Do you want to draw some cool pictures with a few lines of Python code? SketchPy is here to help. In this article, let’s look at what sketchpy is and how you can use it to draw pictures using Python on your computer.
Let’s dive right in.
Installing SketchPy
Sketchpy is a Python module for animating drawings of images. The sketchpy module is created on top of the turtle module in Python.
To install sketchpy on your computer, you can go to your command prompt (command line) and run the following command.
pip install sketchpy
Once you enter this command, sketchpy will get automatically installed on your system.
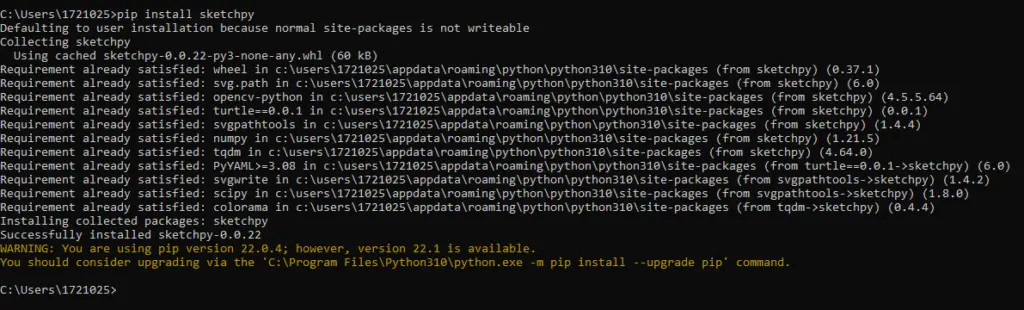
Now you are all set to use this module and draw some awesome stuff on your computer.
Open your code editor and write the example Python code snippets given below. Run your code and see the magic by yourself.
Drawing Robert Downey Jr. Using Python
from sketchpy import library
myObject = library.rdj()
myObject.draw()
Output:
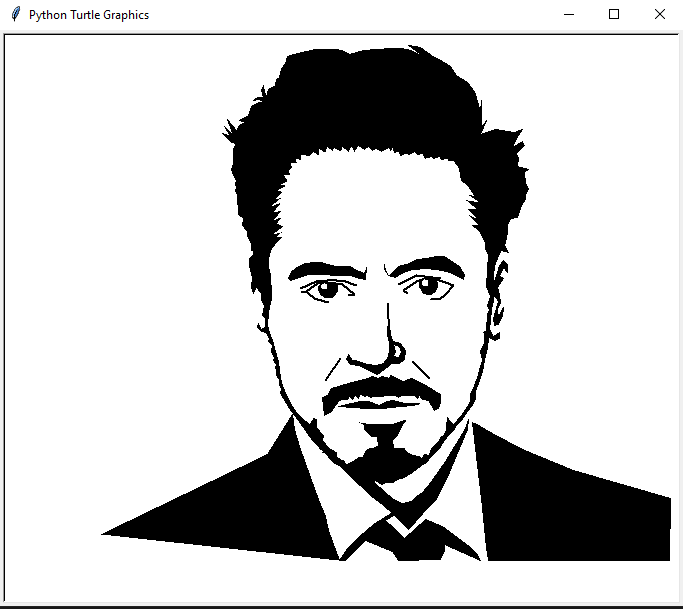
Drawing Tom Holland Using Python
from sketchpy import library
myObject = library.tom_holland()
myObject.draw()
Output:
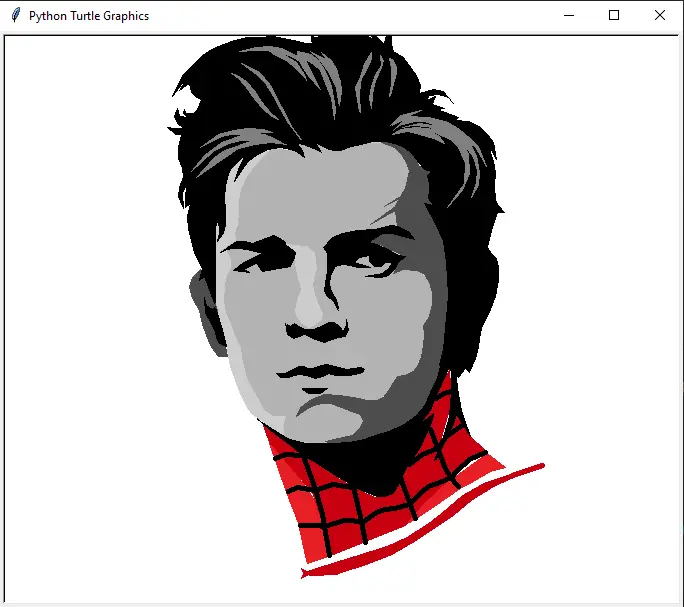
Drawing BTS Using Python
from sketchpy import library
myObject = library.bts()
myObject.draw()
Output:
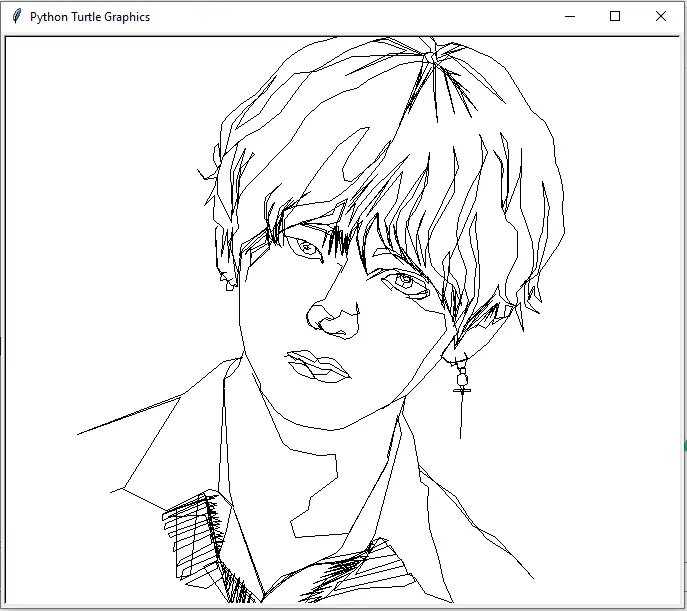
Drawing Iron Man ASCII Animation Using Python
from sketchpy import library
myObject = library.ironman_ascii()
myObject.draw()
Output:
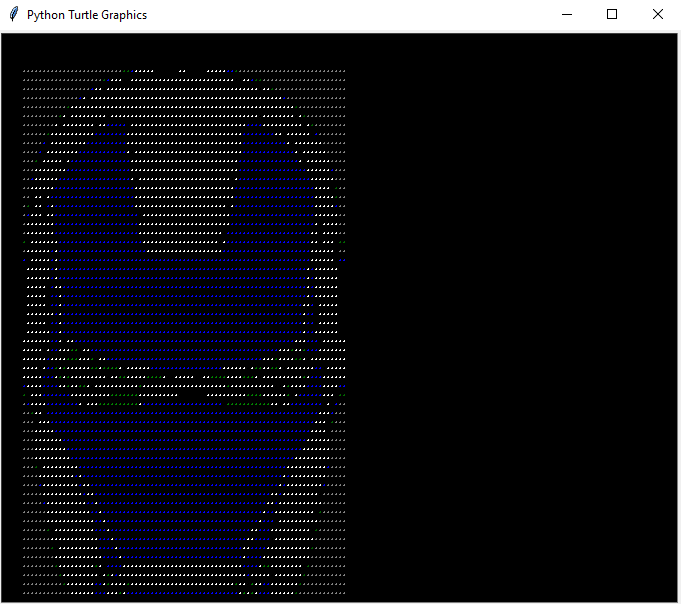
Drawing Gojo Using Python
from sketchpy import library
myObject = library.gojo()
myObject.draw()
Output:
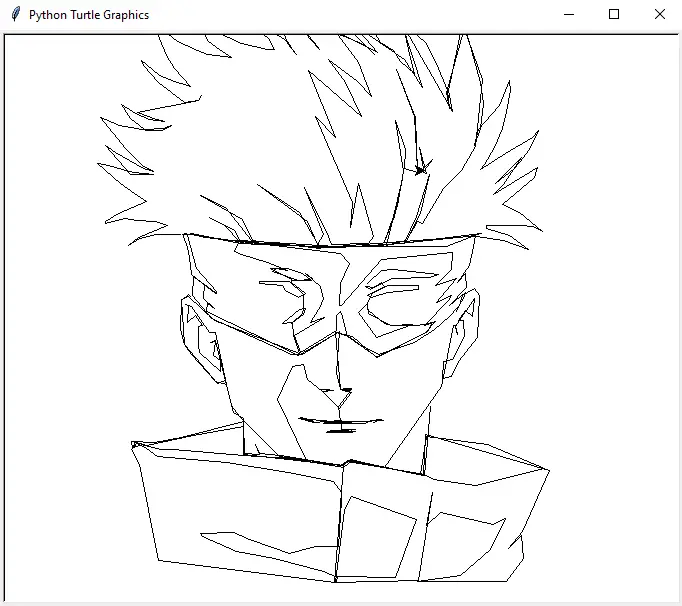
Drawing Indian Flag Using Python
from sketchpy import library
myObject = library.flag()
myObject.draw()
Output:
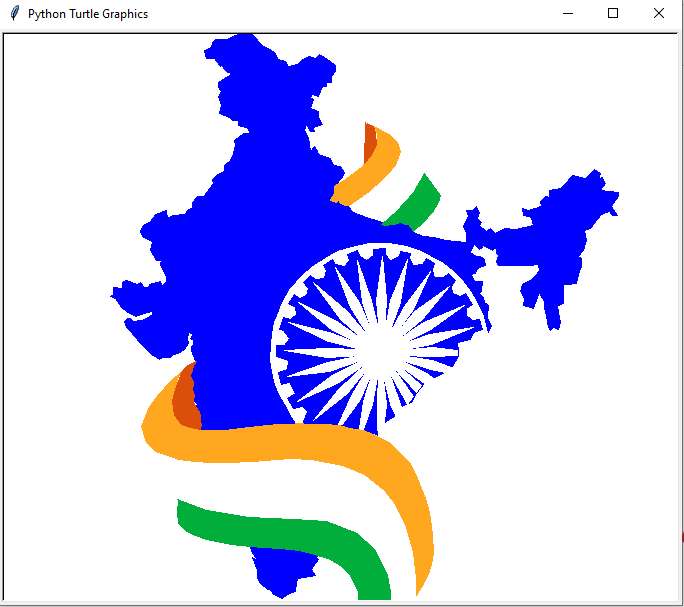
Drawing APJ Abdul Kalam Using Python
from sketchpy import library
myObject = library.apj()
myObject.draw()
Output:
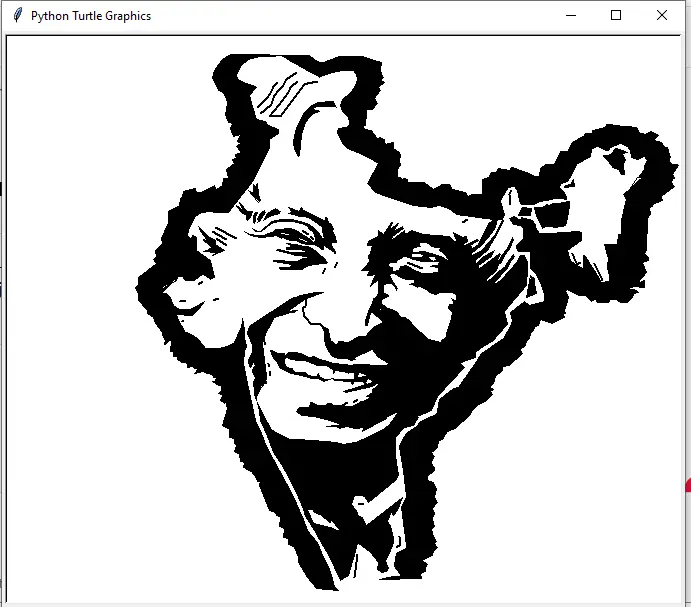
Drawing Vijay Using Python
from sketchpy import library
myObject = library.vijay()
myObject.draw()
Output:
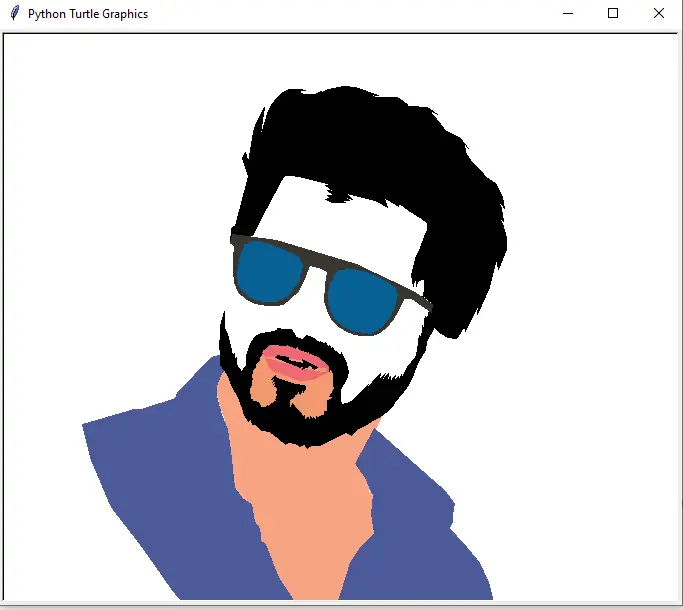
For more information about the sketchpy project, check out this link.
Hello Ashwin,
This is quite impressive! So can we also use our pictures or is it only for those pictures which are available in the library?
You can also draw pictures using your own images in the following way:
from sketchpy import canvas
obj = canvas.sketch_from_image(‘IMAGE PATH’)
obj = draw(threshold = 127)
Hai, I am working with this same method but not drawing my image
For those raw image is not working double the ‘/’ in the path i.e ‘//’ and unicode error will be resolved other errors pls specify
I’can pip install sketchpy
Install Python from Microsoft Store. Then use cmd to pip install
How can we write the name of the person on the canvas after completing the drawing.
It say’s Draw is not defined
obj.draw(threshold=127)
Traceback (most recent call last):
File “C:\Users\KK\AppData\Local\Packages\PythonSoftwareFoundation.Python.3.11_qbz5n2kfra8p0\LocalCache\local-packages\Python311\site-packages\sketchpy\library.py”, line 232, in log
with open(path, ‘r’) as f:
^^^^^^^^^^^^^^^
FileNotFoundError: [Errno 2] No such file or directory: ‘C:\\Users\\KK\\AppData\\Local\\Programs\\Python\\data.txt’
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File “f:\Python\sketch.py”, line 1, in
from sketchpy import library as lib
File “C:\Users\KK\AppData\Local\Packages\PythonSoftwareFoundation.Python.3.11_qbz5n2kfra8p0\LocalCache\local-packages\Python311\site-packages\sketchpy\library.py”, line 243, in
old_data = log(1, path)
^^^^^^^^^^^^
File “C:\Users\KK\AppData\Local\Packages\PythonSoftwareFoundation.Python.3.11_qbz5n2kfra8p0\LocalCache\local-packages\Python311\site-packages\sketchpy\library.py”, line 240, in log
with open(path, ‘w’) as f:
^^^^^^^^^^^^^^^
FileNotFoundError: [Errno 2] No such file or directory: ‘C:\\Users\\KK\\AppData\\Local\\Programs\\Python\\data.txt’
I get this error whether using libraries or canvas
Vedant. I also got the same error. Please do u know how to reslove it?
Why my python don’t read sketchpy & canvas.
Have you installed sketchpy using pip?
i am also faced this issue
obj = canvas.sketch_from_image(“wings.png”)
^^^^^^^^^^^^^^^^^^^^^^^^
AttributeError: module ‘sketchpy.canvas’ has no attribute ‘sketch_from_image’. Did you mean: ‘sketch_from_svg’?
PS C:\Users\Owner\Desktop\PythonFiles>
CODE used
from sketchpy import canvas
obj = canvas.sketch_from_image(‘IMAGE PATH’)
obj = draw(threshold = 127)
I get the error on top. same when I list Path to image
C:\Users\Owner\Desktop\PythonFiles\Wings.png
Hello ashwin ,
I am getting error while drawing my own picture in the last line of code.
It says ” name draw is not defined”
please help me out…
Exception has occurred: FileNotFoundError
[Errno 2] No such file or directory: ‘C:\\Users\\user\\AppData\\Local\\Programs\\Python\\data.txt’
FileNotFoundError: [Errno 2] No such file or directory: ‘C:\\Users\\user\\AppData\\Local\\Programs\\Python\\data.txt’
During handling of the above exception, another exception occurred:
File “C:\Users\user\Downloads\sketchpy-main\sketchpy-main\build\lib\sketchpy\__init__.py”, line 1, in
from sketchpy import library
FileNotFoundError: [Errno 2] No such file or directory: ‘C:\\Users\\user\\AppData\\Local\\Programs\\Python\\data.txt
I’m also getting same error. Did you resolve it?