In Python programming, exceptions are events that occur during the execution of programs that disrupt the normal flow of instructions of the program. When an exceptional condition arises, Python raises an exception. If not handled, the program will crash. To prevent this, the exceptions are caught and handled using try, except, else, finally, and raise keywords.
In this post, I’ve put together some simple examples and exercises for handling exceptions in Python. Check out these examples to get a clear idea of how exception handling works in Python.
Let’s dive right in.
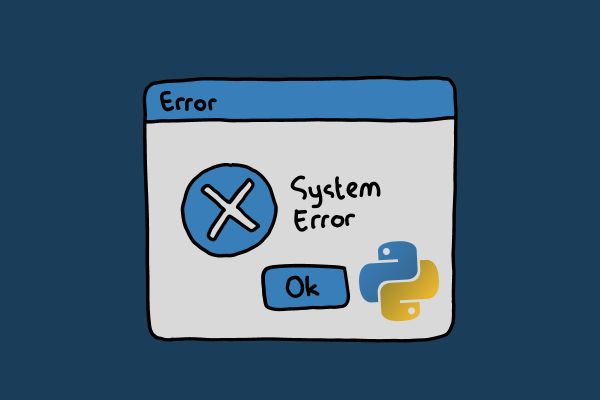
1. Basic try and except block
try:
print(undefined_variable)
except NameError:
print("This variable is not defined.")
Output:

2. Handling multiple exceptions
try:
result = 10 / 0
except (ZeroDivisionError, TypeError) as e:
print("Error occurred:", str(e))
Output:

3. Using the else clause in exception handling
try:
result = 10 / 2
except ZeroDivisionError:
print("Division by zero!")
else:
print("Result is", result)
Output:

4. Using the finally clause in exception handling
try:
result = 10 / 0
except ZeroDivisionError:
print("Division by zero!")
finally:
print("This block of code will always execute.")
Output:

5. Raising exceptions using raise
try:
raise ValueError("This is a custom error message.")
except ValueError as e:
print("An error occurred:", str(e))
Output:

6. Creating custom exceptions
class CustomError(Exception):
pass
try:
raise CustomError("This is a custom error.")
except CustomError as e:
print("An error occurred:", str(e))
Output:

7. Catching all exceptions using Exception
try:
result = 10 / 0
except Exception as e:
print("An error occurred:", str(e))
try:
print(undefined_variable)
except Exception as e:
print("An error occurred:", str(e))
Output:

8. Using assert for exception handling
try:
x = -1
assert x >= 0, "Only positive values are allowed."
except AssertionError as e:
print("An error occurred:", str(e))
Output:

9. Handling exceptions within functions
def divide(a, b):
try:
return a / b
except ZeroDivisionError:
print("Division by zero!")
return None
print(divide(10, 0))
Output:
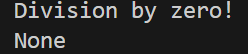
10. Using a while loop for handling exceptions
while True:
try:
x = int(input("Please enter a number: "))
break
except ValueError:
print("Oops! That was no valid number. Try again…")
Output:

11. Reraising exceptions
try:
result = 10 / 0
except ZeroDivisionError:
print("Caught an exception")
raise
Output:

12. Using else clause with try/except inside a function
def divide(a, b):
try:
result = a / b
except ZeroDivisionError:
print("Division by zero!")
return None
else:
print("Division successful!")
return result
print(divide(10, 2))
Output:

13. Handling exceptions with finally clause in file handling
try:
f = open('myfile.txt', 'r')
content = f.read()
f.close()
except IOError:
print('File not found.')
Output:

14. Ignoring exceptions
try:
result = 10 / 0
except ZeroDivisionError:
pass
Output: Prints nothing
15. Propagating exceptions
def sqrt(x):
if x < 0:
raise ValueError("Cannot compute square root of a negative number.")
else:
return x ** 0.5
try:
print(sqrt(-10))
except ValueError as e:
print("An error occurred:", str(e))
Output:

I hope this article helped you in understanding how exception handling works in Python. Always remember that proper exception handling makes your programs robust and easier to debug.
Check out my post on 18 Python Regular Expressions Exercises and Examples. Happy coding!