If you have learned the basics of Java, it is the right time to solve some practice problems. Practicing and solving problems will help you master the Java programming language and take your skills to the next level.
In this post, I have put together some Java coding problems that you can use for practice. I have also provided the Java code solutions and the corresponding output for your reference.
Try to solve these problems by yourself and get better at Java. Let’s dive right in.
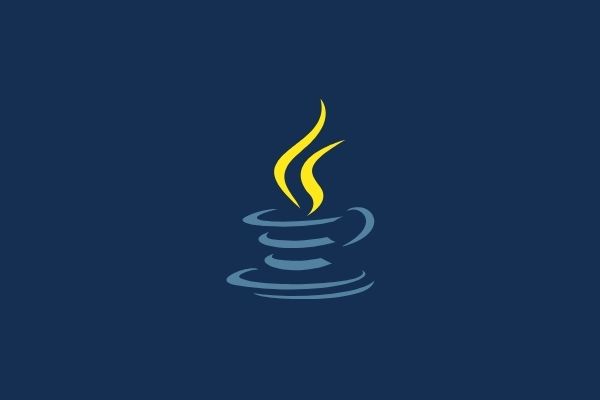
1. Java program to check whether the given number is even or odd
import java.util.Scanner;
class Main {
public static void main(String[] args) {
System.out.println("Enter a number: ");
Scanner sc = new Scanner(System.in);
int number =Integer.parseInt(sc.nextLine());
int x = number%2;
if(x==0){
System.out.println("The number is Even");
}
else{
System.out.println("The number is Odd");
}
}
}
Output:
Enter a number:
24
The number is Even
Enter a number:
33
The number is Odd
2. Java program to convert the temperature in Centigrade to Fahrenheit
import java.util.Scanner;
class Main {
public static void main(String[] args) {
System.out.println("Enter temperature in Centigrade: ");
Scanner sc = new Scanner(System.in);
int c =Integer.parseInt(sc.nextLine());
float f = ((9f*c)/5f)+32;
System.out.println("Temperature in Fahrenheit is: "+f);
}
}
Output:
Enter temperature in Centigrade:
43
Temperature in Fahrenheit is: 109.4
3. Java program to find the area of a triangle whose three sides are given
import java.util.Scanner;
class Main {
public static void main(String[] args) {
Scanner sc= new Scanner(System.in);
System.out.println("Enter the 1st side:");
int a= sc.nextInt();
System.out.println("Enter the 2nd side:");
int b= sc.nextInt();
System.out.println("Enter the 3rd side:");
int c= sc.nextInt();
if((a+b)>c && (a+c)>b && (b+c)>a)
{
double s=(a+b+c)/2.0;
double area=Math.sqrt(s*(s-a)*(s-b)*(s-c));
System.out.println("Area of Triangle is: " + area);
}
else
System.out.println("Area of the triangle does not exist");
}
}
Output:
Enter the 1st side:
4
Enter the 2nd side:
3
Enter the 3rd side:
6
Area of Triangle is: 5.332682251925386
4. Java program to find out the average of a set of integers
import java.util.Scanner;
class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter the count of numbers: ");
int count = Integer.parseInt(sc.nextLine());
int i = 0;
float sum = 0;
for(i=0;i<count;i++){
System.out.println("Enter an integer: ");
int x = Integer.parseInt(sc.nextLine());
sum = sum + x;
}
float avg = sum/count;
System.out.println("The average is: "+avg);
}
}
Output:
Enter the count of numbers:
5
Enter an integer:
3
Enter an integer:
8
Enter an integer:
6
Enter an integer:
7
Enter an integer:
2
The average is: 5.2
5. Java program to find the product of a set of real numbers
import java.util.Scanner;
class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter the number of real numbers: ");
int count = Integer.parseInt(sc.nextLine());
int i = 0;
float product = 1.0f;
for(i=0;i<count;i++){
System.out.println("Enter a real number: ");
float x = Float.parseFloat(sc.nextLine());
product = product * x;
}
System.out.println("The product of the numbers is: "+product);
}
}
Output:
Enter the number of real numbers:
4
Enter a real number:
3.2
Enter a real number:
4.4
Enter a real number:
5.1
Enter a real number:
3.8
The product of the numbers is: 272.87042
6. Java program to find the circumference and area of a circle with a given radius
import java.util.Scanner;
class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Input the radius of the circle: ");
double radius = Float.parseFloat(sc.nextLine());
double c = 2 * Math.PI * radius;
double area = Math.PI * radius * radius;
System.out.println("The circumference of the circle is: "+c);
System.out.println("The area of the circle is: "+area);
}
}
Output:
Input the radius of the circle:
4.3
The circumference of the circle is: 27.017698019294713
The area of the circle is: 58.0880533180921
7. Java program to check whether the given integer is a multiple of 5
import java.util.Scanner;
class Main {
public static void main(String[] args) {
System.out.println("Enter an integer: ");
Scanner sc = new Scanner(System.in);
int number =Integer.parseInt(sc.nextLine());
if(number%5==0){
System.out.println(number+" is a multiple of 5");
}
else{
System.out.println(number+" is not a multiple of 5");
}
}
}
Output:
Enter an integer:
55
55 is a multiple of 5
Enter an integer:
23
23 is not a multiple of 5
8. Java program to check whether the given integer is a multiple of both 5 and 7
import java.util.Scanner;
class Main {
public static void main(String[] args) {
System.out.println("Enter an integer: ");
Scanner sc = new Scanner(System.in);
int number =Integer.parseInt(sc.nextLine());
if((number%5==0)&&(number%7==0)){
System.out.println(number+" is a multiple of both 5 and 7");
}
else{
System.out.println(number+" is not a multiple of both 5 and 7");
}
}
}
Output:
Enter an integer:
33
33 is not a multiple of both 5 and 7
Enter an integer:
70
70 is a multiple of both 5 and 7
9. Java program to find the average of 5 numbers using a while loop
import java.util.Scanner;
class Main {
public static void main(String[] args) {
int count = 0;
int sum = 0;
Scanner sc = new Scanner(System.in);
while(count<5){
System.out.println("Enter an integer: ");
int number =Integer.parseInt(sc.nextLine());
sum = sum+number;
count++;
}
double avg = ((double) sum) / 5.0;
System.out.println("Average is: "+avg);
}
}
Output:
Enter an integer:
6
Enter an integer:
2
Enter an integer:
5
Enter an integer:
7
Enter an integer:
9
Average is: 5.8
10. Java program to display the given integer in the reverse order
import java.util.Scanner;
class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter an integer: ");
int number =Integer.parseInt(sc.nextLine());
int rev = 0;
while(number!=0){
int digit = number%10;
rev = (rev*10)+digit;
number = number/10;
}
System.out.println(rev);
}
}
Output:
Enter an integer:
547
745
11. Java program to find the geometric mean of n numbers
import java.util.Scanner;
class Main {
public static void main(String[] args) {
int c = 0;
double p = 1.0;
Scanner sc = new Scanner(System.in);
System.out.println("Enter the number of values: ");
int count =Integer.parseInt(sc.nextLine());
while((c<count)){
System.out.println("Enter a real number: ");
double x =Double.parseDouble(sc.nextLine());
c = c+1;
p = p * x;
}
double gm = Math.pow(p,1.0/count);
System.out.println("The geometric mean is: "+gm);
}
}
Output:
Enter the number of values:
5
Enter a real number:
4.31
Enter a real number:
3.98
Enter a real number:
1.90
Enter a real number:
4.42
Enter a real number:
9.19
The geometric mean is: 4.210855561928519
12. Java program to find the sum of the digits of an integer using a while loop
import java.util.Scanner;
class Main {
public static void main(String[] args) {
int sum = 0;
Scanner sc = new Scanner(System.in);
System.out.println("Enter an integer: ");
int number =Integer.parseInt(sc.nextLine());
while(number!=0){
int digit = number%10;
sum = sum+digit;
number = number/10;
}
System.out.println(("Sum of digits is: "+ sum));
}
}
Output:
Enter an integer:
357
Sum of digits is: 15
13. Java program to display all the multiples of 3 within the range 10 to 50
import java.util.Scanner;
class Main {
public static void main(String[] args) {
for(int i=10;i<50;i++){
if(i%3==0)
System.out.println(i);
}
}
}
Output:
12
15
18
21
24
27
30
33
36
39
42
45
48
14. Java program to display all integers within the range 100-150 whose sum of digits is an even number
import java.util.Scanner;
class Main {
public static void main(String[] args) {
for(int i=100;i<150;i++){
int num = i;
int sum = 0;
while(num!=0){
int digit = num%10;
sum = sum + digit;
num = num/10;
}
if(sum%2==0){
System.out.println(i);
}
}
}
}
Output:
101
103
105
107
109
110
112
114
116
118
121
123
125
127
129
130
132
134
136
138
141
143
145
147
149
15. Java program to check whether the given integer is a prime number or not
import java.util.Scanner;
class Main {
public static void main(String[] args) {
System.out.println("Enter an integer greater than 1: ");
Scanner sc = new Scanner(System.in);
int number =Integer.parseInt(sc.nextLine());
int isprime = 1;
for(int i=2;i<(number/2);i++){
if(number%i==0){
isprime = 0;
break;
}
}
if(isprime==1){
System.out.println(number+" is a prime number");
}
else{
System.out.println(number+" is not a prime number");
}
}
}
Output:
Enter an integer greater than 1:
23
23 is a prime number
Enter an integer greater than 1:
38
38 is not a prime number
16. Java program to generate the prime numbers from 1 to N
import java.util.Scanner;
class Main {
public static void main(String[] args) {
System.out.println("Enter the range: ");
Scanner sc = new Scanner(System.in);
int number =Integer.parseInt(sc.nextLine());
for (int num = 2; num <= number; num++)
{
boolean isPrime = true;
for (int i=2; i <= num/2; i++)
{
if ( num % i == 0)
{
isPrime = false;
break;
}
}
if ( isPrime == true )
System.out.println(num);
}
}
}
Output:
Enter the range:
10
2
3
5
7
17. Java program to find the roots of a quadratic equation
import java.util.Scanner;
class Main {
public static void main(String[] args) {
System.out.println("Enter the first coefficient: ");
Scanner sc = new Scanner(System.in);
double a = Integer.parseInt(sc.nextLine());
System.out.println("Enter the second coefficient: ");
double b = Integer.parseInt(sc.nextLine());
System.out.println("Enter the third coefficient: ");
double c = Integer.parseInt(sc.nextLine());
if(a!=0.0){
double d = (b*b)-(4*a*c);
if (d==0.0){
System.out.println("The roots are real and equal.");
double r = -b/(2*a);
System.out.println("The roots are "+ r+"and"+ r);
}else if(d>0.0){
System.out.println("The roots are real and distinct.");
double r1 = (-b+(Math.sqrt(d)))/(2*a);
double r2 = (-b-(Math.sqrt(d)))/(2*a);
System.out.println("The root1 is: "+ r1);
System.out.println("The root2 is: "+ r2);
}else{
System.out.println("The roots are imaginary.");
double rp = -b/(2*a);
double ip = Math.sqrt(-d)/(2*a);
System.out.println("The root1 is: "+ rp+ "+ i"+ip);
System.out.println("The root2 is: "+ rp+ "- i"+ip);
}
}else{
System.out.println("Not a quadratic equation.");
}
}
}
Output:
Enter the first coefficient:
4
Enter the second coefficient:
7
Enter the third coefficient:
2
The roots are real and distinct.
The root1 is: -0.3596117967977924
The root2 is: -1.3903882032022077
18. Java program to print the numbers from a given number n till 0 using recursion
class Main {
public static void main(String[] args) {
print_till_zero(8);
}
public static void print_till_zero(int n){
if(n==0)
return;
System.out.println(n);
n=n-1;
print_till_zero(n);
}
}
Output:
8
7
6
5
4
3
2
1
19. Java program to find the factorial of a number using recursion
import java.util.Scanner;
class Main {
public static void main(String[] args) {
System.out.println("Enter an integer: ");
Scanner sc = new Scanner(System.in);
int a = Integer.parseInt(sc.nextLine());
int result = fact(a);
System.out.println("The factorial of"+ a + " is: "+ result);
}
public static int fact(int n){
int f;
if(n==1)
f=1;
else
f = n * fact(n-1);
return f;
}
}
Output:
Enter an integer:
7
The factorial of7 is: 5040
20. Java program to display the sum of n numbers using an array
import java.util.Scanner;
class Main {
public static void main(String[] args) {
System.out.println("How many numbers: ");
Scanner sc = new Scanner(System.in);
int num = Integer.parseInt(sc.nextLine());
int[] numbers = new int[num];
int sum=0;
for(int n=0;n<num;n++){
System.out.println("Enter number: ");
int x = Integer.parseInt(sc.nextLine());
numbers[n] = x;
}
for(int i = 0; i < numbers.length; i++) {
sum = sum + numbers[i];
}
System.out.println("Sum of all the elements of the array: " + sum);
}
}
Output:
How many numbers:
3
Enter number:
2
Enter number:
5
Enter number:
3
Sum of all the elements of the array: 10
21. Java program to implement linear search
import java.util.Scanner;
class Main {
public static void main(String[] args) {
int[] numbers = {4,2,7,1,8,3,6};
int flag = 0;
System.out.println("Enter the number to be found out: ");
Scanner sc = new Scanner(System.in);
int x = Integer.parseInt(sc.nextLine());
for(int i=0;i<numbers.length;i++){
if (x==numbers[i]){
System.out.println("Successful search, the element is found at position "+ i);
flag = 1;
break;
}
}
if(flag==0){
System.out.println("Oops! Search unsuccessful");
}
}
}
Output:
Enter the number to be found out:
7
Successful search, the element is found at position 2
Enter the number to be found out:
9
Oops! Search unsuccessful
22. Java program to implement binary search
import java.util.Scanner;
class Main {
public static void main(String[] args) {
int[] numbers = {1,4,6,7,12,17,25}; //binary search requires sorted numbers
System.out.println("Enter the number to be found out: ");
Scanner sc = new Scanner(System.in);
int x = Integer.parseInt(sc.nextLine());
int result = binarySearch(numbers, 0, numbers.length-1, x);
if (result != -1)
System.out.println("Search successful, element found at position "+result);
else
System.out.println("The given element is not present in the array");
}
public static int binarySearch(int[] numbers,int low,int high,int x){
if (high >= low){
int mid = low + (high - low)/2;
if (numbers[mid] == x)
return mid;
else if (numbers[mid] > x)
return binarySearch(numbers, low, mid-1, x);
else
return binarySearch(numbers, mid+1, high, x);
}else{
return -1;
}
}
}
Output:
Enter the number to be found out:
7
Search successful, element found at position 3
Enter the number to be found out:
9
The given element is not present in the array
23. Java program to find the number of odd numbers in an array
class Main {
public static void main(String[] args) {
int[] numbers = {8,3,1,6,2,4,5,9};
int count = 0;
for(int i=0;i<numbers.length;i++){
if(numbers[i]%2!=0)
count++;
}
System.out.println("The number of odd numbers in the list are: "+count);
}
}
Output:
The number of odd numbers in the list are: 4
24. Java program to find the largest number in an array without using built-in functions
class Main {
public static void main(String[] args) {
int[] numbers = {3,8,1,7,2,9,5,4};
int largest = numbers[0];
int position = 0;
for(int i=0;i<numbers.length;i++){
if(numbers[i]>largest){
largest = numbers[i];
position = i;
}
}
System.out.println("The largest element is "+largest+" which is found at position "+position);
}
}
Output:
The largest element is 9 which is found at position 5
25. Java program to insert a number to any position in an array
import java.util.Scanner;
import java.util.Arrays;
class Main {
public static void main(String[] args) {
int[] numbers = {3,4,1,9,6,2,8};
System.out.println(Arrays.toString(numbers));
System.out.println("Enter the number to be inserted: ");
Scanner sc = new Scanner(System.in);
int x = Integer.parseInt(sc.nextLine());
System.out.println("Enter the position: ");
int y = Integer.parseInt(sc.nextLine());
for(int i=numbers.length-1;i>y;i--){
numbers[i] = numbers[i-1];
}
numbers[y] = x;
System.out.println(Arrays.toString(numbers));
}
}
Output:
[3, 4, 1, 9, 6, 2, 8]
Enter the number to be inserted:
50
Enter the position:
4
[3, 4, 1, 9, 50, 6, 2]
26. Java program to delete an element from an array by index
import java.util.Scanner;
import java.util.Arrays;
import java.util.ArrayList;
class Main {
public static void main(String[] args) {
ArrayList<Integer> numbers = new ArrayList<Integer>(5);
numbers.add(3);
numbers.add(7);
numbers.add(1);
numbers.add(4);
System.out.println(numbers);
System.out.println("Enter the position of the element to be deleted: ");
Scanner sc = new Scanner(System.in);
int x = Integer.parseInt(sc.nextLine());
numbers.remove(x);
System.out.println(numbers);
}
}
Output:
[3, 7, 1, 4]
Enter the position of the element to be deleted:
2
[3, 7, 4]
27. Java program to check whether a string is a palindrome or not
import java.util.Scanner;
class Main {
public static void main(String[] args) {
String a, b = "";
Scanner s = new Scanner(System.in);
System.out.print("Enter the string you want to check: ");
a = s.nextLine();
int n = a.length();
for(int i = n - 1; i >= 0; i--){
b = b + a.charAt(i);
}
if(a.equalsIgnoreCase(b)){
System.out.println("The string is a palindrome.");
}else{
System.out.println("The string is not a palindrome.");
}
}
}
Output:
Enter the string you want to check: hello
The string is not a palindrome.
Enter the string you want to check: pop
The string is a palindrome.
28. Java program to implement matrix addition
class Main {
public static void main(String[] args) {
//creating two matrices
int a[][]={{8,5,1},{9,3,2},{4,6,3}};
int b[][]={{8,5,3},{9,5,7},{9,4,1}};
//matrix to store the sum of two matrices
int c[][]=new int[3][3]; //3 rows and 3 columns
//adding 2 matrices
for(int i=0;i<3;i++){
for(int j=0;j<3;j++){
c[i][j]=a[i][j]+b[i][j];
System.out.print(c[i][j]+" ");
}
System.out.print("\n");
}
}
}
Output:
16 10 4
18 8 9
13 10 4
29. Java program to implement matrix multiplication
class Main {
public static void main(String[] args) {
//creating two matrices
int a[][]={{8,5,1},{9,3,2},{4,6,3}};
int b[][]={{8,5,3},{9,5,7},{9,4,1}};
//matrix to store the product of two matrices
int c[][]=new int[3][3];
//multiplying 2 matrices
for(int i=0;i<3;i++){
for(int j=0;j<3;j++){
c[i][j]=0;
for(int k=0;k<3;k++){
c[i][j]+=a[i][k]*b[k][j];
}
System.out.print(c[i][j]+" ");
}
System.out.print("\n");
}
}
}
Output:
118 69 60
117 68 50
113 62 57
30. Java program to check leap year
import java.util.Scanner;
class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("Enter the year you want to check: ");
int year = Integer.parseInt(sc.nextLine());
boolean leap = false;
// if the year is divided by 4
if (year % 4 == 0) {
// if the year is century
if (year % 100 == 0) {
// if year is divided by 400, then it is a leap year
if (year % 400 == 0)
leap = true;
else
leap = false;
}
// if the year is not century
else
leap = true;
}
else
leap = false;
if (leap)
System.out.println(year + " is a leap year.");
else
System.out.println(year + " is not a leap year.");
}
}
Output:
Enter the year you want to check: 2024
2024 is a leap year.
Enter the year you want to check: 2025
2025 is not a leap year.
31. Java program to find the Nth term in a Fibonacci series using recursion
import java.util.Scanner;
class Main {
public static void main(String[] args) {
Scanner cs=new Scanner(System.in);
int n;
System.out.print("Enter the position(N): ");
n=cs.nextInt();
System.out.print("Nth Fibonacci Number is: "+NthFibonacciNumber(n));
}
static int NthFibonacciNumber(int n){
if(n==1)
return 0;
else if(n==2)
return 1;
else
return NthFibonacciNumber(n-1)+NthFibonacciNumber(n-2);
}
}
Output:
Enter the position(N): 7
Nth Fibonacci Number is: 8
32. Java program to print Fibonacci series using iteration
import java.util.Scanner;
class Main {
public static void main(String[] args) {
int n1=0,n2=1;
Scanner cs=new Scanner(System.in);
System.out.print("Enter the number of terms in the sequence: ");
int count = cs.nextInt();
int n3,i;
System.out.print(n1+" "+n2);//printing 0 and 1
//printing from 2 because 0 and 1 are already printed
for(i=2;i<count;++i){
n3=n1+n2;
System.out.print(" "+n3);
n1=n2;
n2=n3;
}
}
}
Output:
Enter the number of terms in the sequence: 8
0 1 1 2 3 5 8 13
33. Java program to implement a calculator to do basic operations
import java.util.Scanner;
class Main {
public static void main(String[] args) {
int firstNumber, secondNumber, opt, add, sub, mul;
double div;
Scanner s = new Scanner(System.in);
System.out.print("Enter first number: ");
firstNumber = s.nextInt();
System.out.print("Enter second number: ");
secondNumber = s.nextInt();
while(true){
System.out.println("Enter 1 for addition");
System.out.println("Enter 2 for subtraction");
System.out.println("Enter 3 for multiplication");
System.out.println("Enter 4 for division");
System.out.println("Enter 5 to Exit");
int option = s.nextInt();
switch(option){
case 1:
add = firstNumber + secondNumber;
System.out.println("Result:"+add);
break;
case 2:
sub = firstNumber - secondNumber;
System.out.println("Result:"+sub);
break;
case 3:
mul = firstNumber * secondNumber;
System.out.println("Result:"+mul);
break;
case 4:
div = (double)firstNumber / secondNumber;
System.out.println("Result:"+div);
break;
case 5:
System.exit(0);
}
}
}
}
Output:
Enter first number: 4
Enter second number: 6
Enter 1 for addition
Enter 2 for subtraction
Enter 3 for multiplication
Enter 4 for division
Enter 5 to Exit
3
Result:24
Enter 1 for addition
Enter 2 for subtraction
Enter 3 for multiplication
Enter 4 for division
Enter 5 to Exit
5
34. Java Program to Find Your Weight on Mars
Mars’ gravity is about 38% of Earth’s. Write a program that will calculate your weight on Mars.
- Declare all variables at the top of the class.
- Initial variables are to be of float type.
- After making the calculations, assign the result to a new variable, this time of the double type.
- After assigning the assignment, write the variable double to the console, limiting its length to 4 decimal places.
- Cast the above variable of double type to a new variable of int type.
- Cast the above variable of type int to a new variable of type char.
- Do any math operation on this variable char type and assign the value of this activity to the new variable int type.
- Each of the above actions should be written to the console, adding some text explaining what has been done.
import java.util.Scanner;
class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("How many pounds (lbs) do you weigh? ") ;
float weight = input.nextFloat();
// computing the weight on mars
double weightOnMars = (weight * .38);
// displaying results with 4 decimal places
System.out.println("Your weight is "+String.format("%.4f",weightOnMars)+" lbs on Mars");
System.out.println("Converting the variable into integer");
int weightOnMarsInt = (int)weightOnMars;
System.out.println(weightOnMarsInt);
System.out.println("Converting the variable into char");
char weightOnMarsChar = (char)weightOnMars;
System.out.println(weightOnMarsChar);
System.out.println("Converting the variable into Int and doing an operation on it");
int newIntVariable = weightOnMarsChar * 2;
System.out.println(newIntVariable);
}
}
Output:
How many pounds (lbs) do you weigh? 152
Your weight is 57.7600 lbs on Mars
Converting the variable into integer
57
Converting the variable into char
9
Converting the variable into Int and doing an operation on it
114
35. Java Program to Check Whether the Generated Random Number Is Even or Odd
Write a program that generates a random number between 1 and 100 (you can use the Random () method from the Math class.
In the next step check whether it is an even or an odd number. Each of the above actions should be written to the console.
import java.util.Random;
class Main {
public static void main(String[] args) {
int min = 1;
int max = 100;
//Generating a random number
Random r = new Random();
int randomNumber = min + r.nextInt(max);
System.out.println("Generated random number is: "+randomNumber);
//Checking whether the number is odd or even
if(randomNumber%2==0){
System.out.println("The generated random number is even.");
}else{
System.out.println("The generated random number is odd.");
}
}
}
Output:
Generated random number is: 25
The generated random number is odd.
36. Java Program to Find the Number of Containers You Need
Choose an odd number between 50 and 100 and save it as an int variable telling us how many Lego bricks we have (e.g. amountOfBricks ), then select an even number between 5 and 10 stating how many Lego blocks fit in one container (e.g.: containerCapacity ) and save it as an int variable as well.
Write a program that will calculate how many full containers we have, how many containers, in general, are full and not full, and how many blocks are in the container that is not completely full (use the modulo operator for this).
import java.util.Scanner;
class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("How many Lego bricks do we have? Choose an odd number between 50 and 100: ");
int amountOfBricks = Integer.parseInt(sc.nextLine());
System.out.println("How many Lego blocks fit in one container? Choose an even number between 5 and 10: ");
int containerCapacity = Integer.parseInt(sc.nextLine());
int noOfFullContainers = amountOfBricks/containerCapacity;
int noOfTotalContainers;
int noOfBlocksInNotFullContainers = amountOfBricks%containerCapacity;
if(noOfBlocksInNotFullContainers!=0){
noOfTotalContainers = noOfFullContainers + 1;
}else{
noOfTotalContainers = noOfFullContainers;
}
System.out.println("No of full containers we have: "+noOfFullContainers);
System.out.println("No of total containers we have: "+noOfTotalContainers);
System.out.println("No of blocks in the container that is not completely full: "+noOfBlocksInNotFullContainers);
}
}
Output:
How many Lego bricks do we have? Choose an odd number between 50 and 100:
75
How many Lego blocks fit in one container? Choose an even number between 5 and 10:
8
No of full containers we have: 9
No of total containers we have: 10
No of blocks in the container that is not completely full: 3
37. Java Program to Calculate Taxes
Using the double types, implement the following: Suppose a product costs 9.99 net, calculate its gross value (we assume VAT of 23%). Then multiply it by 10,000 (i.e., we sold 10,000 pcs of this product), and calculate this value excluding VAT.
Implement the above actions using the Big Decimal class. Print on the console all computed values and compare their values. What conclusions do you have?
import java.math.BigDecimal;
class Main {
public static void main(String[] args) {
double netValue = 9.99;
double VAT = 23.0;
//calculating grossValue
double grossValue = netValue + (VAT*netValue/100);
System.out.println("The gross value is: "+grossValue);
// multiplying the value by 10000
double grossValue10000 = grossValue * 10000;
System.out.println("The gross value for 10000 units is: "+grossValue10000);
// calculating price excluding VAT 23%
double excludingVAT = grossValue10000 - (VAT*grossValue10000/100);
System.out.println("The value for 10000 units excluding VAT is: "+excludingVAT);
// doing the same operations using BigDecimal instead of double
System.out.println("\n----Using BigDecimal instead of double----\n");
BigDecimal netValue_big = new BigDecimal("9.99");
BigDecimal VAT_big = new BigDecimal("23.0");
BigDecimal HUNDRED = new BigDecimal("100");
BigDecimal TenThousand = new BigDecimal("10000");
//calculating grossValue
BigDecimal grossValue_big = netValue_big.add(VAT_big.multiply(netValue_big.divide(HUNDRED)));
System.out.println("The gross value is: "+grossValue_big);
// multiplying the value by 10000
BigDecimal grossValue10000_big = grossValue_big.multiply(TenThousand);
System.out.println("The gross value for 10000 units is: "+grossValue10000_big);
// calculating price excluding VAT 23%
BigDecimal excludingVAT_big = grossValue10000_big.subtract(VAT_big.multiply(grossValue10000_big.divide(HUNDRED)));
System.out.println("The value for 10000 units excluding VAT is: "+excludingVAT_big);
System.out.println("\nThe accuracy is higher when we use BigDecimal instead of double");
}
}
Output:
The gross value is: 12.287700000000001
The gross value for 10000 units is: 122877.00000000001
The value for 10000 units excluding VAT is: 94615.29000000001
----Using BigDecimal instead of double----
The gross value is: 12.28770
The gross value for 10000 units is: 122877.00000
The value for 10000 units excluding VAT is: 94615.290000
The accuracy is higher when we use BigDecimal instead of double
38. Calculate BMI Using Java
The user enters his height (in inches) and weight (in pounds). The variables passed by the user are assigned to the float type. After calculating the BMI value, the value will be assigned to the appropriate range and the correct message will appear on the console. You can use the if-else-if ladder for printing the message on the console.
Intervals of BMI index:
- 16.00 or less = starvation
- 16.00-16.99 = emaciation
- 17.00-18.49 = underweight
- 18.50-22.99 = normal, low range
- 23.00-24.99 = normal high range
- 25.00-27.49 = overweight low range
- 27.50-29.99 = overweight high range
- 30.00-34.99 = 1st degree obesity
- 35.00-39.99 = 2nd degree obesity
- 40.00 or above = 3rd degree obesity
import java.util.Scanner;
class Main {
public static void main(String[] args) {
float height, weight, bmi;
Scanner s = new Scanner(System.in);
System.out.println("Enter the height (in inches): ");
height = s.nextFloat();
System.out.println("Enter the weight (in pounds): ");
weight = s.nextFloat();
bmi = (float)(weight / Math.pow(height, 2) * 703);
System.out.println("The BMI is: "+bmi);
if(bmi<16.00)
System.out.println("starvation");
else if(bmi>=16.00 && bmi <= 16.99)
System.out.println("emaciation");
else if(bmi>=17.00 && bmi <= 18.49)
System.out.println("underweight");
else if(bmi>=18.50 && bmi<=22.99)
System.out.println("normal, low range");
else if(bmi>=23.00 && bmi<=24.99)
System.out.println("normal, high range");
else if(bmi>=25.00 && bmi<=27.49)
System.out.println("overweight, low range");
else if(bmi>=27.50 && bmi<=29.99)
System.out.println("overweight, high range");
else if(bmi>=30.00 && bmi<=34.9)
System.out.println("1st degree obesity");
else if(bmi>=35.00 && bmi<=39.90)
System.out.println("2nd degree obesity");
else
System.out.println("3rd degree obesity");
}
}
Output:
Enter the height (in inches):
69
Enter the weight (in pounds):
150
The BMI is: 22.148708
normal, low range
39. Java Program to Find the Sum of Even Numbers
Write a program that sums even numbers from 1 to 100 using for loop.
class Main {
public static void main(String[] args) {
int sum=0;
for(int i=1;i<=100;i++){
if(i%2==0){
sum = sum + i;
}
}
System.out.println("The sum of even numbers from 1-100 is: "+sum);
}
}
Output:
The sum of even numbers from 1-100 is: 2550
40. Java Program to Find the Largest and Smallest Numbers From Random Numbers
Write a program that will use the while loop to find the largest and smallest number from the set of 10 randomly drawn integers from 1 to 100. In this task, do not use arrays or other collections.
import java.util.Random;
class Main {
public static void main(String[] args) {
int min = 1, max=100;
int largest=0;
int smallest=100;
int i = 1;
while(i<=10){
//Generating a random number
Random r = new Random();
int randomNumber = min + r.nextInt(max);
System.out.println("Generated random number is: "+randomNumber);
if(randomNumber>largest){
largest = randomNumber;
}
if(randomNumber<smallest){
smallest = randomNumber;
}
i++;
}
System.out.println("\n");
System.out.println("The smallest number is: "+smallest);
System.out.println("The largest number is: "+largest);
}
}
Output:
Generated random number is: 68
Generated random number is: 49
Generated random number is: 17
Generated random number is: 44
Generated random number is: 69
Generated random number is: 96
Generated random number is: 59
Generated random number is: 92
Generated random number is: 93
Generated random number is: 49
The smallest number is: 17
The largest number is: 96
41. Java Program to Calculate the Area of a Rectangle
Write a program that follows the rules of object-oriented programming and will calculate the area of the rectangle.
You need to create two classes, one RectangleArea for the logic of your program and the Main class. In the Main class, we create the RectangleArea object and call three methods on it.
The methods you should create are:
- getData(), gets side lengths from the
- computeField(), performs
- fieldDisplay(), displays info and result.
import java.util.Scanner;
class Main {
public static void main(String[] args) {
RectangleArea r = new RectangleArea();
r.getData();
r.computeField();
r.fieldDisplay();
}
}
class RectangleArea{
public double length, width, area;
public void getData(){
Scanner sc = new Scanner(System.in);
System.out.println("Enter the length of the rectangle: ");
length = sc.nextDouble();
System.out.println("Enter the width of the rectangle: ");
width = sc.nextDouble();
}
public void computeField(){
area = length * width;
}
public void fieldDisplay(){
System.out.println("The area of the rectangle is: "+area);
}
}
Output:
Enter the length of the rectangle:
8
Enter the width of the rectangle:
6
The area of the rectangle is: 48.0
42. Returning Information About an Object in Java
Declare the class Car and define the following fields of this class (with the access modifier private): model, brand, year, price, color, and quantity.
Create a constructor of this class consisting of the previously mentioned fields. Create methods to return each of the fields and methods to set values for each of the fields. Additionally, create the sell() method (simulating car sales) which will change the value of the quantity field when called.
The last method to create is the toString() method that returns an object of type String and prints the names of all fields of a given object and values.
Create another class with the main() method in it. Create an object of the Car class by using the constructor. Call the toString() method and print the details.
Call the sell() method. Print all fields (using the previously declared getter methods of the object field).
Using the setter methods, change some fields of the object. Call the toString() method and print the details to notice the changes.
class Main {
public static void main(String[] args) {
Car c1 = new Car("Model S","Tesla",2021,94990,"White",5);
String result = c1.toString();
System.out.println(result);
c1.sell();
System.out.println("\n1 car is sold.\n");
System.out.println(c1.getModel());
System.out.println(c1.getBrand());
System.out.println(c1.getYear());
System.out.println(c1.getPrice());
System.out.println(c1.getColor());
System.out.println(c1.getQuantity());
c1.setYear(2022);
c1.setPrice(110000);
c1.setColor("Black");
c1.setQuantity(10);
System.out.println("\nUpdated some values for the car.\n");
result = c1.toString();
System.out.println(result);
}
}
class Car{
private String model, brand, color;
private int year, price, quantity;
//constructor
Car(String model, String brand, int year, int price, String color, int quantity){
this.model = model;
this.brand = brand;
this.year = year;
this.price = price;
this.color = color;
this.quantity = quantity;
}
//getters
public String getModel(){
return model;
}
public String getBrand(){
return brand;
}
public String getColor(){
return color;
}
public int getYear(){
return year;
}
public int getPrice(){
return price;
}
public int getQuantity(){
return quantity;
}
//setters
public void setModel(String model){
this.model = model;
}
public void setBrand(String brand){
this.brand = brand;
}
public void setColor(String color){
this.color = color;
}
public void setYear(int year){
this.year = year;
}
public void setPrice(int price){
this.price = price;
}
public void setQuantity(int quantity){
this.quantity = quantity;
}
//sell method
public void sell(){
this.quantity = this.quantity - 1;
}
// toString method
public String toString(){
String output = "Model: "+this.model+"\nBrand: "+this.brand+"\nYear: "+this.year+"\nPrice: "+this.price+"\nColor: "+this.color+"\nQuantity: "+this.quantity;
return output;
}
}
Output:
Model: Model S
Brand: Tesla
Year: 2021
Price: 94990
Color: White
Quantity: 5
1 car is sold.
Model S
Tesla
2021
94990
White
4
Updated some values for the car.
Model: Model S
Brand: Tesla
Year: 2022
Price: 110000
Color: Black
Quantity: 10
43. Filling an Array Using For Loops in Java
Write a program that creates a 10-element array and puts the numbers from 9 to 0 in it.
Use the classic for loop to fill the array, and in the condition, use the array.length method. Use for-each loop as a second loop to display the value of the array.
class Main {
public static void main(String[] args) {
int[] numbers = new int[10];
int value = 9;
for(int i=0;i<numbers.length;i++){
numbers[i] = value;
value--;
}
for (int i : numbers){
System.out.println(i);
}
}
}
Output:
9
8
7
6
5
4
3
2
1
0
44. Java Program to Find the Largest and Smallest Numbers From an Array of Random Numbers
Write a program that will create a one-dimensional array with 10 elements that are chosen randomly from 1 to 100. Then use a loop to find the smallest and largest element in the array.
import java.util.Random;
class Main {
public static void main(String[] args) {
int min = 1, max=100;
int largest=0;
int smallest=100;
int[] numbers = new int[10];
for(int i=0;i<numbers.length;i++){
Random r = new Random();
int randomNumber = min + r.nextInt(max);
numbers[i] = randomNumber;
}
for (int i : numbers){
System.out.println(i);
if(i>largest)
largest = i;
if(i<smallest)
smallest = i;
}
System.out.println("The largest number is: "+largest);
System.out.println("The smallest number is: "+smallest);
}
}
Output:
6
83
72
6
25
67
82
6
37
49
The largest number is: 83
The smallest number is: 6
45. Two-dimensional Array in Java
Write a program that creates a two-dimensional array with dimensions of 10×10 and named matrix. On the diagonal of this matrix, put the numbers from 0 to 9 and the number 0 everywhere else. Additionally, the program should calculate the sum of the elements on the diagonal.
class Main {
public static void main(String[] args) {
int[][] matrix = new int[10][10];
int number = 0;
int sum = 0;
for(int i=0; i<matrix.length;i++){
for(int j=0; j<matrix[i].length;j++){
if(i==j){
matrix[i][j] = number;
number++;
}else{
matrix[i][j] = 0;
}
}
}
for(int i=0; i<matrix.length;i++){
for(int j=0; j<matrix[i].length;j++){
System.out.print(matrix[i][j]+" ");
if(i==j){
sum = sum + matrix[i][j];
}
}
System.out.println();
}
System.out.println("The sum of diagonal numbers is: "+sum);
}
}
Output:
0 0 0 0 0 0 0 0 0 0
0 1 0 0 0 0 0 0 0 0
0 0 2 0 0 0 0 0 0 0
0 0 0 3 0 0 0 0 0 0
0 0 0 0 4 0 0 0 0 0
0 0 0 0 0 5 0 0 0 0
0 0 0 0 0 0 6 0 0 0
0 0 0 0 0 0 0 7 0 0
0 0 0 0 0 0 0 0 8 0
0 0 0 0 0 0 0 0 0 9
The sum of diagonal numbers is: 45
What a wonderful template! I found what I needed and more, Keep It Up Legend <3
*
*2
*2*
*2*4
*2*4*
Drawn above pattern in java ?
Your Centigrade converter doesn’t work. You need 9f instead of 9, or rounding will give you the wrong answer.
Thank you for pointing out the mistake. I have corrected the code.
It is to most and very workfull 🤩🤩
You have done it well, sir