When it comes to virtual environments, Python has several options like virtualenv, pipenv, conda, etc., and they do a fantastic job in keeping Python libraries project-specific. Poetry can do the same thing, but also a whole lot more.
Python does not have a single official command-line utility for managing every aspect of a project, unlike some languages like Go and Rust. But third-party projects are available to help. Poetry is a third-party tool that can manage all your Python installations and projects. It is an all-in-one system for managing projects in Python.
Poetry is a Python utility for dependency management, packaging, and publishing. It covers all bases of managing a Python project with ease through its CLI and a single configuration file.
Installation
Before we begin installing poetry, make sure that you already have Python 3 installed in your system. Now let’s see how we can install poetry.
Windows
Open your Powershell and run the following command to install poetry.
(Invoke-WebRequest -Uri https://raw.githubusercontent.com/python-poetry/poetry/master/get-poetry.py -UseBasicParsing).Content | python -

To make the shell functional again, restart it.
Linux/Mac
On Linux and other POSIX-based systems (including mac), run the following command on your terminal.
curl -sSL https://raw.githubusercontent.com/python-poetry/poetry/master/get-poetry.py | python -
Check Version
You can check the version to confirm whether the installation was successful or not. Open a new shell and type the following:
poetry --version
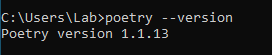
Installation via pip
pip install --user poetry
While you can install poetry with the usual pip command, please note that it will also install poetry’s dependencies which might cause conflicts with other packages.
Updating poetry
You can update poetry to the most recent stable version using the self update command.
poetry self update
Creating a Project in Poetry
Poetry includes a command-line interface (CLI) that allows us to create and set up Python projects. Let’s create a new project.
To create a new project, you can run the following command in your terminal or command prompt.
poetry new myProject
Here, “myProject” is the name of the project that we are creating.

If we check the “myProject” folder, we can see the following structure of files and folders created automatically by poetry.
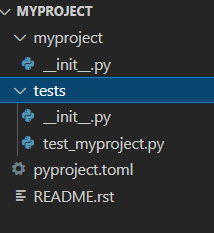
README.rst is a boilerplate readme file you can fill in as you want for your project. There is a subdirectory called “tests” to hold your unit tests. Also, there is a subdirectory with the project’s name, which holds the actual code of the project.
The most important file in this directory is pyproject.toml, which contains all the information we need to manage our package. Everything from our project’s information to dependencies, scripts, etc., is defined here. The pyproject.toml file looks like this.
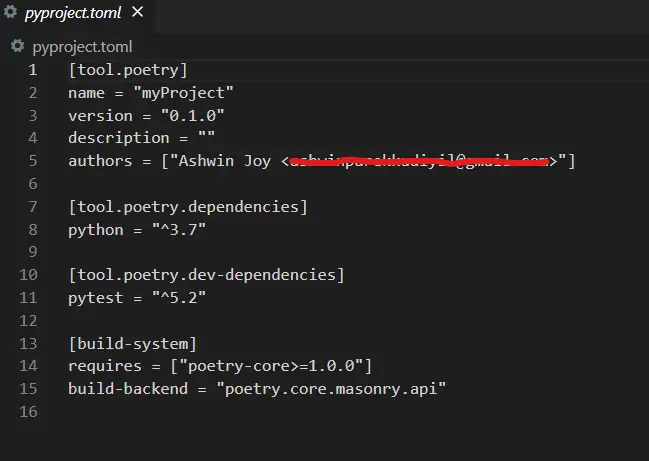
Four sections are defined here in the pyproject.toml file:
- The first part defines all non-dependency package metadata such as name, version, and so on.
- The second part defines all the required project dependencies.
- The third part lists all development requirements that aren’t required to create the project but are required to perform other tasks such as testing, building, and documentation. Contributing developers should obtain dev dependencies in order to iterate on this project.
- The fourth part defines a build system. Unless you upgrade your version of poetry, this section is normally ignored.
Using an existing project with poetry
If you already have one project that you wish to handle using poetry, you can use the init command and interactively create a pyproject.toml file. To do that, you can navigate to your project folder in your terminal and run the following command.
poetry init

You can manually input the required details here.
Poetry CLI
Poetry’s command-line interface is user-friendly. It offers capabilities that allow you to manage multiple package setups.
You may use poetry CLI to:
- Installing and Managing Dependencies
- Configuring your packages
- Executing Applications
- Building and Publishing
Installing and Managing Dependencies
Now let’s see how we can install and manage dependencies using poetry.
Poetry Shell
Poetry comes with a shell command that creates a new shell inside the virtual environment. We will now be able to use the pytest command without having to execute poetry first.
poetry shell

We can exit this shell by typing “exit”.
Adding a Package
Let’s say we want to add a package to our project. For example, let’s use the requests package to execute some API queries. Adding a package with poetry is simple, and it adds a dependency to pyproject.toml.
poetry add requests
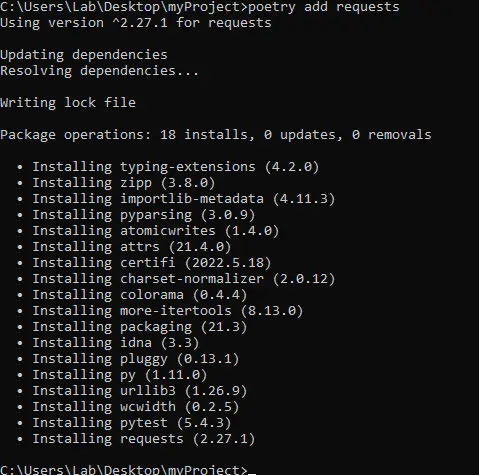
Installing Dependencies
For installing the defined dependencies for your project, you can run the install command. Then, poetry will install the required dependencies mentioned in the pyproject.toml file by itself.
poetry install
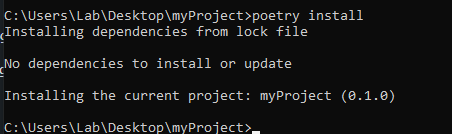
Updating Dependencies
The update command can be used to update all dependencies.
poetry update
If you only want to update a few packages, you can use the update command’s arguments and mention the packages you need to update.
poetry update package1 package2
Removing a Package
If you want to remove a dependency from pyproject.toml, you can use the following command.
poetry remove requests
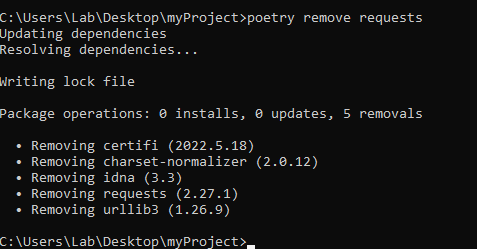
Export to requirements.txt
To export the contents of the .lock file in your project to a requirements.txt file, run the following command.
poetry export -f requirements.txt > requirements.txt

A requirements.txt file will be created in your project folder when you run this command.
Configuring Your Packages
Poetry uses the PyPI repository by default for package installation and publishing. But, if you want to keep your package private with the ability to share it with your teammates, you can use a private repository.
You can easily add a new repository with the config command.
poetry config repositories.example https://examplelink.xyz/example
This will configure the URL for the repository example to https://examplelink.xyz/example.
You can also store your credentials for a specific repository using the config command.
poetry config http-basic.example username password
In the above command, “username” and “password” correspond to your username and password for logging into the private repository. Note that if the password is not specified, you will be required to type it in when you deploy the package.
Error Checking
We can check if there’s an error in pyproject.toml file using the following command.
poetry check
Executing Applications
The following command can be used to execute a script provided in pyproject.toml’s [tool.poetry.scripts] section.
poetry run [script-name]
Building and Publishing
Building the Final Package
We need to add some relevant metadata to distribute and explain how to use our package before we build it, such as the license, some keywords, and the packages we want to include in the final build. We use the build command to create a package.
poetry build
Deployment
After you’ve finished configuring the package, you can simply use the publish command to deploy it.
poetry publish
If you use a private repository, you must specify the name you defined previously through the -r option.
poetry publish -r example
Final Thoughts
In this article, we have seen how to work with the poetry module in Python. I hope this was helpful to you. Now you are ready to use the poetry module to manage your Python projects. Happy coding!