In this post, let’s see how you can find the maximum value or minimum value of an attribute in a list of objects in Pythion.
Let’s say we have a class called “Car” with attributes id (int), name (string), and price (float).
Let’s create a class Car with an __init__ method (constructor) that will accept the attribute values as arguments and use them to initialize the class variables.
class Car:
def __init__(self,id,name,price):
self.id = id
self.name = name
self.price = price
We use “self” as the first argument for every class method. The self keyword is used to point to the current instance of the class (current object).
Let’s create another class CarShop with the attributes carShopName (string) and carList (list of Car objects). We will define the __init__ method for this class as well.
class CarShop:
def __init__(self,carShopName,carList):
self.carShopName = carShopName
self.carList = carList
We need to create two methods inside this class. First of all, let’s create a method inside the Car class for finding out the Car that has the maximum price.
Finding the Maximum Value of an Attribute in a List of Objects in Python
You can do this in two ways. Either you can manually write the code to compare the price values of all the objects and find out the maximum value, or you can use the max() method in Python.
Let’s see how we can do this in both ways.
Option 1: Manually write the code to find the max value
def findCarWithMaximumPrice(self):
if(len(self.carList)==0):
return None
max = self.carList[0].price
maxObject = self.carList[0]
for i in self.carList:
if(i.price>max):
max = i.price
maxObject = i
return maxObject
In the above code, we are checking whether the length of the list is 0 or not. If the list is empty, we will return None, and otherwise, we will continue with the operations.
We can define a max variable and initialize it with the first value. Let’s loop through the list and compare the price of each object with the max variable. If the price of the object is greater than the max variable value, we will set that price as the new max value. We will also keep a copy of the object in a variable (maxObject) so that we can return it.
Option 2: Use the max() method in Python
def findCarWithMaximumPrice(self):
if(len(self.carList)==0):
return None
maxObject = max(self.carList,key=lambda x:x.price)
return maxObject
Here, we can pass the list of objects as the first argument of the max() method. Then, you can follow the syntax key=lambda x:x.attribute_name, where attribute_name is the attribute that we are using to find out the maximum value.
Finding the Minimum Value of an Attribute in a List of Objects in Python
Let’s create the next method to find out the Car that is having the minimum price.
Option 1: Manually write the code to find the min value
def findCarWithMinimumPrice(self):
if(len(self.carList)==0):
return None
min = self.carList[0].price
minObject = self.carList[0]
for i in self.carList:
if(i.price<min):
min = i.price
minObject = i
return minObject
Here, we follow a similar approach as we did in the case of finding the max value. We initialize the min variable with the first value in the list. Then, we can check the price of each object in the list and compare the price value with the min variable value. Finally, we will return the object that has the minimum price.
Option 2: Use the min() method in Python
def findCarWithMinimumPrice(self):
if(len(self.carList)==0):
return None
minObject = min(self.carList,key=lambda x:x.price)
return minObject
Now, let’s write the main program. Create a few Car objects and read the attribute values for each object. For that, we will read the value for n (number of objects that needs to be created) from the user. We will run a for loop for n iterations and create the objects by reading the attribute values for each object. Let’s put the objects created in a list (initialize an empty list and append each object to the list in each iteration). We will pass this list as an argument when we create an object of the CarShop class.
n = int(input("Enter the number of objects: "))
carList = []
for i in range(n):
id = int(input("Enter the id: "))
name = input("Enter the name: ")
price = float(input("Enter the price: "))
carObject = Car(id,name,price)
carList.append(carObject)
carShopObject = CarShop("ABC",carList)
Now, let’s find out the car that has the minimum price and the one that has the maximum price. We can call the methods using the carShopObject and print the details.
Here is the complete program and output:
class Car:
def __init__(self,id,name,price):
self.id = id
self.name = name
self.price = price
class CarShop:
def __init__(self,carShopName,carList):
self.carShopName = carShopName
self.carList = carList
def findCarWithMaximumPrice(self):
if(len(self.carList)==0):
return None
maxObject = max(self.carList,key=lambda x:x.price)
return maxObject
def findCarWithMinimumPrice(self):
if(len(self.carList)==0):
return None
minObject = min(self.carList,key=lambda x:x.price)
return minObject
n = int(input("Enter the number of objects: "))
carList = []
for i in range(n):
id = int(input("Enter the id: "))
name = input("Enter the name: ")
price = float(input("Enter the price: "))
carObject = Car(id,name,price)
carList.append(carObject)
carShopObject = CarShop("ABC",carList)
result1 = carShopObject.findCarWithMaximumPrice()
print("---Printing the Car with maximum price---")
print(result1.id)
print(result1.name)
print(result1.price)
result2 = carShopObject.findCarWithMinimumPrice()
print("---Printing the Car with minimum price---")
print(result2.id)
print(result2.name)
print(result2.price)
Output:
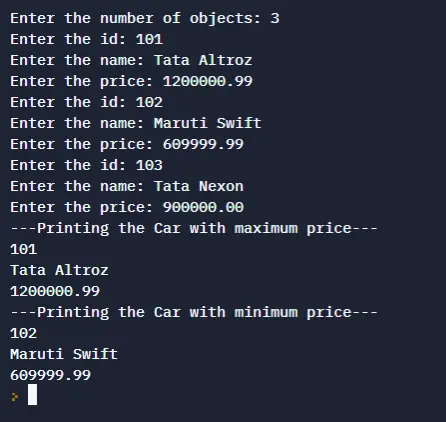