In Python programming, tuples are immutable ordered sequences of elements, similar to lists but with the key difference that they cannot be changed once defined. Tuples are immutable, whereas lists are mutable.
A tuple is defined using parentheses () with elements separated by commas. Tuples are an important data structure in Python, and we can use them in various scenarios.
In this post, I’ve put together some simple examples and exercises for using tuples in Python for various tasks. Check out these examples to get a clear idea of how tuples work in Python.
Let’s dive right in.
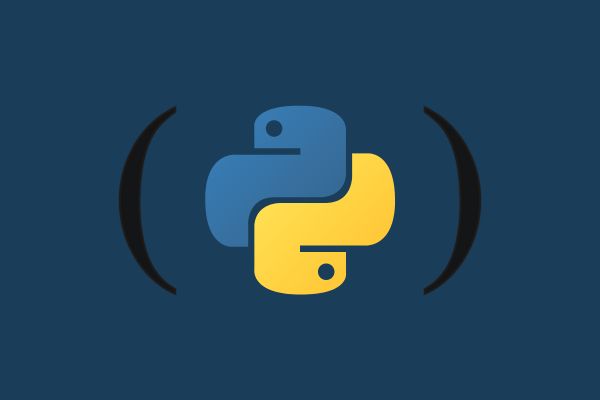
1. Example of Creating a Tuple in Python
myTuple = (1, 2, 3, 4, 5)
print("Tuple elements:", myTuple)
Output:

2. Example of Accessing Elements in a Tuple
myTuple = (10, 20, 30, 40, 50)
print("First element:", myTuple[0])
print("Third element:", myTuple[2])
Output:
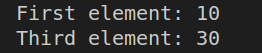
3. Example of Tuple Slicing
myTuple = (1, 2, 3, 4, 5, 6, 7, 8, 9)
slicedTuple = myTuple[1:6]
print("Sliced tuple:", slicedTuple)
Output:

4. Example of Concatenating Tuples
tuple1 = (1, 2, 3)
tuple2 = (4, 5, 6)
concatenatedTuple = tuple1 + tuple2
print("Concatenated tuple:", concatenatedTuple)
Output:

5. Example of Repeating Tuples
myTuple = (1, 2, 3)
repeatedTuple = myTuple = (1, 2, 3) * 3
print("Repeated tuple:", repeatedTuple)
Output:

6. Example of Finding the Length of a Tuple
myTuple = (1, 2, 3, 4, 5)
tupleLength = len(myTuple)
print("Length of tuple:", tupleLength)
Output:

7. Example of Counting the Occurrences of an Element in a Tuple
myTuple = (1, 2, 3, 1, 1, 4, 5, 1)
count = myTuple.count(1)
print("Number of occurrences of 1:", count)
Output:

8. Example of Finding the Index of an Element in a Tuple
myTuple = (10, 20, 30, 40, 50)
index = myTuple.index(30)
print("Index of element 30:", index)
Output:

9. Example of Nested Tuples
nestedTuple = (1, (2, 3), 4, (5, (6, 7)))
print("Nested tuple:", nestedTuple)
Output:

10. Example of Unpacking a Tuple
myTuple = (1, 2, 3, 4, 5)
a, b, c, d, e = myTuple
print("Unpacked tuple values:", a, b, c, d, e)
Output:

11. Example of Swapping Variables Using Tuples
x = 10
y = 20
x, y = y, x
print("Swapped variables:", x, y)
Output:

12. Example of Converting a List to a Tuple
myList = [1, 2, 3, 4, 5]
myTuple = tuple(myList)
print("Converted tuple:", myTuple)
Output:

I hope this post was helpful. Check out my tutorial on 23 Python List Exercises and Examples.
Quite interesting. I never knew you could unpack a tuple like that.
Try to add code in terms of converting a tuple to List and performing all such operations on converted list so far.