Fibonacci series is an important problem in the field of computer science. Also, it is one of the most frequently asked problems in programming interviews and exams.
Fibonacci is a special kind of series in which the current term is the sum of the previous two terms. It’s like 0, 1, 1, 2, 3, 5, 8, 13,…. and so on.
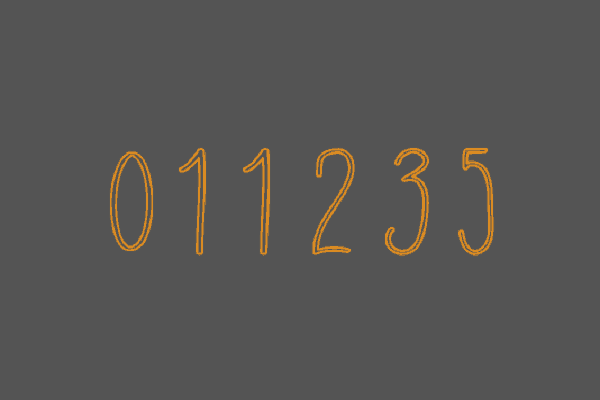
There are many ways to solve this problem.
The primitive recursive solution takes a huge amount of time because for each number calculated, it needs to calculate all the previous numbers more than once.
This gets worse and worse the higher the number you want to compute.
The major problem of this approach is that with each Fibonacci number we calculate in our list, we don’t use the previous numbers we have knowledge of to make the computation faster.
Instead, we compute each number from scratch.
In python, you can write the solution to this problem very easily, to avoid all these complexities.
Let’s look at how can we write the fastest solution to the Fibonacci sequence.
Iterative Solution to find Fibonacci Sequence
In Python, we can solve the Fibonacci sequence in both recursive as well as iterative ways, but the iterative way is the best and easiest way to do it.
The source code of the Python Program to find the Fibonacci series without using recursion is given below.
a = 0
b = 1
n=int(input("Enter the number of terms in the sequence: "))
print(a,b,end=" ")
while(n-2):
c=a+b
a,b = b,c
print(c,end=" ")
n=n-1
The user must enter the number of terms to be printed in the Fibonacci sequence. We use a while loop to find the sum of the first two terms and proceed with the series by interchanging the variables.
We decrement the value of n and print the Fibonacci series till n-2 is greater than 0. Note that the program uses assignment and swapping of values in a single line.
Python language has the built-in capability to do this to reduce your coding efforts. This is why we love Python. It is so easy to code when you compare it with any other programming language.
You can just copy and paste this code and run it on your python IDLE. You will get an output like the one that is given below.
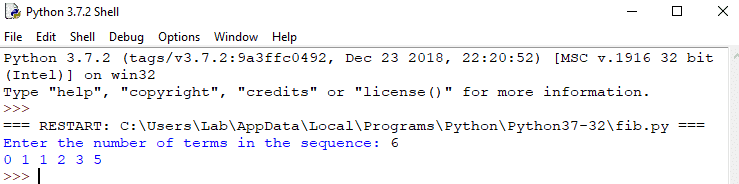
Iterative Solution to find the nth term in Fibonacci Sequence
So, if you want to find the nth term in this series, you can do this in a few lines of code as follows.
def fibonacci(n):
a,b = 0,1
for i in range(n):
a,b = b,a+b
return a
print(fibonacci(6))
You can see how simple and beautiful the code is written in this method.
The main part of the code is at line no.4. Here, the program uses assignments and swapping of values in just a single line.
Please note that this method is efficient in predicting the nth term of the Fibonacci sequence.
If your goal is to create a list of Fibonacci numbers, then this method is not recommended.
That’s it. This code is going to give back 8 which is exactly the 6th term in the series. You can put any position instead of 6. The code will generate the corresponding value as the output.
This is a very simple solution and this is the right way you should write the solution when you are at a job interview or test.
If you could not understand the logic, just go through the code once again. I’m sure that once you get that kick in your brain, this supercool Python trick will be helpful in your programming journey.
If you have any doubts or suggestions, feel free to let me know in the comments section.
Also, do share this article if it was helpful for you.
Hi Ashwin. How do I update your program below to output the sum of first n terms in the Fibonacci sequence? Thanks!
def fibonacci(n):
a,b = 0,1
for i in range(n):
a,b = b,a+b
return a
Hi Lottie, Here is the Python code to find the sum of the first n terms of the Fibonacci sequence-
sum = 1
a = 0
b = 1
n=int(input("Enter the number of terms in the sequence: "))
print(a,b,end=" ")
while(n-2):
----c=a+b
----a,b = b,c
----sum = sum + c
----print(c,end=" ")
----n=n-1
print("\nThe sum of n terms is: ",sum)
You can put spaces instead of the “-” symbols.
n=int(input(“Enter the input number:”))
x=0
y=1
z=0
while(z<=n):
print(z)
x=y
y=z
z=x+y