Python is a high-level programming language that lets you work quickly and integrate systems more effectively while emphasizing code readability and beginner-friendliness. It has a simple syntax and is highly portable, reliable, and versatile. Python has an extensive library of built-in modules. It has a large user base and community to reach out to in case of any queries and doubts.
Python is used in almost every field and domain today. It is currently used in web development, data science, and scripting. Python helps developers to create various task automation scripts. Businesses and companies use Python for most of their software applications. Python also facilitates the creation of machine learning and artificial intelligence algorithms.
Currently, there is an increased and renewed interest in Python among recruiters. So I have constructed the ultimate guide with the top 100 Python interview questions that might be useful for your Python journey.
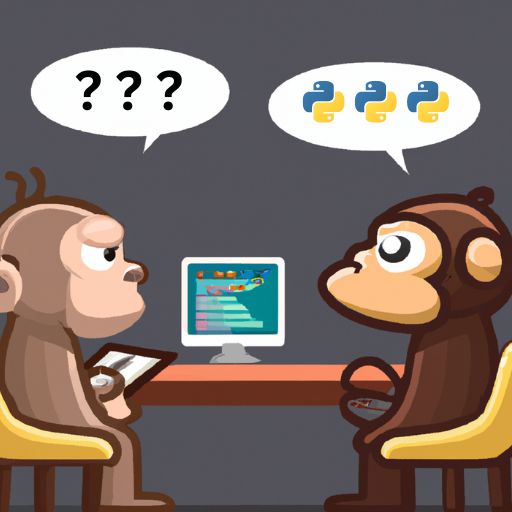
Let’s dive right in.
1. What are Python iterators?
Iterators are objects which can be traversed through or iterated upon loops, arrays, etc. Coders use an iter() method, which is used to get an iterator.
It is not necessary to use the iter() method to create an iterator in Python. Many objects, such as lists, tuples, and strings, are iterable and can be used directly in a for loop or with the next method. However, if you have an object that is not iterable, you can use the iter() method to create an iterator for it.
Example:
a=[1,2,3,4,5]
for i in a:
print(i)
Output :
1 2 3 4 5
The for loop creates an iterator object and automatically executes the next() method for each loop.
2. What is self in Python?
Self is used to refer to the current instance of a class. In Python, it is explicitly included as the first parameter. In Java, it is optional to use self. Self differentiates between the attributes and methods of the class with local variables.
The self variable in the init method points to the newly created object, while in other methods, it is referred to the object whose method was called.
In Python, instance methods are called on an instance of a class, and they automatically receive a reference to the instance as the first argument (which is traditionally called self ). This is different from languages like Java, where instance methods are called on an instance of a class and receive an implicit this reference to the instance.
3. What is the lambda function in Python?
The lambda function is a small anonymous function that is not bound to an identifier. The function can have any number of parameters but can only have one statement.
The lambda functions are defined using the lambda keyword, and they can be used to create quick throwaway functions for simple tasks.
Example:
x = lambda x,y : x+y
print(x(1,2))
Output :
3
Here, the lambda function is used for the addition of two numbers called using parameters.
4. What is init in Python?
The __init__ is a method or constructor in Python. It is automatically called to allocate memory when a new object of a class is created. The __init__ method initializes the attributes of the instance.
Example:
class Student:
def __init__(self, name):
self.name=name
S = Student('Ashwin')
print(S.name)
Output :
Ashwin
5. Is Python case-sensitive?
Yes, python is a case-sensitive language. In Python, identifiers (variable names, function names, and class names) are treated as distinct based on the case of the letters in their names.
Example:
a = 5
A = 8
print(a) # Output: 5
print(A) # Output: 8
Output:
5 8
6. What are functions in Python?
A function is a block of code that is executed when it is called. Functions help you to reuse and modularize your code, making it easier to write and maintain.
Example :
def addition(a,b):
return a+b
x=addition(3,2)
print(x)
Output :
5
7. What are local and global variables in Python?
Local variables are variables defined inside a function and are accessible only inside the body of the function.
Global variables are variables that are defined outside of any function or method, and they have a global scope and are accessible throughout the program.
Example:
x = 10 # x is a global variable
def my_function():
global x
x = 20 # Modify the global variable x
y = 99 # y is a local variable
print(x)
print(y)
my_function() # Output: 20
print(x) # Output: 20
print(y) # Causes an Error
8. How do Python functions work without brackets?
When a function call contains brackets, the code gets executed, and the result is returned. Without brackets present in the function call, the function reference is returned and it will not get executed.
This technique of getting a reference to a function object is often used when you want to pass a function as an argument to another function, or when you want to assign a function to a variable or data structure for later use.
9. What are Python modules? Name some of the commonly used built-in modules in Python.
Python modules are simple files with the file extension .py containing python code which can be reused and imported into other python programs.
Modules help in organizing and reusing code, as they allow you to define code in a separate file and then import it into your program as needed. This helps to make your code more modular and easier to maintain.
Example:
# In a file called sample.py:
def greet(name):
print("Hello, " + name + "!")
# In another file:
import sample
sample.greet("Ashwin") # Output: "Hello, Ashwin!"
There are many built-in modules available in Python. Some commonly used built-in modules in python are operator, decimal, random, string, and math.
10. What is a namespace in Python?
Namespace uses a unique naming system for every object in the program, which identifies all object names inside a program. It is a declarative region providing scope to identifiers. A namespace is used to prevent naming conflicts between different identifiers in a program.
There are three types of namespaces in python: global, local, and built-in.
Example:
x = 10 # x is a global variable
def printValue():
x = 20 # x is a local variable
print(x)
printValue() # Output: 20
print(x) # Output: 10
When we call the printValue function, it prints the value of the local variable x, which is 20. When we print the value of the global variable x from the global scope, it has the value 10, because the local variable x defined in the printValue function is in a different namespace than the global variable x.
11. How is memory managed in Python?
Memory is managed in Python by a private heap containing all objects and data structures. The memory manager allocates a heap space for Python objects to be stored.
One important feature of memory management in Python is its use of reference counting to keep track of the number of references to an object. When an object is no longer needed, the memory manager automatically deallocates the object’s memory by decrementing the object’s reference count. When the reference count reaches zero, the memory manager frees the object’s memory and it becomes available for reuse.
Python also uses a garbage collector to periodically scan the heap and deallocate objects that are no longer needed.
12. What is pep8 in Python?
PEP8(Python Enhancement Proposal) is a document that provides rules and guidelines on how to improve the readability of Python code.
The pep8 tool is a command line utility that checks your python program’s code style conventions with PEP8.
13. Is Python a programming language or a scripting language?
Python is both a scripting language and a general-purpose programming language. Python is often referred to as a “scripting language” because python programs generally contain scripts that translate the source code.
14. How does Python work as an interpreted language?
Python works as an interpreter language as it converts the source code of a program into bytecode. It simply executes code without compilation and interprets it line by line. Python code isn’t required to be compiled, compared to programming languages like C and C++.
15. What is type conversion in Python?
Type conversion is the conversion of an object from one data type to another. There are two types of type conversion: implicit and explicit. By default, the Python interpreter performs implicit type conversion.
16. List the built-in data types in Python.
The built-in data types in Python are Numeric (int, float, complex), Sequence type (list, tuple), Mapping type (dict), Boolean (bool), Set (set), Text type (str), etc.
17. What are tuples in Python?
In Python, the tuple is a collection of objects separated by a comma. They are used to store multiple items in a single variable. A tuple is similar to lists but it is immutable, ordered, and unchangeable.
18. What is pass in python?
The pass keyword is a placeholder for future code additions. It fills up empty blocks of code to prevent code conflicts and errors during runtime. It represents a null operation in Python.
Example 1:
a = 200
b = 33
if b > a:
print("b is greater than a")
else:
pass
Output 1:
No output as b is less than a and the pass keyword is used.
Example 2:
a = 200
b = 330
if b > a:
print("b is greater than a")
else:
pass
Output 2:
b is greater than a
19. What is PYTHONPATH?
PYTHONPATH is an environment variable used when importing a module. It sets the path for user-defined modules used in the Python program. It lets you configure additional directories for Python to look into for modules and packages.
20. What are Python Decorators?
Decorator allows programmers to modify or alter the behavior of a function or class. It is a design pattern that facilitates us to add new functionalities to existing objects present in the program without modifying its structure.
21. What are iterators in Python?
Iterators in Python are objects representing a stream of data that can be iterated or traversed. It allows the user to sequentially traverse elements within a container, such as a list or a string, without exposing the underlying implementation of the container.
22. Why do we need to use a break and continue commands in a loop in Python?
The break and continue commands are used to control the flow of execution within a loop. The break command breaks the current loop and controls the Python loop by preventing it from execution and transferring the control to the next block.
The continue command skips the current iteration of the loop and jumps to the next iteration of the loop.
23. What are python packages?
Python packages are a collection of several modules and sub-packages based on similar functions. It is a folder containing various modules and sub-folders with modules.
24. How do we create a multiline comment in python?
We can create a multi-line comment in python by enclosing the text in a delimiter (”’) on both ends of the comment.
For example:
'''
This is a
multi-line
comment
'''
We can also do the same by prefixing the start of each line using #.
#This is a
#multi-line
#comment
25. What is a classifier in Python?
Classifier in Python predicts the class of any data points. It is a machine learning model used in assigning class labels to particular data points. It uses training data to understand the relationship between input variables and the class.
26. What is recursion in Python?
Recursion is a technique using functions and algorithms that calls itself one or more times in its own definition. A recursive function should have a condition that stops the recursion to prevent an infinite loop. Python supports recursion in the program for the defined functions to call itself.
27. What is the use of the bytes() function?
The bytes() function converts objects into bytes objects or creates empty bytes objects of a particular size. It returns a byte object, which is an immutable sequence of integers in the range of 0 <= x < 256.
28. What is Encapsulation in Python?
Encapsulation binds the code and data together. Python supports encapsulation, but it doesn’t have a private keyword. Python relies on a class variable that is prefixed with an underscore and can’t be directly accessed. In Python, code and data are bundled together in a single unit.
29. What is the difference between .py and .pyc files?
.py are the source code files that the python interpreter interprets. Whereas .pyc compiled files are bytecodes generated by the python compiler for inbuilt modules/files.
30. When is a Python code referred to as Pythonic?
Python code is said to be Pythonic if it is not only syntactically correct but also follows the guidelines and conventions of the Python community.
31. Define a string in Python.
A string is a sequence of characters, immutable and written within single or double quotes interpreted by a Python script. In Python, strings are used to represent text, which can include letters, numbers, symbols, and spaces. They are arrays of bytes that represent Unicode characters.
32. What does a Python Virtual Machine perform?
The Python Virtual machine(PVM) is responsible for executing the bytecode and converting the bytecode instructions generated from the Python source code into machine code to display output. PVM provides a programming environment.
33. What is a statement in Python?
A statement is the smallest executable unit within a Python program. It specifies instructions given to the machine to perform specific tasks.
34. What is a loader in Python?
A loader is an object that loads a module that can be imported. These loaders use one or more dataset sources and create Entry, Entity, and Classifier entries in the database.
35. What is a class in Python?
A class in Python acts as a template for creating user-defined objects. It is a user-defined data structure holding its data members and member functions accessed by creating an instance with the class.
36. What is a file object in Python?
A file object allows us to access and modify all the user-accessible files by reading and writing to any such files. It is an object which exposes a file-oriented API to an underlying resource. Whenever we open a file to perform various operations, Python returns a file object.
37. List out how a reverse string can be applied in a program.
A reverse string can be applied using five different ways in a program. We can reverse it using Loops, Recursion, Stack, Extended Slice Syntax, and Reversed.
38. What are assignment operators in Python?
Assignment Operators are used for assigning values to variables. In Python, we use the “=” symbol as the most common assignment operator. They use special symbols to carry out various arithmetic, logical and bitwise operations.
Here are some examples of using assignment operators.
x = 5
x += 1 #is equivalent to x = x + 1
x *= 2 #is equivalent to x = x * 2
x /= 3 #is equivalent to x = x / 3
39. Is Python Platform Independent?
Yes, Python is platform-independent as most modules and functions are available on every platform. We use Python on different platforms by building an interpreter tailored for that particular platform.
40. What is the use of self in Python?
Self is used to represent a class instance. With this keyword, the class’s attributes and methods are accessed. Self connects the attributes to the arguments and appears in various contexts.
The keyword “self” is always the first parameter in a class method definition, and it is automatically passed by the Python interpreter when the method is called. It is used to distinguish between instance variables and class variables.
41. What are Literals in Python? Name its types.
A literal in Python source code indicates a fixed value for primitive data types. There are five types of literals in Python. The types of literals in Python are String literal, Numeric literal, Character Literal, Boolean Literal, and Literal Collections.
42. What are docstrings?
Docstrings are documentation strings used to document a Python module, class, function, etc. so that Python coders can understand what it does without having to read the details of the implementation.
Docstrings are declared inside triple quotations and aren’t allocated to any variable. So they can also be used as comments in a program.
Example:
def myFunction():
'''This is an example of
a docstring in Python.'''
return None
#print(myFunction.__doc__)
help(myFunction)
Output:
Help on function myFunction in module __main__:
myFunction()
This is an example of
a docstring in Python.
43. What is a dictionary in Python?
A dictionary is a built-in datatype in Python. It creates a correspondence between keys and values. Dictionary keys and values get stored in pairs in dictionaries. The keys are used for indexing dictionaries.
44. What are the best ways to add elements to an Array in Python?
The best way to add elements to an array in Python is by using append(), extend(), and insert() methods.
45. What are Python Libraries?
A Python library is a group of related Python packages and modules used in different programs. Numpy, Pandas, Matplotlib, Scikit-learn, and many other Python libraries are widely used today.
46. How to import modules in Python?
We can import modules in Python using the import keyword.
47. List some Python libraries used for machine learning.
NumPy, SciPy, Scikit-learn, Theano, TensorFlow, Keras, PyTorch, and Pandas are some Python libraries used for machine learning today.
48. What is slicing in Python?
Slicing is a technique used for accessing specific bits of sequences such as strings, tuples, and lists. Slicing is a simple flexible tool used to build new lists out of an existing list.
49. How do you make a Python script executable in Unix?
The script file should start with #!/usr/bin/env python.
#! tells the shell about which program to execute. Then we can chmod the Python file to make it executable, and run it using the bash command (./scriptname).
50. What is MRO in Python?
MRO (Method Resolution Order) defines the order in which the Python Interpreter looks for a method in a hierarchy of classes.
51. Is Multiple Inheritance supported in python?
Yes, unlike Java, Python offers a wide range of inheritance and usage support to its users. Multiple inheritances describe a situation in which a class is instantiated from more than one parent class. This gives users a lot of functionality and benefits.
52. What do you understand by the word Tkinter?
Tkinter is a Python module for creating graphical user interfaces. It is Python’s standard GUI development toolkit. Tkinter is included with Python, so there is no need for a separate installation. You can begin by importing it into your script.
53. Explain the use of the ‘with’ statement and its syntax.
Using the ‘with’ statement in Python, we can open a file and close it when the block of code containing the ‘with’ statement exits. We can avoid using the close() method this way.
Example:
with open(“filename” , “mode”) as file_var:
# then use the file
54. What do *args and **kwargs mean?
-> *args is used to pass multiple arguments in a function.
-> **kwargs is used to pass multiple keyword arguments in a function in python.
55. In Python, how do ternary operators work?
The ternary operator is used to display conditional statements in Python. This consists of boolean true or false values and a statement that must be checked.
Syntax:
[on_true] if [expression] else [on_false]
Example:
x = 10
y = 20
count = x if x<y else y
print(count)
Output:
10
Explanation:
In the above expression, if x<y is true, the value is returned as count=x; if it is false, count=y is stored in the result.
56. Explain the split() method of the ‘re’ module in python.
This method belongs to the Python RegEx or ‘re’ module and is used to modify strings.
-> split() method is generally used to split a given string into a list at each occurrence of a specified character.
Example:
import re
words = re.split("/","Hello/Hi/Good/Morning")
print(words)
Output:
['Hello', 'Hi', 'Good', 'Morning']
57. Explain sub() and subn() methods of the ‘re’ module in python.
These methods belong to the “re” module of Python and are used to modify strings.
-> sub(): This method finds a substring that matches a regex pattern and then replaces the matched substring with a different string.
Example:
import re
phoneNumber = '999-456-1800'
pattern = '-'
result = re.sub(pattern,'',phoneNumber)
print(result)
Output:
9994561800
-> subn(): This method is similar to sub() in that it returns the new string as well as the number of replacements.
Example:
import re
phoneNumber = '999-456-1800'
pattern = '-'
result = re.subn(pattern,'',phoneNumber)
print(result)
Output:
('9994561800', 2)
58. Can we make multi-line comments in Python?
Python does not have a syntax for displaying multi-line comments like other languages. Python programmers use triple-quoted (docstrings) strings to display multi-line comments. The Python parser will ignore the docstring if it is not used for anything.
59. What is the difference between range and xrange?
Both methods are primarily used in Python to iterate the for loop for a predetermined number of times.
They differ when it comes to Python versions. The xrange() and range() functions are used in Python2 and Python3 respectively
range() method:
- Because the xrange() method is not supported in Python3, the range() method is used to iterate through for loops.
- The list is returned by this range() method.
- It takes up a lot of memory because it stores the entire list of iterating numbers in memory.
xrange() method:
- The xrange() method is only used in Python version 2 for loop iteration.
- Because it does not generate a static list during runtime, it only returns the generator object.
- It takes up less memory because it only stores one number at a time.
60. How does Python Flask handle requests to databases?
Flask can run database-powered applications (RDBS). A schema must be created for such a system, which requires piping the schema.sql file into the sqlite3 command. To create or start a database in Flask, Python developers must install the sqlite3 command.
Flask allows to request a database in three ways:
- before request: This function is called before a request and receives no arguments.
- after request: These functions are called after a request and pass the response to the client.
- teardown request: This function is invoked when an exception is thrown and responses are not guaranteed. They are called after the response has been built. They are not permitted to make changes to the request, and their values are ignored.
61. What exactly is monkey patching in Python?
Monkey patching is the term used among Python programmers to describe changes made to a class or module during runtime. This is only possible because Python allows for changes in the behavior of the program while it is being executed.
62. How does Python achieve multithreading?
Python has a multi-threading package, but it is not generally considered good practice to use it because it increases code execution time.
- The Global Interpreter Lock (GIL) is available in Python. The GIL ensures that only one of your ‘threads’ can run concurrently. The process ensures that a thread obtains the GIL, performs some work, and then passes the GIL to the next thread.
- This happens in a very short period, so your threads appear to be executed parallel to the human eye, but they are executed one by one by simply taking turns using the same CPU core.
63. Define Dataframes and their syntaxes in pandas.
A data frame in Python pandas is a mutable two-dimensional data structure or data aligned in tabular form with rows and columns.
Syntax :
pandas.DataFrame( data, index, columns, Dtype)
- data: It can take other DataFrames as input and refers to various forms such as ndarray, series, map, lists, dict, and constants.
- index: This argument is optional because the pandas library will handle the index for row labels automatically.
- columns: This argument is optional because the pandas library will handle the index for column labels automatically.
- Dtype: Refers to the data type of each column.
Example:
import pandas as pd
playersData = {
"Ronaldo": [420, 380, 390, 770],
"Messi": [500, 340, 450, 545]
}
#load data into a DataFrame object
df = pd.DataFrame(playersData)
print(df)
Output:
Ronaldo Messi
0 420 500
1 380 340
2 390 450
3 770 545
64. What is SVM?
Support vector machines (SVM) are s set of supervised machine learning algorithms that can be used to solve problems related to classification, regression, and outlier detection. The support vector machine is a representation of the training data in which points in space are separated into categories using a clear gap as wide as possible.
The SVM module is available in the scikit-learn library of Python.
65. In Python, what is the purpose of the map() function?
The map() function applies a function to each iterable item. The results are then returned in a list. The value returned by the map() function can then be passed on to functions such as list() and set().
The given function is typically the first argument to a map() function, and the iterable is available as the second argument. If the function accepts more than one argument, several tables are returned.
Example:
def myFunction(n):
return len(n)
x = map(myFunction, ('hello', 'world', 'hi'))
print(list(x))
Output:
[5, 5, 2]
66. What does the // operator stand for? What is its function?
The // is a Floor Division Operator that divides two operands and displays the result as a quotient with digits before the decimal point. For example, 10//5 equals 2 and 10.0//5.0 equals 2.0.
Example:
print(12//5)
print(12/5)
Output:
2
2.4
67. What are the Python built-in types?
The main built-in types are:
Numeric Types | int , float , complex |
Text Type | str |
Sequence Types | list , tuple , range |
Mapping Type | dict |
Set Types | set , frozenset |
Boolean Type | bool |
Binary Types | bytes , bytearray , memoryview |
None Type | None |
68. In Python, what are pickling and unpickling?
Pickling is the process of converting a Python object hierarchy into a string representation.
Unpickling is the process of retrieving original Python objects from a stored string representation, which is the inverse of pickling.
69. Explain the terms try, raise, and finally.
These are the keywords we use when dealing with exceptions. We put risky code in a try block, use the raise statement to explicitly raise an error, and put code that we want to execute anyway in the finally block.
70. Distinguish between deep and shallow copy.
A deep copy is a copy of one object into another. This means that modifying a copy of an object does not affect the original object. In Python, we use the function deepcopy() from the copy module to accomplish this.
import copy
a = a = [[1, 2, 3], [4, 5, 6]]
b = copy.deepcopy(a)
a[0][0] = 55
print(b[0][0])
Output:
1
A shallow copy, on the other hand, copies the reference to one object to another. As a result, any changes we make to the copy will affect the original object. To implement this, we can use the copy() method from copy module.
import copy
a = a = [[1, 2, 3], [4, 5, 6]]
b = copy.copy(a)
a[0][0] = 55
print(b[0][0])
Output:
55
Note: The difference between deep copy and shallow copy is only relevant when we have to deal with compound objects (nested structures).
71. What exactly is Django?
Django is a web application development framework of Python that supports agile growth and clean pragmatic design. Django allows you to focus on creating your web app without the need of reinventing the wheel. This framework makes web development easy using Python.
72. What are the benefits of using the Django framework?
Django’s main goal in design is to make it simple for its users. To accomplish this, Django employs:
- The principles of rapid development, which imply that developers can work on more than one iteration at a time without having to restart the entire schedule from scratch
- DRY (Do Not Replicate Yourself) philosophy of Django allows developers to reuse the existing code.
- Django is highly scalable.
- Django has a great set of libraries to use. Since Django is powered by Python, you can make use of so many Python libraries.
73. What can the Django framework be used for?
You can use Django to develop web applications from small scale to large scale. You can use it for creating eCommerce websites, content management systems, SaaS apps, social media apps, etc.
74. What is the function of manage.py in Django?
The manage.py is a file that is automatically generated within each Django project. It is a flat wrapper that contains django-admin.py. It has the following applications:
- It adds your project’s package to sys.path.
- It modifies the DJANGO_SETTING_MODULE environment variable to point to the setting.py file in your project.
75. What are the web frameworks of Python?
Python has many frameworks for web development like Django, Flask, FastAPI, Web2Py, etc.
76. Are access specifiers used in Python?
Python does not support access specifiers and does not provide a method to access an instance variable. To mimic the behavior of private and protected access specifiers, Python introduced the concept of prefixing the name of the method, function, or variable with a double or single underscore.
77. What exactly is the Dogpile effect?
This is defined as the occurrence of an event when the cache expires and the websites receive multiple requests from the client at the same time.
A semaphore lock can be used to prevent the dogpile effect.
78. What exactly are virtualenvs?
A virtualenv is a term used by Python developers to describe an isolated environment for developing, running, and debugging Python code.
- It is used to isolate a Python interpreter as well as a collection of libraries and settings.
- Together with pip, it enables the development, deployment, and execution of multiple applications on a single host, each with its own Python interpreter and set of libraries.
79. What is the Python nonlocal statement (in Python 3.0 and later) used for?
- The nonlocal statement causes the listed identifiers to refer to variables that were previously bound in the nearest enclosing scope, excluding globals.
- For example, the counter generator can be rewritten to use this, making it more idiomatic of languages with closures.
- To put it briefly, it enables you to assign values to variables in an external (but non-global) scope.
80. Why isn’t all of the memory released when Python exits?
- When Python exits, objects that have circular references to other objects or objects that are referenced from the global namespaces are not always de-allocated or freed.
- It is not possible to de-allocate memory that has been reserved by the C library.
- Python would try to de-allocate every object on exit because it has its efficient clean-up mechanism.
81. What is the output of the following code?
x = ['ab','cd']
print(list(map(list, x)))
Output:
[['a', 'b'], ['c', 'd']]
The output of the following code is [ [‘a’, ‘b’], [‘c’, ‘d’]
82. Write Python code to sort a numerical list
list = [2,5,7,8,1]
list.sort()
print(list)
Output:
[1, 2, 5, 7, 8]
83. Can you write a code that efficiently counts the number of capital letters in a file?
with open("sample.txt") as countletter:
count = 0
text = countletter.read()
for character in text:
if character.isupper():
count += 1
print(count)
Output:
12
The above code reads a text file in the computer called “sample.txt” and prints the number of upper-case letters as output in the console.
84. How to remove values from a python list?
The pop() and remove() methods can be used to remove elements from a Python list.
The pop() function returns the element that was removed. The remove() function does not return the element that was removed.
Example:
myList = [8.1, 2.4, 6.8, 1.1, 7.7, 1.2, 3.6]
print(myList.pop())
print(myList.pop(3))
myList.remove(8.1)
print(myList)
Output:
3.6
1.1
[2.4, 6.8, 7.7, 1.2]
85. Write a Python program to count the total number of lines in a text file.
with open("sample.txt", 'r') as myFile:
lines = len(myFile.readlines())
print('Total number of lines:', lines)
Output:
Total number of lines: 18
86. How will you send a mail with Python?
The Simple Mail Transfer Protocol (SMTP) is a protocol for transmitting and routing emails between mail servers. The smtplib module in Python creates an SMTP client session object that may be used to send email to any Internet system that has an SMTP or ESMTP listener daemon.
Here is a simple syntax for creating a single SMTP object that can then be used to send an email:
import smtplib
smtpObj = smtplib.SMTP(host, port, local_hostname)
87. What is Pylint?
Pylint is a static code analysis tool that validates your code and enforces coding standards. It also makes refactoring suggestions, such as changing the name of your variable to snake case.
Pylint can be configured both within code and via an external configuration file. It can advise you on conventions (C), refactoring (R), warnings (W), errors (E), and fatal errors (F).
To run it, you can type in the command prompt: pylint palindrome.py
88. Is Python used for web client or web server-side programming?
Python can do both, but it is best suited for web server-side programming because it can implement web server hosting, database interactions, and business logic. It can be used on the client side, but conversions must be implemented so that the client-side logic can be interpreted by the browser.
Generally, developers prefer using Python on the server side and a language like JavaScript on the client side.
Python can also be used to write standalone desktop applications, scripts, and test automation. It is a general-purpose language that excels at many tasks.
89. How will you measure the performance of your code?
We can use the time module to evaluate the performance of our code. We can obtain the time at various stages of execution using time.time() to determine how long a specific section of code takes. We can also log data with timestamps using the logging module.
Code:
import time
count=0
print(time.ctime(time.time()))
for i in range(10000000):
count+=1
print(time.ctime(time.time()))
Output:
Fri Mar 10 16:29:24 2023
Fri Mar 10 16:29:25 2023
time.ctime() will give you the local time.
90. How can you download an image using Python?
We can use the urllib module for this.
Code :
import urllib.request
urllib.request.urlretrieve("https://pythonistaplanet.com/wp-content/uploads/2020/01/Logo-480x240-1-300x150.png", "pythonistaplanet.jpg")
The urlretrieve() function saves a URL to the disc in a temporary location. We give it the image’s URL and what to call it. This code saves the logo as pythonistaplanet.jpg in the current directory.
91. When should you use a generator?
Iterable objects are returned by generators, which we can iterate over with the yield keyword. Generators enable us to pause execution whenever we want.
When dealing with large datasets, we should use generators rather than loops. We can also employ them when we do not require all of the outcomes. They can also be used as callbacks.
92. What is memoization?
Memoization is an optimization technique that involves storing the results of previous function calls to return the cached result when the same input is encountered again. This speeds up the code execution. Decorators can be used to implement this.
93. Write a program to reverse a number in Python.
myNumber = int(input("Enter the number: "))
rev=0
while(myNumber>0):
lastDigit=myNumber%10
rev = rev*10 + lastDigit
myNumber = myNumber//10
print("The reverse of the number:",rev)
Output:
Enter the number: 487
The reverse of the number: 784
94. Write a Python Program to Check if a Number is a Palindrome or not.
myNumber = int(input("Enter the number: "))
number = myNumber
rev=0
while(myNumber>0):
lastDigit=myNumber%10
rev = rev*10 + lastDigit
myNumber = myNumber//10
if(number == rev):
print("The number is a palindrome.")
else:
print("The number isn't a palindrome.")
Output:
Enter the number: 98789
The number is a palindrome.
95. Write a Python Program to count the number of vowels in a String.
myString = input("Enter a string: ")
vowels = 0
for i in myString:
if(i.lower() in ['a','e','i','o','u']):
vowels=vowels+1
print("The number of vowels is:",vowels)
Output:
Enter a string: Hello World
The number of vowels is: 3
96. Write a Python Program to Check if a Number is a Strong Number.
sum = 0
myNumber = int(input("Enter a number: "))
temp = myNumber
while(myNumber):
i = 1
f = 1
r = myNumber%10
while(i<=r):
f = f*i
i = i+1
sum = sum + f
myNumber = myNumber//10
if(sum==temp):
print("The number is a strong number.")
else:
print("The number is not a strong number.")
Output:
Enter a number: 145
The number is a strong number.
97. Write a Python Program to Check if a String is a Palindrome or Not.
myString = input("Enter string:")
if(myString==myString[::-1]):
print("The string is a palindrome.")
else:
print("The string isn't a palindrome.")
Output:
Enter string:bob
The string is a palindrome.
98. Write a Python Program to Check if a Number is a Perfect Number.
n = int(input("Enter any number: "))
sum = 0
for i in range(1, n):
if(n % i == 0):
sum = sum + i
if (sum == n):
print("The number is a perfect number.")
else:
print("The number is not a perfect number.")
Output:
Enter any number: 6
The number is a perfect number.
99. What is Enumerate() Function in Python?
The enumerate() function in Python adds a counter to an iterable object. The enumerate() function accepts sequential indexes beginning with zero.
Code:
subjects = ('Python', 'Interview', 'Questions')
for i,s in enumerate(subjects):
print(i,s)
Output:
0 Python
1 Interview
2 Questions
100. Write a Python Program to Check Common Letters in Two Input Strings?
s1 = input("Enter the first string: ")
s2 = input("Enter the second string: ")
commonLetters = list(set(s1)&set(s2))
print("The common letters are:")
for i in commonLetters:
print(i)
Output:
Enter the first string: Hello
Enter the second string: World
The common letters are:
o
l