Image recognition is now being used in plenty of real-world applications. In the food industry, artificial intelligence (AI) and image recognition can be used to detect food to automatically remove damaged food items.
In this tutorial, let’s see how we can easily implement food detection using LogMeal API, an online platform for AI and deep learning solutions for food recognition. Don’t worry, I’ll share the step-by-step instructions and the complete code.
Let’s dive right in.
Step 1: Create a LogMeal API Account
Open the LogMeal website and sign up for a new account.
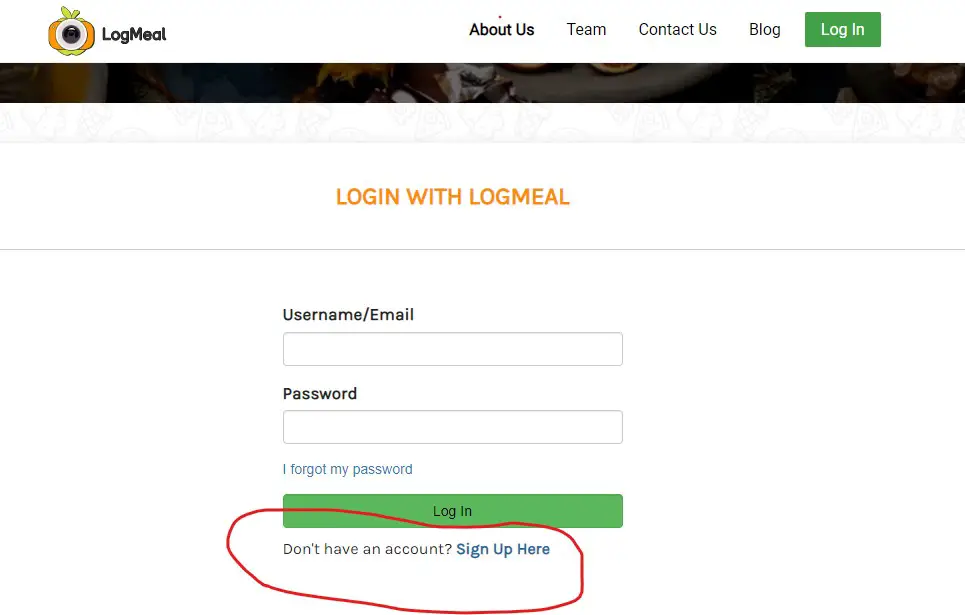
Enter your username, email id, and create a password. Choose the “Free” plan. You don’t need to add any payment method details. Make sure you verify your email address. You will get a mail to your email id, where you can confirm your email.
Once your account is active, you can see your profile like this:
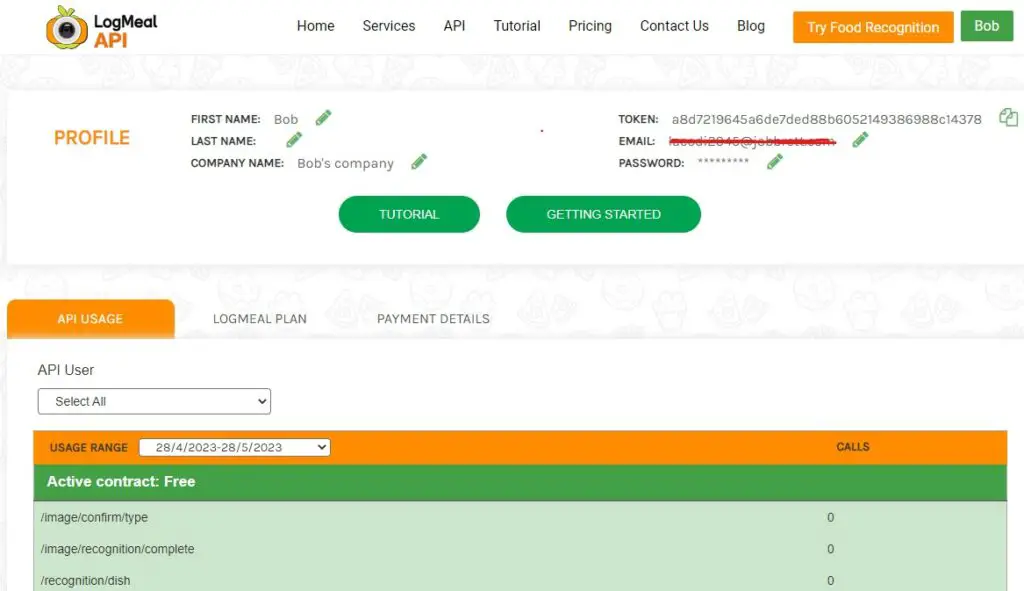
Step 2: Find the API Key
You can find your API key in your LogMeal account by clicking on your username at the top-right corner and then clicking on “Users“.
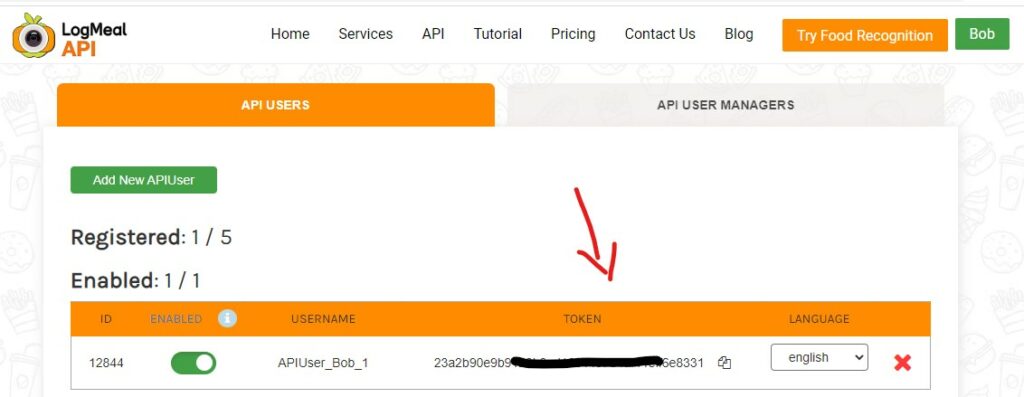
Copy the API token. We will be using this API key in our Python code.
Step 3: Write Python Code for Food Detection
We can use Python to send the API request to the LogMeal API, receive the response, and based on the JSON response we receive, do the prediction.
In this example, we will be doing the food type prediction. The categories are food, drinks, non-food, and ingredients. When we upload the image of a food item, the LogMeal API will predict the probability of the image being in all these categories. We will print the category that gets the most probability as the output.
Here is the Python code to implement this:
import requests
# Parameters
img = 'IMAGE_PATH'
api_user_token = 'YOUR_API_KEY'
headers = {'Authorization': 'Bearer ' + api_user_token}
# Food Type Detection
api_url = 'https://api.logmeal.es/v2'
endpoint = '/image/recognition/type'
response = requests.post(api_url + endpoint,
files={'image': open(img, 'rb')},
headers=headers)
resp = response.json()
#print(resp)
# Loop through food_types and print the name with highest probability
max_prob = 0
max_name = ''
for food in resp['food_types']:
if food['probs'] > max_prob:
max_prob = food['probs']
max_name = food['name']
print(max_name)
Note: Don’t forget to replace “IMAGE_PATH” with the path of the image that you want to test and “YOUR_API_KEY” with your actual LogMeal API key.
Also, make sure you add clean images of food items without any other objects in the frame (in .jpg or .jpeg format). The cleaner the image is, the better the prediction will be.
For example, when I tested the code with the following image, the prediction was “food”.
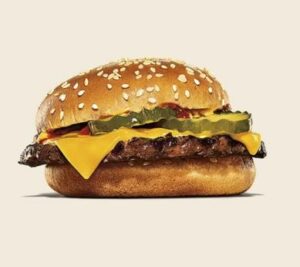
And for the following image, the prediction was “drinks”.
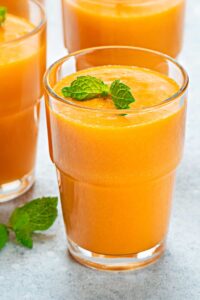
Now you can run the code and see the predictions by yourself. Test the code with different images of food items and see how accurate the predictions are.
Final Thoughts
This was a simple way to do food detection from images using Python. Apart from food type detection, you can use this API for food group detection, dishes recognition, ingredient information, nutrition information, etc. If you want to explore more, check out their official tutorial.
We haven’t discussed what is happening behind the scenes and how this API handles image detection. That is out of the scope of this tutorial. If you want to know how to classify images using Python and deep learning in detail, check out my tutorial on that topic.
I hope this article was helpful. Happy coding!