Regular expressions are a powerful tool for working with text data in Python. A regular expression is a sequence of characters that define a search pattern, which can be used for pattern-matching, replacing, or extracting specific information from a text. The re
module of Python provides support for regular expressions.
In this article, I have added some simple examples and exercises to demonstrate the use of regular expressions in Python. Check out these examples to get a clear idea of how regular expressions work.
Let’s jump right in.
1. Importing the re module
import re
2. Basic pattern matching using search()
import re
pattern = "Python"
text = "I write code in Python."
match = re.search(pattern, text)
print("Match found:", bool(match))
Output:

3. Using findall() to find all occurrences of a pattern
import re
pattern = "a"
text = "I love to code in Python and it's amazing!"
matches = re.findall(pattern, text)
print("Matches found:", len(matches))
Output:

4. Using split() to split a string based on a pattern
import re
pattern = "\s"
text = "I like Python and it is amazing!"
split_text = re.split(pattern, text)
print("Split text:", split_text)
Output:

5. Using sub() to replace a pattern with a string
import re
pattern = "Python"
replacement = "Java"
text = "Python is fun"
substituted_text = re.sub(pattern, replacement, text)
print("Substituted text:", substituted_text)
Output:

6. Using ^ to match the start of a string
import re
pattern = "^I"
text = "I love Python!"
match = re.search(pattern, text)
print("Match found:", bool(match))
Output:

7. Using $ to match the end of a string
import re
pattern = "Python!$"
text = "Python programming is great!"
match = re.search(pattern, text)
print("Match found:", bool(match))
Output:

8. Using [] to create a character set
import re
pattern = "[Pp]ython"
text = "I write code in python and Python!"
matches = re.findall(pattern, text)
print("Matches found:", len(matches))
Output:

9. Using . to match any character
import re
pattern = "Py...n"
text = "I love Python, Pyt3on, Py45n, and Py@#n!"
matches = re.findall(pattern, text)
print("Matches found:", len(matches))
Output:

10. Using * to match zero or more occurrences of a character
import re
pattern = "Py.*n"
count = 0
text = ["Python coding", "Pyt3on","Java", "Py45n", "Py@#n","Pyn"]
for i in text:
if(re.findall(pattern, i)):
count+=1
print("Matches found:", count)
Output:

11. Using + to match one or more occurrences of a character
import re
pattern = "Py.+n"
count = 0
text = ["Python coding", "Pyt3on","Java", "Py45n", "Py@#n","Pyn"]
for i in text:
if(re.findall(pattern, i)):
count+=1
print("Matches found:", count)

12. Using ? to match zero or one occurrence of a character
import re
pattern = "Py.?n"
count = 0
text = ["Python coding", "Pyt3on","Java", "Py45n", "Py@#n","Pyn"]
for i in text:
if(re.findall(pattern, i)):
count+=1
print("Matches found:", count)
Output:

13. Using {} to specify the number of occurrences of a character
import re
pattern = "Py{1,2}n"
text = "I love Pyn, Pyyn, and Pyyyn!"
matches = re.findall(pattern, text)
print("Matches found:", len(matches))
Output:

14. Using \d to match digits
import re
pattern = "\d+"
text = "My phone number is 123-456-7890."
matches = re.findall(pattern, text)
print(matches)
print("Matches found:", len(matches))
Output:

15. Using \w to match alphanumeric characters
import re
pattern = "\w+"
text = "I_love_Python!"
matches = re.findall(pattern, text)
print(matches)
print("Matches found:", len(matches))
Output:
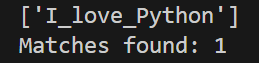
16. Using \s to match whitespace characters
import re
pattern = "\s+"
text = "I love Python!"
matches = re.findall(pattern, text)
print("Matches found:", len(matches))
Output:

17. Using | as an OR operator
import re
pattern = "Python|Java"
text = "I write code in Python and Java!"
matches = re.findall(pattern, text)
print("Matches found:", len(matches))
Output:

18. Using () to group patterns
import re
pattern = "(\d{3})-(\d{3})-(\d{4})"
text = "My phone number is 123-456-7890."
match = re.search(pattern, text)
if match:
print("Area code:", match.group(1))
print("Local exchange:", match.group(2))
print("Line number:", match.group(3))
Output:
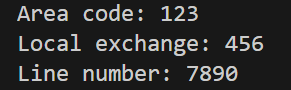
I hope this article was helpful. Check out my post on 15 Python String Manipulation Exercises.