Hello World Program
#include <stdio.h>
int main() {
printf("Hello World \n");
return 0;
}
Output:

You can use int main() and void main to write the main() method in C. Here, void main() denotes that the main() function will not return any value, whereas int main() indicates that the main() function can return an integer type value.
It is good practice to use int main() over the void main() as it will terminate the execution of the main() method when it finds the return value which can be zero or a non-zero value.
Variables and Data Types
#include <stdio.h>
int main() {
int myInteger;
float myFloat;
double myDouble;
char myChar;
char myString[10];
printf("Enter an integer value: ");
scanf("%d",&myInteger);
printf("Enter a float value: ");
scanf("%f",&myFloat);
printf("Enter a double value: ");
scanf("%lf",&myDouble);
printf("Enter a character: ");
scanf(" %c",&myChar);
printf("Enter a string: ");
scanf("%s",myString);
printf("\n");
printf("%d \n%f \n%lf \n",myInteger,myFloat,myDouble);
printf("%c \n%s \n",myChar,myString);
return 0;
}
Output:
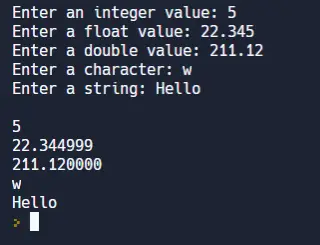
Operators
#include <stdio.h>
int main() {
int a = 12;
int b = 5;
// Arithmetic Operators.
printf("Addition: %d \n",a+b);
printf("Subtraction %d \n",a-b);
printf("Multiplication: %d \n",a*b);
printf("Division: %d \n",a/b);
printf("Modulus(Remainder): %d \n\n",a%b);
// Unary Operators
// a gets incremented after printing it
printf("Increment: %d \n",a++);
// a gets incremented before printing it
printf("Increment: %d \n",++a);
// a gets decremented after printing it
printf("Decrement: %d \n",a--);
// a gets decremented before printing it
printf("Decrement: %d \n",--a);
return 0;
}
Output:
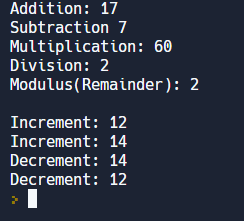
Conditional Statements
if-else statements
#include <stdio.h>
int main() {
int myNumber;
printf("Enter a number: ");
scanf("%d",&myNumber);
if(myNumber>20){
printf("The number is greater than 20");
}else if(myNumber==20){
printf("The number is equal to 20");
}else{
printf("The number is less than 20");
}
return 0;
}
Output:



switch-case statements
#include <stdio.h>
int main() {
int myNumber = 30;
switch(myNumber){
case 20:
printf("myNumber is 20");
break;
case 25:
printf("myNumber is 25");
break;
case 30:
printf("myNumber is 30");
break;
default:
printf("myNumber is unknown");
}
return 0;
}
Output:
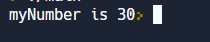
Loops
while loop
#include <stdio.h>
int main() {
int myNumber = 1;
while(myNumber<=10){
printf("%d \n",myNumber);
myNumber++;
}
}
Output:
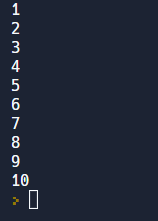
do-while loop
The do-while loop gets executed at least once (even if the while condition is not true).
#include <stdio.h>
int main() {
int myNumber = 1;
//while loop
do{
printf("%d \n",myNumber);
myNumber++;
} while(myNumber<=10);
}
Output:
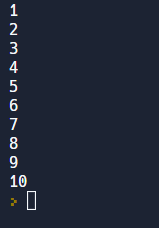
for loop
#include <stdio.h>
int main() {
//for(initialize, condition, increment)
for(int i=0;i<=10;i++){
printf("%d \n",i);
}
}
Output:
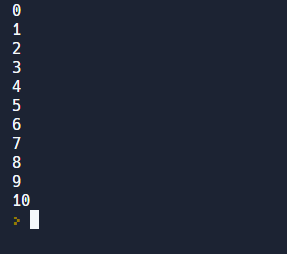