Method overloading and method overriding are two important terms in object-oriented programming. You might find these two terms confusing, but it won’t be anymore. In this article, let’s understand the differences between method overloading and method overriding.
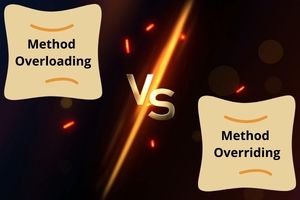
What is the difference between method overloading and method overriding? Method overloading allows multiple methods in the same class to have the same name but different parameters. Method overriding allows a parent class and a child class to have methods with the same name and same parameters. The child class method will override the parent class method.
Below is a table that points out the differences between method overloading and method overriding.
Method Overloading | Method Overriding |
---|---|
A method with the same name but different number or type of arguments. | A method with the same name and the same number and type of arguments. |
Inheritance is optional | Inheritance is required. |
Takes place within a single class | Methods reside in parent and child classes |
Can be done within a class | At least 2 classes are required |
The binding of overloaded methods is done at compile-time; hence it is part of compile-time polymorphism. | The binding of overridden methods is done at run-time; hence it is part of run-time polymorphism. |
Increases code flexibility and readability by providing multiple ways to perform a single operation. | Used in the implementation of specific scenarios and allows a child class to provide a specific implementation of a method already provided by its parent class. |
Relates with Polymorphism | Relates with Inheritance |
Now let’s dive deep into both overloading and overriding and understand the concepts clearly with some simple examples.
Method Overloading
To understand method overloading and overriding, you must know the concept of polymorphism. Poly means many. Morphism means forms. So, polymorphism means “many forms”. In programming terms, it means that there can be many functions with the same name but different forms, arguments, or operations.
An Example of Polymorphism and Method Overloading in Java:
class OverloadingExample {
// Method with 2 parameters
public void add(int a, int b) {
System.out.println(a+b);
}
// Method with 3 parameters
public void add(int a, int b, int c) {
System.out.println(a+b+c);
}
}
public class Main {
public static void main(String[] args) {
OverloadingExample obj = new OverloadingExample();
obj.add(5,10); // Output: 15
obj.add(5,10,20); // Output: 35
}
}
In the example above, we define a class OverloadingExample
with two methods named add
. One method takes two integers as parameters, the other takes three. When we call add(5,10) on the instance obj
of OverloadingExample
, it prints the sum of two integers. But when we call add(5,10,20), it prints the sum of three integers.
This is an example of method overloading. The add
method is overloaded with two different parameter lists. The appropriate add
method to call is determined at compile time based on the number and type of arguments.
It is important to note that Python, the primary language discussed on this site, does not support method overloading natively. This is because Python methods are allowed to have variable argument lists, so the need for method overloading is not as great. If you try to define two methods with the same name but different parameters in Python, it will simply consider the last method definition and ignore the previous ones. However, you can achieve similar functionality in Python using optional arguments or a variable number of arguments.
Method Overriding
In method overriding, we will have many methods, and this time the number of arguments will also be the same.
Now you must be wondering how these methods are different from each other if their name and signatures are the same. So, the answer is that they differ in location. Methods will be placed in different classes.
class ClassOne:
def display(self):
print("Hello from ClassOne")
class ClassTwo:
def display(self):
print("Hello from ClassTwo")
c1 = ClassOne()
c2 = ClassTwo()
c1.display()
c2.display()
Output:
Hello from ClassOne Hello from ClassTwo
Now let’s connect both classes using Inheritance.
In Inheritance, as the name suggests, one class inherits the properties of another class. Let’s understand inheritance with the help of a real-world example:
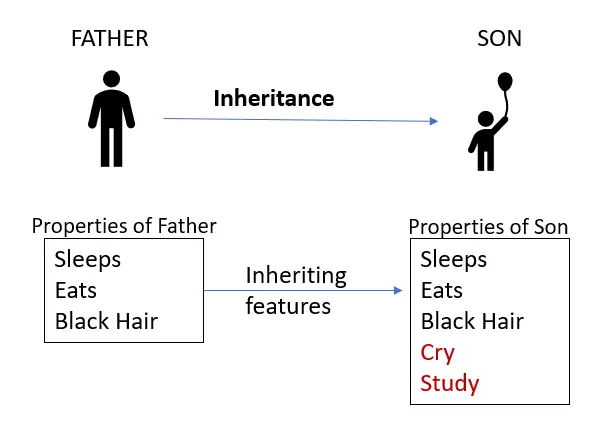
Son inherits all the features from his Father but has his unique features too. Here, the Father is the parent class (also called base class, super class, etc.), and Son is the child class (also called derived class).
class Father:
def sleep(self):
print("Sleep")
def eat(self):
print("Eat")
def blackHair(self):
print("Black Hair")
class Son(Father):
def cry(self):
print("Cry")
def study(self):
print("Study")
sonObject = Son()
sonObject.sleep()
sonObject.eat()
sonObject.blackHair()
sonObject.cry()
sonObject.study()
Output:
Sleep Eat Black Hair Cry Study
You can see that the object of the Son class can access the methods of the Father class, along with accessing its own methods.
Now that we know how inheritance works, let’s see what happens when we do method overriding.
class ClassOne:
def display(self):
print("Hello from ClassOne")
class ClassTwo(ClassOne):
def display(self):
print("Hello from ClassTwo")
c2 = ClassTwo()
c2.display()
Output:
Hello from ClassTwo
Here, ClassTwo inherits ClassOne. Hence, the objects of ClassTwo should be able to access the methods of ClassOne. But since the method names are the same in both classes, the method in the child class overrides the method in the parent class.
So, if you want to access overridden methods inside the child class, you need to use the super() method. The super() function in Python is a built-in function that is used to call a method from a parent class in a child class.
class ClassOne:
def display(self):
print("Hello from ClassOne")
class ClassTwo(ClassOne):
def display(self):
print("Hello from ClassTwo")
super().display()
c2 = ClassTwo()
c2.display()
Output:
Hello from ClassTwo Hello from ClassOne
Final Thoughts
In this article, we have seen the fundamental concepts of method overloading and method overriding along with example Python programs.
I hope these concepts are clear to you. Now you can implement these concepts while you do object-oriented programming.
Check out this article if you want to understand the differences between method overloading and operator overloading.
Happy coding!