What is .NET?
.NET is an open-source development platform created by Microsoft for building different types of apps. A developer platform contains programming languages and libraries. C#, F#, and Visual Basic are the languages available within .NET.
.NET has the following platforms for developing apps:
.NET Framework | Websites, services, and apps on Windows. |
.NET Core | Runs anywhere (Windows, macOS, and Linux) |
Xamarin/Mono | .NET for mobile |
What is C#?
C# (pronounced “See Sharp”) is a modern programming language that helps you to create many types of applications that run in .NET. C# is an object-oriented language and has its roots in the C family of languages.
Working of C#
The C# code you write is converted into an intermediate language code (IL code) using the compiler. This IL code is platform-independent. This code is then run on CLR (Common Language Runtime). The CLR performs a Just-In-Time (JIT) compilation to convert the IL code to native machine code.
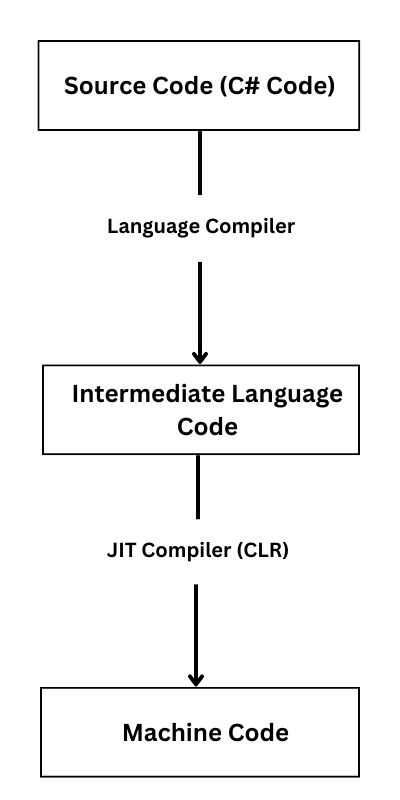
Let’s start writing C# code with a simple “Hello World” program.
Hello World Program in C#
using System;
class Hello
{
static void Main()
{
Console.WriteLine("Hello World");
}
}
Output:
Hello World
We start with the “using” keyword that references a namespace called System. The System namespace contains a number of types such as Console class, IO, Collections, and many more.
We create a class to write the code since C# is an object-oriented programming language. We create a static method inside the class called Main which serves as the entry point of a C# program.
Data Types and Variables
C# is a statically-typed language, which means when you create a variable, you have to mention the data type along with it.
A variable is a name you give to computer memory locations that are used to store values in a computer program. We can assign values of different data types to variables.
The most commonly used data types in C# are int, float, double, char, string, bool, etc.
using System;
public class MainClass
{
public static void Main(string[] args)
{
int myNumber = 25; // Integer value (whole number)
float myFloatNumber = 5.33F; // Floating point number
double myDoubleNumber = 9.99D; // Double
char myLetter = 'A'; // Character
bool myBool = true; // Boolean
string myString = "Hello World"; // String
Console.WriteLine(myNumber);
Console.WriteLine(myFloatNumber);
Console.WriteLine(myDoubleNumber);
Console.WriteLine(myLetter);
Console.WriteLine(myBool);
Console.WriteLine(myString);
}
}
Output:
25
5.33
9.99
A
True
Hello World
Note: The difference between float and double is the precision of values. The precision of a float
variable is only 6-7 decimal digits, while a double
variable has a precision of about 15 digits. Hence, it is safer to use double
for most calculations.
Comments in C#
A comment is a programmer-readable line of code that makes the source code easier for humans to understand. Comments are ignored by the compiler.
In C#, we use // for single-line comments. Multi-line comments in C# start with /* and end with */.
using System;
public class MainClass
{
public static void Main(string[] args)
{
// This is a single-line comment.
/* This is a
multi-line comment */
Console.WriteLine("Hello World");
}
}
Output:
Hello World
Reading User Input in C#
We can use Console.ReadLine() to read user input in C#.
using System;
public class MainClass
{
public static void Main(string[] args)
{
Console.WriteLine("Enter your name: ");
string userName = Console.ReadLine();
Console.WriteLine("Your name is: " + userName);
}
}
Output:
Enter your name:
Ashwin
Your name is: Ashwin
By default, the Console.ReadLine()
method returns a string
. If you want to read data and save it as other data types, you need to explicitly convert the data.
In C#, you can convert any data type explicitly, by using one of the Convert.To
methods.
using System;
public class MainClass
{
public static void Main(string[] args)
{
Console.WriteLine("Enter a number: ");
int myNumber = Convert.ToInt32(Console.ReadLine());
Console.WriteLine("The number is: " + myNumber);
}
}
Output:
Enter a number:
57
The number is: 57
Some other examples of Convert.To methods are: ToInt64, ToDouble, ToBoolean, and ToString.
You can also convert data into another type using datatype.Parse() method.
using System;
public class MainClass
{
public static void Main(string[] args)
{
Console.WriteLine("Enter a number: ");
int myNumber = int.Parse(Console.ReadLine());
Console.WriteLine("The number is: " + myNumber);
}
}
Output:
Enter a number:
53
The number is: 53
C# Operators
We have various types of operators available in C#. The following are the most commonly-used operators in C# language.
Type of Operator in C# | Operators |
---|---|
Arithmetic Operators | + (addition) – (subtraction) * (multiplication) / (division) % (modulus) |
Assignment Operators | = (equals) += (x += 1 is the same as x = x+1) -= (x -= 1 is the same as x = x-1) *= (x *= 1 is the same as x = x*1) /= (x /= 1 is the same as x = x/1) |
Increment/Decrement Operators | ++ (increment) — (decrement) |
Comparison Operators | == (checking equality) != (checking non-equality) < (less than) <= (less than or equal to) > (greater than) >= (greater than or equal to) |
Logical Operators | && (logical AND) || (logical OR) ! (logical NOT) |
Arithmetic Operators
using System;
class MainClass {
static void Main() {
int firstNumber = 6;
int secondNumber = 4;
Console.WriteLine(firstNumber+secondNumber);
Console.WriteLine(firstNumber-secondNumber);
Console.WriteLine(firstNumber*secondNumber);
Console.WriteLine(firstNumber/secondNumber);
Console.WriteLine(firstNumber%secondNumber);
}
}
Output
10
2
24
1
2
Assignment Operators
using System;
class MainClass {
static void Main() {
int myNumber = 3;
myNumber += 2;
Console.WriteLine(myNumber);
myNumber -= 1;
Console.WriteLine(myNumber);
myNumber *= 2;
Console.WriteLine(myNumber);
}
}
Output:
5
4
8
Increment/Decrement Operators
using System;
class MainClass {
static void Main() {
int firstNumber = 6;
int secondNumber = 4;
firstNumber++;
secondNumber--;
Console.WriteLine(firstNumber);
Console.WriteLine(secondNumber);
}
}
Output:
7
3
Comparison Operators
using System;
class MainClass {
static void Main() {
int firstNumber = 6;
int secondNumber = 4;
Console.WriteLine(firstNumber<secondNumber);
Console.WriteLine(firstNumber>secondNumber);
Console.WriteLine(firstNumber<=secondNumber);
Console.WriteLine(firstNumber>=secondNumber);
Console.WriteLine(firstNumber==secondNumber);
Console.WriteLine(firstNumber!=secondNumber);
}
}
Output:
False
True
False
True
False
True
Logical Operators
using System;
class MainClass {
static void Main() {
Console.WriteLine(3==3 && 4==4);
Console.WriteLine(3==3 && 5==4);
Console.WriteLine(3==3 || 5==4);
Console.WriteLine(3==4 || 5==4);
Console.WriteLine(!(3==3 && 4==4));
}
}
Output:
True
False
True
False
False
Conditional Statements
Conditional statements are used to check conditions and take logical decisions based on the conditions. In C#, we use if-else statements and switch-case statements to perform conditional logic.
If-Else Statements
using System;
class MainClass {
static void Main() {
int firstNumber = 6;
int secondNumber = 4;
if(firstNumber>secondNumber){
Console.WriteLine("1st number is greater than 2nd.");
}else if(firstNumber<secondNumber){
Console.WriteLine("2nd number is greater than 1st.");
}else{
Console.WriteLine("Both numbers are equal.");
}
}
}
Output:
1st number is greater than 2nd.
Switch-Case Statements
using System;
class MainClass {
static void Main() {
int myNumber = 2;
switch(myNumber)
{
case 1:
Console.WriteLine("The number is 1.");
break;
case 2:
Console.WriteLine("The number is 2.");
break;
case 3:
Console.WriteLine("The number is 3.");
break;
default:
Console.WriteLine("The number is unknown.");
break;
}
}
}
Output:
The number is 2.
Loops in C#
Loops in C# allow us to execute a set of code instructions multiple times. This avoids repetition of the same code and thereby reduces the effort of the programmer.
In C#, we have different types of loops, such as, while loop, do-while loop, for loop, foreach loop, etc.
While Loop
using System;
class MainClass {
static void Main() {
int myNumber = 0;
while(myNumber < 5)
{
Console.WriteLine(myNumber);
myNumber++;
}
}
}
Output:
0
1
2
3
4
Do-While Loop
In a do-while loop, the loop will run at least once, even if the condition is false. The loop starts execution for the first iteration and checks the condition only after the first iteration is completed. If the condition is true, loop will continue with the next iteration, else it will stop.
using System;
class MainClass {
static void Main() {
int myNumber = 0;
do{
Console.WriteLine(myNumber);
myNumber++;
} while(myNumber < 5);
}
}
Output:
0
1
2
3
4
For Loop
For loops are preferred when we have to iterate through a group of values in C#.
using System;
class MainClass {
static void Main() {
for(int i = 0; i < 10; i++){
Console.WriteLine(i);
}
}
}
Output:
0
1
2
3
4
5
6
7
8
9
For-Each Loop
The foreach loop is another type of loop available in C#. We use it mostly when we have to iterate through an array.
using System;
class MainClass {
static void Main() {
int[] myNumbers = {1,5,7,9};
foreach(int i in myNumbers)
{
Console.WriteLine(i);
}
}
}
Output:
1
5
7
9
Break Statement in Loops
The break statement is used to terminate the loop. If the code encounters a break statement, the loop will terminate there.
using System;
class MainClass {
static void Main() {
for(int i = 0; i < 10; i++){
if(i==4){
break;
}
Console.WriteLine(i);
}
}
}
Output:
0
1
2
3
Continue Statement in Loops
The continue statement is used to skip the current iteration of the loop. If the code encounters a continue statement, the loop will skip the rest of the current iteration and go to the next iteration.
using System;
class MainClass {
static void Main() {
for(int i = 0; i < 10; i++){
if(i==4){
continue;
}
Console.WriteLine(i);
}
}
}
Output:
0
1
2
3
5
6
7
8
9
Arrays in C#
Arrays are used to store a group of values of the same data type in contiguous memory locations.
using System;
using System.Linq;
class MainClass {
static void Main() {
// Defining an int array.
int[] numbers = new int[10];
numbers[0] = 11;
numbers[1] = 22;
numbers[2] = 33;
// Defining an int array with values.
int[] myNumbers = {5, 10, 20, 30, 40, 50};
// Defining a string array with values.
string[] players = {"Ronaldo","Messi","Neymar"};
// Accessing values in an array using index.
Console.WriteLine(myNumbers[0]);
Console.WriteLine(players[2]);
// Finding the length of an array.
Console.WriteLine(myNumbers.Length);
Console.WriteLine(players.Length);
// Adding a value to an array (another way).
numbers.SetValue(value: 44, index: 3);
Console.WriteLine(numbers[3]);
// Looping through an array.
for(int i = 0; i < players.Length; i++){
Console.WriteLine(players[i]);
}
// Looping through an array using a for-each loop.
foreach (int i in myNumbers){
Console.WriteLine(i);
}
// Sorting an array.
Array.Sort(players);
for(int i = 0; i < players.Length; i++){
Console.WriteLine(players[i]);
}
// Finding max, min, and sum using System.Linq (write the "using" line at the top).
Console.WriteLine(myNumbers.Max());
Console.WriteLine(myNumbers.Min());
Console.WriteLine(myNumbers.Sum());
}
}
Output:
5
Neymar
6
3
44
Ronaldo
Messi
Neymar
5
10
20
30
40
50
Messi
Neymar
Ronaldo
50
5
155
Multi-Dimensional Arrays in C#
The single comma inside the square brackets [,]
specifies that the array is 2-dimensional. A 3-dimensional array has two commas: int[,,]
.
using System;
class MainClass {
static void Main() {
// Defining a 2-D Array.
int[,] numbers = { {1, 3, 5}, {7, 9, 11} };
Console.WriteLine(numbers[1,2]);
// Defining a 3-D Array.
int[,,] myNumbers = { {{1,2}, {3,4}}, {{5,6}, {7,8}} };
Console.WriteLine(myNumbers[1,1,1]);
}
}
Output:
11
8
Methods in C#
A method in C# is a block of code that you can create and reuse anytime. You can give a name to your method and that block of code will be executed only if it is called.
There are many in-built methods in C#. For example, Console.WriteLine() and Console.ReadLine() are in-built methods available in C#.
Programmers can also create their own methods. A method can take data as input (called method parameters) and it can return data as output. When you create a method, you should define the return type (the data type that the method returns) before the method name. If the method doesn’t return anything, you can put “void” as the return type.
We can create any number of methods inside a class. This method can be called by the object of the class.
So, let’s understand what classes and objects are. Then we will see an example.
Classes and Objects
C# is an object-oriented programming (OOP) language. OOP is a programming paradigm in which every entity is treated as an object. A class is a blueprint of objects.
For example, let’s say a company creates thousands of cars of the same model. In this case, all these individual cars can be called objects and a common design used for building all these cars can be called a class. You can create any number of objects from a class, and these objects will get all the properties of a class.
Here is an example of creating classes and objects in C#. Let’s create a Customer class for a Bank. This class will have name, id, and balance as the variable attributes. This class will also have some methods to check balance, deposit money, and withdraw money.
using System;
class Customer{
public string name;
public int id;
public double balance;
public double fetchBalance(){
return balance;
}
public void depositAmount(double amount){
balance += amount;
}
public bool withdrawAmount(double amount){
double remanining = balance - amount;
if(remanining<500){
return false;
}else{
balance -= amount;
return true;
}
}
}
public class MainClass
{
public static void Main(string[] args)
{
Customer ashwin = new Customer();
ashwin.name = "Ashwin";
ashwin.id = 101;
ashwin.balance = 2000;
Console.WriteLine(ashwin.fetchBalance());
ashwin.depositAmount(5500);
Console.WriteLine(ashwin.fetchBalance());
ashwin.withdrawAmount(500);
Console.WriteLine(ashwin.fetchBalance());
}
}
Output:
2000
7500
7000
This is not the recommended way since the data is public (data is accessible even outside the class). It is better to keep data private (so that the data can be accessed only inside the class). The private and public keywords are called access specifiers.
You can write the code in a way that any changes to the data can be done only using methods of the class. By default, the data members in a C# class are private.
Properties in C#
You can create properties to create get and set methods. A property will allow you to access and update the value of a private attribute. This process is called the encapsulation of data.
A property is a combination of a variable and a method. It has two methods: a get
method and a set
method.
using System;
class Customer{
string name;
// Creating a property to get and set values.
public string pName{
get{
return name;
}
set{
name = value;
}
}
}
public class MainClass
{
public static void Main(string[] args)
{
Customer ashwin = new Customer();
ashwin.pName = "Ashwin";
Console.WriteLine(ashwin.pName);
}
}
Output:
Ashwin
You can also create getters and setters with shorthand properties. Here, you can create a property as public. The data member will be automatically created by the compiler and it will be hidden.
using System;
class Customer{
// Creating a public property with get & set.
public string pName {get; set;}
}
public class MainClass
{
public static void Main(string[] args)
{
Customer ashwin = new Customer();
ashwin.pName = "Ashwin";
Console.WriteLine(ashwin.pName);
}
}
Output:
Ashwin
Constructors in C#
A constructor is a method that gets automatically invoked whenever you create an object of a class. You can create a constructor of a class by creating a method with the name same as that of the class name. A constructor allows the programmer to give default values to the class attributes.
using System;
class Customer{
// Creating a public property with get & set.
public string pName {get; set;}
public Customer(){
pName = "Bob";
}
}
public class MainClass
{
public static void Main(string[] args)
{
Customer ashwin = new Customer();
Console.WriteLine(ashwin.pName);
}
}
Output:
Bob
You can also create parameterized constructors. This will allow us to pass values when we create the object and these values can be assigned to the data members of the class.
using System;
class Customer{
// Creating a public property with get & set.
public string pName {get; set;}
public Customer(string customerName){
pName = customerName;
}
}
public class MainClass
{
public static void Main(string[] args)
{
Customer ashwin = new Customer("Ashwin Joy");
Console.WriteLine(ashwin.pName);
}
}
Output:
Ashwin Joy
If the parameter name and the data member name are the same, you can use the “this” keyword to point to the data member of the current object. Using the “this” keyword will avoid confusion if both names are the same.
using System;
class Customer{
// Creating a public property with get & set.
public string pName {get; set;}
public Customer(string pName){
this.pName = pName;
}
}
public class MainClass
{
public static void Main(string[] args)
{
Customer ashwin = new Customer("Ashwin Joy");
Console.WriteLine(ashwin.pName);
}
}
Output:
Ashwin Joy
Inheritance in C#
We can inherit all the properties of one class to another in C#. The class that inherits from another class is called the subclass or the child class or the derived class. The class being inherited from is called the parent class or the base class or the superclass.
The child class will get all the data members(attributes and methods) of the parent class.
To inherit from a class in C#, use the :
symbol.
Let’s see an example.
using System;
class Animal
{
public string name = "Animal";
public void makeSound()
{
Console.WriteLine("Animal Sound!");
}
}
class Cat : Animal
{
public string animalName = "Cat";
}
class Program
{
static void Main(string[] args)
{
Cat myCat = new Cat();
myCat.makeSound();
Console.WriteLine(myCat.name);
Console.WriteLine(myCat.animalName);
}
}
Output:
Animal Sound!
Animal
Cat
You can see that the object of the child class Cat is able to access the attributes and methods of its own class and its parent class.
Note: If you want to restrict other classes to inherit from a class, you can use the sealed
keyword.
sealed class Animal
{
...
}
Polymorphism in C#
In C#, polymorphism allows coders to perform a single action in different ways. Multiple functions can have the same name. The same function call can get responses in different ways.
There are two types of polymorphism: compile-time polymorphism and run-time polymorphism.
Run-time Polymorphism
Run-time polymorphism can be achieved using method overriding in C#. In method overriding, parent classes can define and implement virtual methods, and child classes can override them. The overridden methods in child classes provide their own definition and implementation.
During run-time, when the code calls the method, the CLR looks up the run-time type of the object. It then invokes the override of the virtual method.
Let’s look at an example where Animal is the base class and Cat and Dog are child classes. The makeSound() method is defined as virtual in the parent class. The Cat and Dog class overrides this method in their respective classes.
using System;
class Animal
{
public virtual void makeSound()
{
Console.WriteLine("Animal Sound!");
}
}
class Cat : Animal
{
public override void makeSound()
{
Console.WriteLine("Meow, Meow!");
}
}
class Dog : Animal
{
public override void makeSound()
{
Console.WriteLine("Boww, Boww!");
}
}
class Program
{
static void Main(string[] args)
{
Animal myAnimal = new Animal();
Cat myCat = new Cat();
Dog myDog = new Dog();
myAnimal.makeSound();
myCat.makeSound();
myDog.makeSound();
}
}
Output:
Animal Sound!
Meow, Meow!
Boww, Boww!
Note: A child class can override a parent class member only if the parent class member is declared as virtual or abstract.
Compile-time Polymorphism
Compile-time polymorphism can be achieved by method overloading in C#. In method overloading, there are multiple methods with the same name but different parameters. The data type of the parameters could be different from each other or the number of parameters could be different.
Let’s look at an example of method overloading in C#.
using System;
class Addition {
public int addNumbers(int a, int b){
return a + b;
}
public int addNumbers(int a, int b, int c){
return a + b + c;
}
public double addNumbers(double a, double b, double c){
return a + b + c;
}
}
class Program
{
static void Main(string[] args)
{
Addition a = new Addition();
Console.WriteLine(a.addNumbers(5, 3));
Console.WriteLine(a.addNumbers(6, 9, 2));
Console.WriteLine(a.addNumbers(7.32, 4.19, 3.14));
}
}
Output:
8
17
14.65
Collections in C#
Collections in C# allow us to store, manage, and manipulate data in an efficient way. There are two types of collections in C#: generic and non-generic.
Here are some examples of generic and non-generic collections in C#.
Generic Collections | Non-generic Collections |
---|---|
List | ArrayList |
Dictionary | HashTable |
SortedList | SortedList |
Stack | Stack |
Queue | Queue |
Generic collections are strongly typed (you can store only objects of the same type). This eliminates runtime type mismatches. Non-generic collections are not so strongly typed and are more dynamic. You can add data of different types in non-generic collections.
For example, in a List, you can only add data of the same type, whereas, in an ArrayList, you can add data of multiple types.
We prefer using generic collections in most cases because they perform faster than non-generic collections and also reduce exceptions by giving compile-time errors.
Lists and dictionaries are the most commonly used collections in C#.
Lists in C#
A List class is used to create a collection of data of the same type (integers, strings, etc.). A list is similar to an array, but we can resize a list dynamically, which we can’t do with an array.
using System;
using System.Collections.Generic;
class HelloWorld {
static void Main() {
List<int> myList = new List<int>();
// Adding and removing values.
myList.Add(20);
myList.Add(40);
myList.Add(30);
myList.Add(10);
myList.Remove(40);
// Finding get the length of the list
Console.WriteLine(myList.Count);
// Printing the elements of the list.
foreach (int i in myList)
{
Console.Write(i+ " ");
}
Console.WriteLine();
// For sorting the list.
myList.Sort();
// For reversing the list.
myList.Reverse();
foreach (int i in myList)
{
Console.Write(i+ " ");
}
}
}
Output:
3
20 30 10
30 20 10
There are many other in-built methods available for lists. We can use those methods for managing and manipulating data inside a list.
Dictionaries in C#
In C#, we use dictionaries to store key-value pairs of data.
using System;
using System.Collections.Generic;
class HelloWorld {
static void Main() {
Dictionary<string, int> contacts = new Dictionary<string, int>();
// Adding data to the dictionary
contacts.Add("Bob", 56566543);
contacts.Add("John", 98767525);
contacts.Add("Kiara", 13413325);
// Updating data in a dictionary.
contacts["John"] = 18009892;
// Removing data from the dictionary
contacts.Remove("Kiara");
// Accessing a value using a key.
Console.WriteLine(contacts["Bob"]);
// Printing the data in a dictionary
foreach(var kvp in contacts)
{
Console.Write(kvp.Key + " " + kvp.Value);
Console.WriteLine();
}
}
}
Output:
56566543
Bob 56566543
John 18009892
Dictionaries also provide several in-built methods to make the job easy for programmers.
Final Thoughts
C# is a language that has a lot more features. This post is a simplified version of the most important topics in C# covering the fundamentals of C#. To explore more, check out the official documentation of C# created by Microsoft.
Once you are comfortable with C#, you can check out the tutorials on MS SQL Server and ADO.NET. These technologies will help you to connect your C# code to a database.
I hope this tutorial was helpful. Happy coding!