Features of Java
- Simple: Java has a clean and simple syntax. Java is easy to learn, especially for those who already know C++.
- Object-oriented: Every entity in Java is an object
- Platform-independent: Java code is compiled by the compiler and converted into bytecode, which is a platform-independent code. The byte code can be run on multiple platforms or operating systems.
- Secure: Java provides several security features
- Robust: Java is robust because it uses strong memory management. Java provides automatic garbage collection which gets rid of objects which are not being used by a Java application anymore.
- Compiled & interpreted: Java can be considered both a compiled and an interpreted language. The Java source code is first compiled into a binary byte code. This byte code runs on the Java Virtual Machine (JVM), which is a software-based interpreter.
Java Installation and Setup
You can check out this post for installing Java on your system. You can also use an online editor like replit.com if you want to get started without worrying about setting up Java on your system.
Hello World Java Program in Java
class Main{
public static void main(String[] args){
System.out.println("Hello World");
}
}
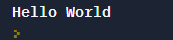
Comments in Java
class Main{
public static void main(String[] args){
// This is a single line comment
/* This is a
multi-line
comment */
}
}

Data Types and Variables in Java
class Main{
public static void main(String[] args) {
int count = 45;
float avg = 22.4f;
double salary = 27782.22;
char letter = 'A';
String sentence = "Good afternoon";
boolean result = false;
System.out.println(count);
System.out.println(avg);
System.out.println(salary);
System.out.println(letter);
System.out.println(sentence);
System.out.println(result);
}
}
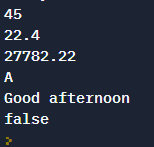
Reading Data from the Console in Java
import java.util.Scanner;
class Main{
public static void main(String[] args){
Scanner sc = new Scanner(System.in);
int count = sc.nextInt();
sc.nextLine();
String name = sc.nextLine();
System.out.println(count +" "+ name);
}
}
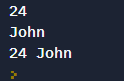
The nextInt() method doesn’t move the cursor to the next line. So, you need to use sc.nextLine() to move to the next line.
You can also read integers or non-string data types using sc.readLine() and convert that into the required data type.
import java.util.Scanner;
class Main{
public static void main(String[] args){
Scanner sc = new Scanner(System.in);
int count = Integer.parseInt(sc.nextLine());
String name = sc.nextLine();
System.out.println(count +" "+ name);
}
}
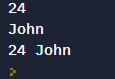
Operators in Java
class Main{
public static void main(String[] args) {
int a = 10;
int b = 5;
int c = 15;
//arithmetic operators
System.out.println(a+b);
System.out.println(a-b);
System.out.println(a*b);
System.out.println(a/b);
System.out.println(a%b);
//unary operators (increment)
System.out.println(a++); //a becomes 11 after print
System.out.println(++a); //a becomes 11 before print
//relational operators
System.out.println(a<b);
System.out.println(a>b);
System.out.println(a>=b);
System.out.println(a==b);
System.out.println(a!=b);
//logical operators
System.out.println(a>b && a<c); //true && true
System.out.println(a>b && a>c); // true && false
System.out.println(a>b || a>c); // true || false
//assignment operators
int value = 200;
value += 30; // value = value + 30
value -= 10; // value = value - 10
}
}
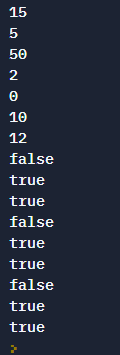
Conditional Statements in Java
If-Else Statements in Java
class Main{
public static void main(String[] args) {
// if else statements
int x = 15;
if(x>15){
System.out.println("X is greater than 15");
}
else if(x<15){
System.out.println("X is less than 15");
}
else{
System.out.println("X is equal to 15");
}
}
}
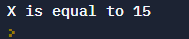
Switch Case Statements in Java
class Main{
public static void main(String[] args){
//switch-case statements
int y = 30;
switch(y){
case 20:
System.out.println("y is 20");
break;
case 25:
System.out.println("y is 25");
break;
case 30:
System.out.println("y is 30");
break;
default:
System.out.println("y is unknown");
}
}
}
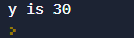
Loops in Java
While Loop in Java
class Main{
public static void main(String[] args){
int x = 1;
//while loop
while(x<=10){
System.out.println(x);
x++;
}
}
}
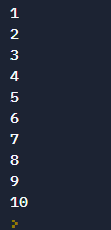
Do-While Loop in Java
class Main{
public static void main(String[] args){
//do-while loop
// gets executed at least once
int a = 10;
do{
System.out.println(a);
a++;
}while(a<=20);
}
}
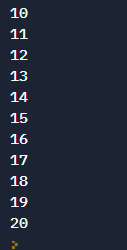
For Loop in Java
class Main{
public static void main(String[] args){
//for loop
//for(initialize, condition, increment)
for(int i=0;i<=10;i++){
System.out.println(i);
}
}
}
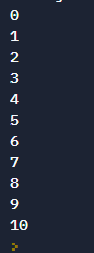
Arrays in Java
class Main{
public static void main(String[] args){
//defining an array
String[] players = {"Ronaldo","Messi","Neymar","Mbappe"};
int[] numbers = {5,7,9,11,23};
//accessing values inside an array
System.out.println(players[1]);
System.out.println(numbers[3]);
//finding the length of an array
System.out.println(numbers.length);
//looping through an array
for(int i=0;i<players.length;i++){
System.out.println(players[i]);
}
//another way of looping through an array
for (String i: players){
System.out.println(i);
}
}
}
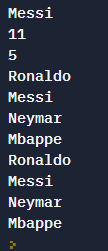
Multi-dimensional Arrays in Java
class Main{
public static void main(String[] args){
// multi-dimensional array (2D)
int[][] myNumbers = {{1,2,3}, {4,5,6}};
// access values in a multi-dimensional array
int z = myNumbers[1][1];
System.out.println(z);
//looping through a multi-dimensional array
for(int i=0; i<myNumbers.length;i++){
for(int j=0; j<myNumbers[i].length;j++){
System.out.println(myNumbers[i][j]);
}
}
}
}
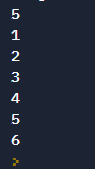
Objects and Methods in Java
class Main {
public static void main(String[] args) {
//creating an object of Car
Car c1 = new Car();
//calling a method using the object
c1.insertData(4536262,"Tata Nexon");
c1.display();
//calling the add method that returns the sum
int result = c1.addNumbers(23, 11);
System.out.println(result);
}
}
class Car{
// private & public are access modifiers
public int price;
private String name;
// a method to insert data to the variables
void insertData(int p, String n){
price = p;
name = n;
}
void display(){
System.out.println("The car name is "+name+" and the price is "+price+" Rupees");
}
// a method that takes two values & returns their sum
int addNumbers(int x, int y){
int sum = x+y;
return sum;
}
}

Constructors in Java
class Main {
public static void main(String[] args) {
//creating an object of Car
Car c1 = new Car(4536262,"Tata Nexon");
c1.display();
}
}
class Car{
// private & public are access modifiers
public int price;
private String name;
// a method to insert data to the variables
Car(int p, String n){
price = p;
name = n;
}
void display(){
System.out.println("The car name is "+name+" and the price is "+price+" Rupees");
}
}

Object Arrays in Java
class Main {
public static void main(String[] args) {
Car[] myCars = new Car[5];
myCars[0] = new Car(123,"Tata Harrier");
myCars[1] = new Car(234,"Tata Nano");
myCars[2] = new Car(453,"Tata Altroz");
myCars[3] = new Car(678,"Tata Safari");
myCars[4] = new Car(699,"Tata Nexon");
Car.displayValues(myCars);
myCars[0].setPrice(235);
System.out.println(myCars[0].getPrice());
System.out.println(myCars[0].name);
}
}
class Car{
private int price;
String name;
public int getPrice(){
return price;
}
public void setPrice(int newPrice){
this.price = newPrice;
}
Car(int p, String n){
price = p;
name = n;
}
static void displayValues(Car[] myCars){
for(int i=0;i<myCars.length;i++){
System.out.println(myCars[i].name);
}
}
}
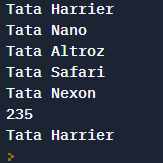
Inheritance in Java
Inheritance is the process of deriving a new class from an old class (parent class). The old class is called the superclass and the new one is called the subclass. The subclass can use all the stuff that is inside a superclass.
We use the extends keyword in Java to inherit a class.
class Main{
public static void main(String[] args){
TataNexon myCar = new TataNexon();
myCar.startCar();
myCar.changeGear();
myCar.openSunroof();
}
}
class Car{
void startCar(){
System.out.println("Starting the car...");
}
void changeGear(){
System.out.println("Changing the gear...");
}
}
class TataNexon extends Car{
void openSunroof(){
System.out.println("Opening the sunroof...");
}
}
Output:
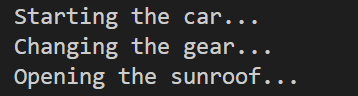
Polymorphism in Java
Polymorphism is the property by which objects belonging to different classes are able to respond to the same message but in different ways.
We can carry out polymorphism through inheritance, with subclasses making use of superclass methods or overriding them so that the same method works differently based on the object that we are working with.
In short, polymorphism allows coders to perform a single action in different ways.
We can achieve polymorphism in Java using method overriding and method overloading.
Method Overriding in Java
The process in which a call to an overridden method is resolved at runtime is called method overriding (or runtime polymorphism). This happens when a child class has a method definition for one of the member functions of the parent class. In this case, the child method will override the parent method.
An example of polymorphism in Java using method overriding:
class Main{
public static void main(String[] args){
Animal myAnimal = new Animal();
Animal myCat = new Cat();
Animal myDog = new Dog();
myAnimal.makeSound();
myCat.makeSound();
myDog.makeSound();
}
}
class Animal {
public void makeSound() {
System.out.println("The animal makes a sound...");
}
}
class Cat extends Animal {
public void makeSound() {
System.out.println("Meow Meow...");
}
}
class Dog extends Animal {
public void makeSound() {
System.out.println("Bow Bow...");
}
}
Output:
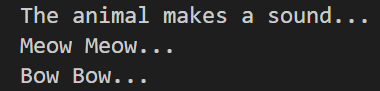
Method Overloading in Java
Overloading occurs when there are multiple functions with the same name but different parameters. We can overload functions by changing the number of arguments or/and by changing the data type of arguments.
An example of polymorphism in Java using method overloading:
class Main{
public static void main(String[] args){
System.out.println(Addition.addNumbers(5, 3));
System.out.println(Addition.addNumbers(6, 9, 2));
System.out.println(Addition.addNumbers(7.32, 4.19, 3.14));
}
}
class Addition {
static int addNumbers(int a, int b){
return a + b;
}
static int addNumbers(int a, int b, int c){
return a + b + c;
}
static double addNumbers(double a, double b, double c){
return a + b + c;
}
}
Output:
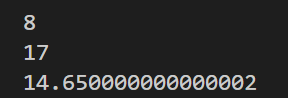
ArrayList in Java
ArrayList in Java provides a dynamic array for storing the elements. There is no size limit to an ArrayList. We can add or remove elements as required.
import java.util.*;
class Main{
public static void main(String[] args){
//arraylist of string values
ArrayList<String> players =new ArrayList<String>();
players.add("Ronaldo");
players.add("Messi");
players.add("Neymar");
players.add("Mbappe");
System.out.println(players);
//size of the arraylist
System.out.println(players.size());
//sorting
Collections.sort(players);
System.out.println(players);
//looping
for(String player:players){
System.out.println(player);
}
//removing
players.remove(0);
System.out.println(players);
//clearing
players.clear();
System.out.println(players);
//arraylist of integers
ArrayList<Integer> numbers = new ArrayList<Integer>();
numbers.add(43);
numbers.add(27);
System.out.println(numbers);
}
}
Output:
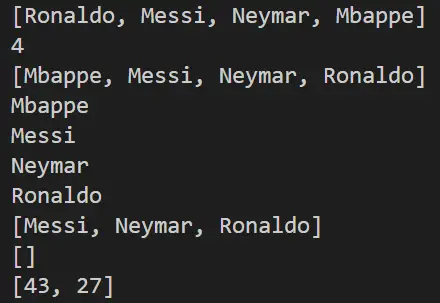
HashMap in Java
Java HashMap allows us to store key and value pairs, where keys should be unique.
import java.util.HashMap;
import java.util.Map.Entry;
class Main{
public static void main(String[] args){
HashMap<Integer,String> players=new HashMap<Integer,String>();
players.put(7,"Ronaldo");
players.put(10,"Messi");
players.put(11,"Neymar");
players.put(9,"Benzema");
System.out.println(players);
// accessing a value using a key in hashmap
System.out.println(players.get(7));
//key-based removal
players.remove(11);
System.out.println(players);
//key-value pair based removal
players.remove(9, "Benzema");
System.out.println(players);
// iterating over the hashmap
for(Entry<Integer, String> p : players.entrySet()){
System.out.println(p.getKey()+" "+p.getValue());
}
}
}
Output:
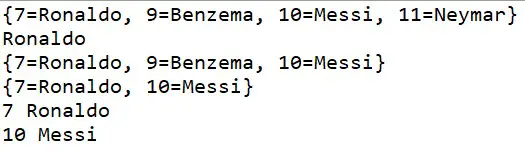
Exception Handling
Here is the syntax for exception handling in Java:
try {
// Block of code to try
}
catch(Exception e) {
// Block of code to catch errors and handle them
}
finally{
// optional block. Gets executed after try-catch, regardless of the result
}
Example of Exception Handling:
public class Main {
public static void main(String args[]){
// ArithmeticException
try{
System.out.println(10/0);
}catch(Exception e){
System.out.println(e);
}
// NullPointerException
int[] myNumbers = null;
try{
System.out.println(myNumbers.length);
}catch(Exception e){
System.out.println(e);
}
//ArrayIndexOutOfBoundsException
int[] myArray = {1,2,3,4};
try{
System.out.println(myArray[6]);
}catch(Exception e){
System.out.println(e);
}
// StringIndexOutOfBoundsException
String myString = "Hello";
try{
System.out.println(myString.charAt(6));
}catch(Exception e){
System.out.println(e);
}
// NumberFormatException
int num;
try{
num = Integer.parseInt("Hello");
}catch(Exception e){
System.out.println(e);
}
}
}
An example that handles the error until the user enters the correct input:
import java.util.Scanner;
public class Exc {
public static void main(String args[]){
Scanner sc = new Scanner(System.in);
// Let's say the number can't be less than 0 and a string
boolean success = false;
while(!success){
try{
int n = Integer.parseInt(sc.nextLine());
if(n<=0){
throw new Exception("Invalid number. Please try again.");
}else{
success = true;
System.out.println("The number is "+n);
}
}catch(Exception e){
System.out.println(e);
continue;
}
}
sc.close();
}
}
Interface in Java
An interface in Java defines a contract. If a class implements that contract by inheriting the interface, then it must provide an implementation of the members defined inside the interface.
An interface is used to achieve abstraction and multiple inheritance in Java. You can only put abstract methods in a Java interface, not a method body.
public class Main {
public static void main(String[] args){
Mailbox mb = new Mailbox();
myEmail e = new Gmail();
e = new Outlook();
mb.send(e);
}
}
interface myEmail{
void sendMail();
}
class Gmail implements myEmail{
public void sendMail(){
System.out.println("Mail sent through Gmail!");
}
}
class Outlook implements myEmail{
public void sendMail(){
System.out.println("Mail sent through Outlook!");
}
}
class Mailbox{
void send(myEmail e){
e.sendMail();
}
}
JDBC
Steps for setting up JDBC:
Sample code for JDBC:
import java.sql.*;
public class Main {
public static void main(String[] args) throws SQLException {
Connection c = DBConnection.getConnection();
insertData(c);
updateData(c);
deleteData(c);
String sql = "SELECT * FROM Student";
selectData(c,sql);
c.close();
}
public static void selectData(Connection c, String sql) throws SQLException{
Statement stmt = c.createStatement();
ResultSet rs = stmt.executeQuery(sql);
while (rs.next()) {
System.out.println(rs.getInt(1));
System.out.println(rs.getString(2));
}
}
public static void insertData(Connection c) throws SQLException{
PreparedStatement insertStudent = c.prepareStatement("insert into Student values(?,?)");
insertStudent.setInt(1,2);
insertStudent.setString(2, "Bob");
insertStudent.executeUpdate();
}
public static void updateData(Connection c) throws SQLException{
Statement stmt = c.createStatement();
String sql = "UPDATE Student SET Name = 'Joe' WHERE rollno=1";
stmt.executeUpdate(sql);
}
public static void deleteData(Connection c) throws SQLException{
Statement stmt = c.createStatement();
String sql = "DELETE from Student WHERE rollno=1";
stmt.executeUpdate(sql);
}
}
class DBConnection {
private static final String DRIVERNAME = "org.apache.derby.jdbc.EmbeddedDriver";
private static final String URL = "jdbc:derby:C:\\Users\\1721025\\MyDB;create=true";
// private static final String PASSWORD = "123";
// private static final String USERNAME = "ashwin";
public static Connection getConnection() {
Connection conn = null;
try{
Class.forName(DRIVERNAME);
conn = DriverManager.getConnection(URL);
System.out.println("Connection Established");
}
catch(Exception E1){
System.out.println(E1);
}
return conn;
}
}