if you have a mac, “pip” doesn’t work, then you have to use “pip3”
Yeah. That’s right. Thanks Juan.
This is an amazing project thank you for sharing it!
Thank you for sharing this wonderful learning opportunity.
Glad it helped.
i am getting syntax error at elif statement. could you please tell me how to solve this