The file I/O (input/output) involves the process of reading data from a file or writing data to a file. Python provides several in-built methods necessary to manipulate files. You can do most of the file manipulation using a file object.
In this post, I will illustrate some exercises and examples demonstrating file I/O operations in Python. Let’s dive right in.
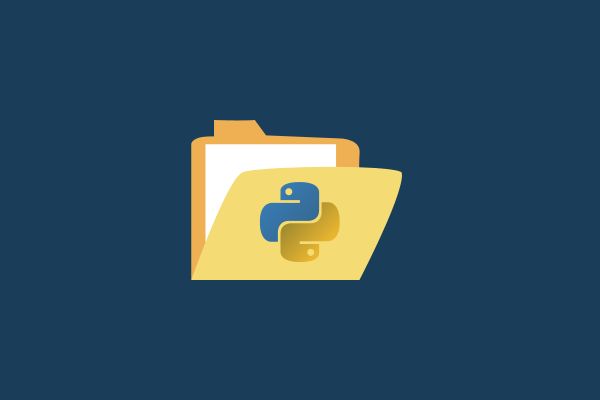
1. Basic file opening and reading
Let’s create a file called “example.txt” with some text in it. Save the file in the same folder as that of the Python file. We will try to read this text file using Python.
try:
file = open('example.txt', 'r')
content = file.read()
print(content)
finally:
file.close()
Output:

2. Using with statement for file handling
with open('example.txt', 'r') as file:
print(file.read())
Output:

3. Writing to a file
with open('example.txt', 'w') as file:
file.write("Hello, Pythonista!")
Output:
The file content has changed to “Hello, Pythonista!”.
4. Reading a specific number of characters
with open('example.txt', 'r') as file:
print(file.read(5))
Output:
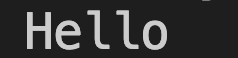
5. Reading one line at a time using readline
with open('example.txt', 'r') as file:
print(file.readline())
Output:

6. Reading all lines at once using readlines
with open('example.txt', 'r') as file:
print(file.readlines())
Output:

7. Looping over a file object
with open('example.txt', 'r') as file:
for line in file:
print(line, end='')
Output:

8. Writing multiple lines to a file
lines = ["Hello, Pythonista!\n", "Welcome to the world of Python.\n"]
with open('example.txt', 'w') as file:
file.writelines(lines)
Output:
The file content changed to the given 2 lines.
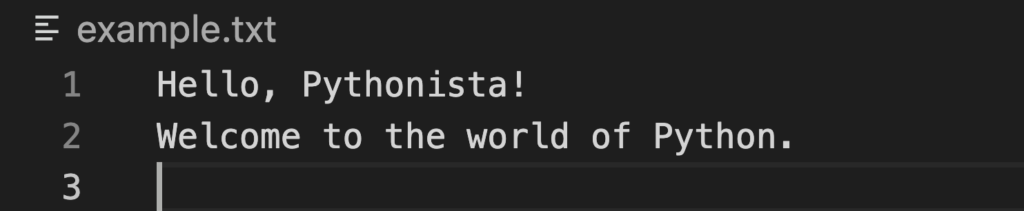
9. Appending to a file
with open('example.txt', 'a') as file:
file.write("\nKeep on learning!")
Output:
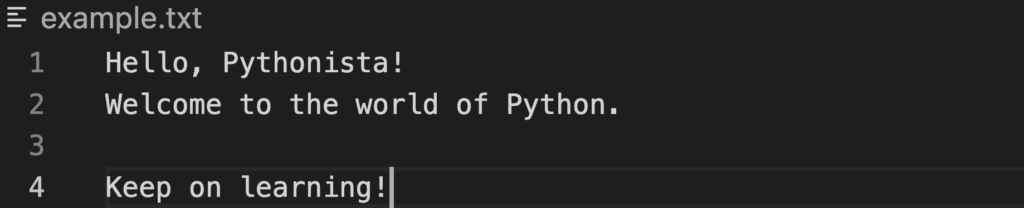
10. Using tell to get the current file position
with open('example.txt', 'r') as file:
file.read(10)
print(file.tell())
Output:

11. Using seek to change the file position
with open('example.txt', 'r') as file:
file.seek(15)
print(file.read())
Output:
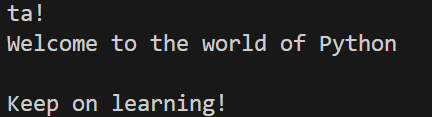
12. Checking if a file exists
import os
print(os.path.isfile('example.txt'))
Output:
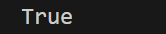
13. Getting the size of a file
import os
print(os.path.getsize('example.txt'))
Output:

14. Renaming a file
import os
os.rename('example.txt', 'new_example.txt')
Output:
The file is renamed.

15. Removing a file
import os
os.remove('new_example.txt')
Output:
File deleted.
16. Creating a directory
import os
os.mkdir('newDirectory')
Output:
A new folder was created.
17. Changing the current working directory
import os
os.chdir('newDirectory')
18. Getting the current working directory
import os
os.chdir('newDirectory')
print(os.getcwd())
Output:
The new folder path is displayed.
19. Removing a directory
import os
os.rmdir('newDirectory')
Output:
The newDirectory folder got deleted.
20. Listing all files and directories
import os
print(os.listdir())
Output:
Prints the file names of all files in the current working directory.
I hope this article was helpful in understanding file I/O operations in Python. It’s a fundamental part of Python programming and is crucial when dealing with data analysis or any tasks that involve file manipulation.
Check out my other post on 15 Python Exception Handling Exercises and Examples.