In Python programming, a set is an unordered collection of unique elements. Sets are mutable, but the elements inside a set must be immutable and hashable. We use sets for operations like membership testing, deduplication, and set-based mathematical operations like union, intersection, and difference.
In this post, I’ve put together some simple examples and exercises for using sets in Python for various needs. Check out these examples to get a clear idea of how sets work in Python.
Let’s dive right in.
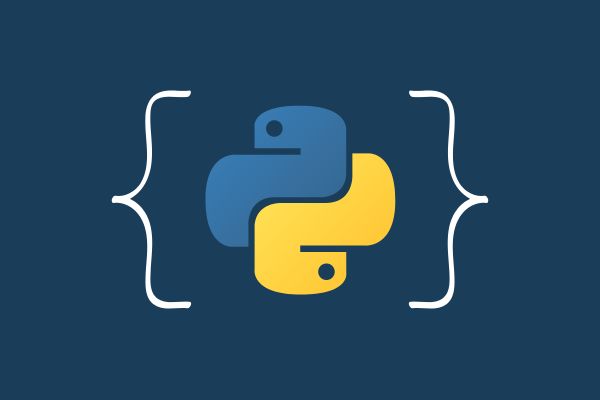
1. Creating a set in Python
mySet = {1, 2, 3, 4, 5}
print("Set elements:", mySet)
Output:

2. Adding an element to a set
mySet = {1, 2, 3}
mySet.add(4)
print("Set after adding an element:", mySet)
Output:

3. Removing an element from a set
mySet = {1, 2, 3, 4}
mySet.remove(3)
print("Set after removing an element:", mySet)
Output:

4. Union of two sets
setOne = {1, 2, 3}
setTwo = {3, 4, 5}
unionSet = setOne.union(setTwo)
print("Union of two sets:", unionSet)
Output:

5. Intersection of two sets
setOne = {1, 2, 3}
setTwo = {3, 4, 5}
intersectionSet = setOne.intersection(setTwo)
print("Intersection of two sets:", intersectionSet)
Output:

6. Difference between two sets
setOne = {1, 2, 3}
setTwo = {3, 4, 5}
differenceSet = setOne.difference(setTwo)
print("Difference between two sets:", differenceSet)
Output:

7. Checking if an element is a member of a set
mySet = {1, 2, 3, 4, 5}
print("Is 3 a member of the set?", 3 in mySet)
Output:

8. Converting a list to a set
myList = [1, 2, 3, 4, 4, 5, 5]
mySet = set(myList)
print("Converted set:", mySet)
Output:

9. Iterating over a set
mySet = {1, 2, 3, 4, 5}
for element in mySet:
print("Element:", element)
Output:
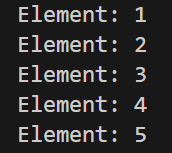
10. Finding the length of a set
mySet = {1, 2, 3, 4, 5}
setLength = len(mySet)
print("Length of set:", setLength)
Output:

I hope this article was helpful. Check out my post on 12 Python Tuple Exercises and Examples.
Happy coding!