In Python, a list is a data structure that stores a list of items in an arranged order. We can create a list by using square brackets with items inside and use commas to separate each item.
In this post, I have compiled some examples of using lists in Python. I hope these examples would help you understand the working of lists in Python. Let’s dive right in.
1. Python list of strings
groceryList = ["Rice","Wheat","Barley"]
print(groceryList)

2. Python list of numbers
myNumbers = [1,5,9,16,28]
print(myNumbers)
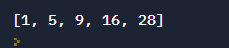
3. Python list with duplicate values
myNumbers = [1,5,5,9,9,9,16,28]
print(myNumbers)

4. Python list that contains different data type values
heterogeneousList = ["Python",123,24.5,True,35,"a"]
print(heterogeneousList)

5. Finding the length of a list in Python
ProgrammingList = ["CSharp","Python","PHP","R"]
print(len(ProgrammingList))
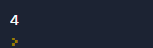
6. Finding the index of items in a Python list
fruits = ["apple","banana","mango"]
bananaIndex = fruits.index("banana")
mangoIndex = fruits.index("mango")
print(bananaIndex)
print(mangoIndex)
pythonList = ["p","y","t","h","o","n"]
# to get the 1st item
print(pythonList[0])
#to get the 4th item
print(pythonList[3])
# Negative indexing to get last item
print(pythonList[-1])
# To get second last item
print(pythonList[-2])
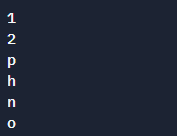
7. Slicing of lists in Python
myList = ['p','y','t','h','o','n','i','s','t','a']
# elements from 5 to 8
print(myList[4:8])
# from 1st element to 4th element
print(myList[:4])
# from 8th element to last element
print(myList[7:])
# from 1st to last elements
print(myList[:])
# negative slicing
print(myList[-1:-9])
print(myList[-5:])
print(myList[:-4])
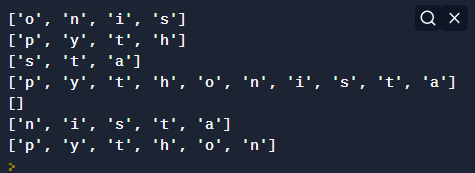
8. Adding items to a list using insert() and append() in Python
If we use the append method to add an item to a list, the new item will be added at the end of the list.
numbers = [10,20,30,40,50,60]
numbers.append(70)
print(numbers)

If we use the insert method to add items to a list, we have to specify the position at which we want to insert an item. The insert() method takes two arguments [ insert(position,item) ].
numbers = [10,20,30,40,50,60]
numbers.insert(0,5)
print(numbers)
9. Merging two lists using extend method in Python
list1 = ["Apple","Orange","Mango"]
list2 = ["Grape","Strawberry"]
list1.extend(list2)
print(list1)

10. Removing items from a list using pop() in Python
x = [23,45,67,89,34,12]
# removing the last element
x.pop()
print(x)
# removing the 0th index element
x.pop(0)
print(x)
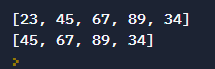
11. Removing items from a list using remove() in Python
dryFruits = ["Cashew nuts","Dates","Almonds","Raisins"]
dryFruits.remove("Raisins")
print(dryFruits)

12. Remove all the elements from a list in Python
dryFruits = ["Cashew nuts","Dates","Almonds","Raisins"]
dryFruits.clear()
print(dryFruits)
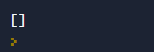
13. Creating a copy of a list in Python
dryFruits = ["Cashew nuts","Dates","Almonds","Raisins"]
d = dryFruits.copy()
print(d)

14. Finding the number of times an item is present in a list
myList = [1,2,2,3,3,3,4,4,4,4]
count = myList.count(4)
print(count)
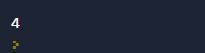
15. Reversing a list in Python
myList = ["Pen","Pencil","Book"]
myList.reverse()
print(myList)

16. Sorting a list in Python
myList = ["Pen","Pencil","Book","Eraser"]
myList.sort()
print(myList)

17. Deleting items in a list using del keyword
colorList = ["Red","Blue","Green","Yellow","Orange","Purple"]
# deleting an item
del colorList[2]
print(colorList)
# deleting multiple items
del colorList[1:3]
print(colorList)
# deleting the entire list
del colorList

18. Nested list in Python
nestedList = ["Apple",["Ball",1,2.5],['a',128]]
print(nestedList)
print(nestedList[0])
print(nestedList[1])
print(nestedList[2])
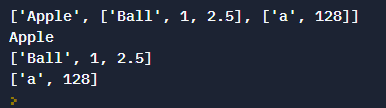
19. Creating a list using the list() constructor
myList = list(("Mac","Windows","Linux"))
print(myList)

20. Checking whether an item exists in a list or not
myList = list(("Mac","Windows","Linux"))
if "Mac" in myList:
print("Yes")
else:
print("No")
if "Linux" not in myList:
print("Yes")
else:
print("No")
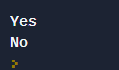
21. Concatenating lists in Python
myList1 = ["Mac","Windows","Linux"]
myList2 = ["Book","Pencil","Pen"]
combinedList = myList1 + myList2
print(combinedList)

22. Repeating a list in Python
myList1 = ["Mac","Windows"]
combinedList = myList1 * 3
print(combinedList)

23. Python list of numbers of the power of 2
myList = [2**x for x in range(10)]
print(myList)

Let me warmly congratulate you for your initiative in sharing the useful and nice python features!
Thank you. Appreciate it!